Updated external content (Jenkins build 1439)
parent
f05de57ecb
commit
ebc9d573b3
File diff suppressed because one or more lines are too long
|
@ -16,6 +16,7 @@ install: manual
|
|||
# JavaScript Scripting
|
||||
|
||||
This add-on provides support for JavaScript (ECMAScript 2022+) that can be used as a scripting language within automation rules.
|
||||
It is based on [GraalJS](https://www.graalvm.org/javascript/) from the [GraalVM project](https://www.graalvm.org/).
|
||||
|
||||
Also included is [openhab-js](https://github.com/openhab/openhab-js/), a fairly high-level ES6 library to support automation in openHAB. It provides convenient access
|
||||
to common openHAB functionality within rules including items, things, actions, logging and more.
|
||||
|
@ -26,8 +27,9 @@ to common openHAB functionality within rules including items, things, actions, l
|
|||
- [Adding Actions](#adding-actions)
|
||||
- [UI Event Object](#ui-event-object)
|
||||
- [Scripting Basics](#scripting-basics)
|
||||
- [Require](#require)
|
||||
- [Console](#console)
|
||||
- [`let` and `const`](#let-and-const)
|
||||
- [`require`](#require)
|
||||
- [`console`](#console)
|
||||
- [Timers](#timers)
|
||||
- [Paths](#paths)
|
||||
- [Deinitialization Hook](#deinitialization-hook)
|
||||
|
@ -37,8 +39,9 @@ to common openHAB functionality within rules including items, things, actions, l
|
|||
- [Things](#things)
|
||||
- [Actions](#actions)
|
||||
- [Cache](#cache)
|
||||
- [Log](#log)
|
||||
- [Time](#time)
|
||||
- [Quantity](#quantity)
|
||||
- [Log](#log)
|
||||
- [Utils](#utils)
|
||||
- [File Based Rules](#file-based-rules)
|
||||
- [JSRule](#jsrule)
|
||||
|
@ -110,6 +113,8 @@ See [openhab-js](https://openhab.github.io/openhab-js) for a complete list of fu
|
|||
### UI Event Object
|
||||
|
||||
**NOTE**: Note that `event` object is different in UI based rules and file based rules! This section is only valid for UI based rules. If you use file based rules, refer to [file based rules event object documentation](#event-object).
|
||||
Note that `event` object is only available when the UI based rule was triggered by an event and is not manually run!
|
||||
Trying to access `event` on manual run does not work (and will lead to an error), use `this.event` instead (will be `undefined` in case of manual run).
|
||||
|
||||
When you use "Item event" as trigger (i.e. "[item] received a command", "[item] was updated", "[item] changed"), there is additional context available for the action in a variable called `event`.
|
||||
|
||||
|
@ -145,13 +150,33 @@ console.log(event.itemState.toString() == "test") // OK
|
|||
|
||||
The openHAB JavaScript Scripting runtime attempts to provide a familiar environment to JavaScript developers.
|
||||
|
||||
### Require
|
||||
### `let` and `const`
|
||||
|
||||
Due to the way how openHAB runs UI based scripts, `let`, `const` and `class` are not supported at top-level.
|
||||
Use `var` instead or wrap your script inside a self-invoking function:
|
||||
|
||||
```javascript
|
||||
// Wrap script inside a self-invoking function:
|
||||
(function (data) {
|
||||
const C = 'Hello world';
|
||||
console.log(C);
|
||||
})(this.event);
|
||||
|
||||
// Defining a class using var:
|
||||
var Tree = class {
|
||||
constructor (height) {
|
||||
this.height = height;
|
||||
}
|
||||
}
|
||||
```
|
||||
|
||||
### `require`
|
||||
|
||||
Scripts may include standard NPM based libraries by using CommonJS `require`.
|
||||
The library search will look in the path `automation/js/node_modules` in the user configuration directory.
|
||||
See [libraries](#libraries) for more information.
|
||||
|
||||
### Console
|
||||
### `console`
|
||||
|
||||
The JS Scripting binding supports the standard `console` object for logging.
|
||||
Script logging is enabled by default at the `INFO` level (messages from `console.debug` and `console.trace` won't be displayed), but can be configured using the [openHAB console](https://www.openhab.org/docs/administration/console.html):
|
||||
|
@ -194,7 +219,7 @@ JS Scripting provides access to the global `setTimeout`, `setInterval`, `clearTi
|
|||
|
||||
When a script is unloaded, all created timeouts and intervals are automatically cancelled.
|
||||
|
||||
#### SetTimeout
|
||||
#### 'setTimeout'
|
||||
|
||||
The global [`setTimeout()`](https://developer.mozilla.org/en-US/docs/Web/API/setTimeout) method sets a timer which executes a function once the timer expires.
|
||||
`setTimeout()` returns a `timeoutId` (a positive integer value) which identifies the timer created.
|
||||
|
@ -207,7 +232,7 @@ var timeoutId = setTimeout(callbackFunction, delay);
|
|||
|
||||
The global [`clearTimeout(timeoutId)`](https://developer.mozilla.org/en-US/docs/Web/API/clearTimeout) method cancels a timeout previously established by calling `setTimeout()`.
|
||||
|
||||
#### SetInterval
|
||||
#### `setInterval`
|
||||
|
||||
The global [`setInterval()`](https://developer.mozilla.org/en-US/docs/Web/API/setInterval) method repeatedly calls a function, with a fixed time delay between each call.
|
||||
`setInterval()` returns an `intervalId` (a positive integer value) which identifies the interval created.
|
||||
|
@ -355,9 +380,9 @@ Calling `getItem(...)` or `...` returns an `Item` object with the following prop
|
|||
- .getMetadata(namespace) ⇒ `object|null`
|
||||
- .replaceMetadata(namespace, value, configuration) ⇒ `object`
|
||||
- .removeMetadata(namespace) ⇒ `object|null`
|
||||
- .sendCommand(value): `value` can be a string or a [`time.ZonedDateTime`](#time)
|
||||
- .sendCommandIfDifferent(value) ⇒ `boolean`: `value` can be a string or a [`time.ZonedDateTime`](#time)
|
||||
- .postUpdate(value): `value` can be a string or a [`time.ZonedDateTime`](#time)
|
||||
- .sendCommand(value): `value` can be a string, a [`time.ZonedDateTime`](#time) or a [`Quantity`](#quantity)
|
||||
- .sendCommandIfDifferent(value) ⇒ `boolean`: `value` can be a string, a [`time.ZonedDateTime`](#time) or a [`Quantity`](#quantity)
|
||||
- .postUpdate(value): `value` can be a string, a [`time.ZonedDateTime`](#time) or a [`Quantity`](#quantity)
|
||||
- .addGroups(...groupNamesOrItems)
|
||||
- .removeGroups(...groupNamesOrItems)
|
||||
- .addTags(...tagNames)
|
||||
|
@ -770,9 +795,9 @@ The following rules are used during the conversion:
|
|||
| `java.time.ZonedDateTime` | converted to the `time.ZonedDateTime` equivalent | |
|
||||
| JavaScript native `Date` | converted to the equivalent `time.ZonedDateTime` using `SYSTEM` as the timezone | |
|
||||
| `number`, `bingint`, `java.lang.Number`, `DecimalType` | rounded to the nearest integer and added to `now` as milliseconds | `time.toZDT(1000);` |
|
||||
| [`Quantity`](#quantity) or `QuantityType` | if the units are `Time`, that time is added to `now` | `time.toZDT(item.getItem('MyTimeItem').rawState);`, `time.toZDT(Quantity('10 min'));` |
|
||||
| [`Quantity`](#quantity) or `QuantityType` | if the unit is time-compatible, added to `now` | `time.toZDT(item.getItem('MyTimeItem').rawState);`, `time.toZDT(Quantity('10 min'));` |
|
||||
| `items.Item` or `org.openhab.core.types.Item` | if the state is supported (see the `Type` rules in this table, e.g. `DecimalType`), the state is converted | `time.toZDT(items.getItem('MyItem'));` |
|
||||
| `String`, `java.lang.String`, `StringType` | parsed based on the following rules | |
|
||||
| `String`, `java.lang.String`, `StringType` | parsed based on the following rules; if no timezone is specified, `SYSTEM` timezone is used | |
|
||||
| [ISO8601 Date/Time](https://en.wikipedia.org/wiki/ISO_8601) String | parsed, depending on the provided data: if no date is passed, today's date; if no time is passed, midnight time | `time.toZDT('00:00');`, `time.toZDT('2022-12-24');`, `time.toZDT('2022-12-24T18:30');` |
|
||||
| RFC String (output from a Java `ZonedDateTime.toString()`) | parsed | `time.toZDT('2019-10-12T07:20:50.52Z');` |
|
||||
| `"kk:mm[:ss][ ]a"` (12 hour time) | today's date with the time indicated, the space between the time and am/pm and seconds are optional | `time.toZDT('1:23:45 PM');` |
|
||||
|
@ -1092,7 +1117,7 @@ Operations and conditions can also optionally take functions:
|
|||
|
||||
```javascript
|
||||
rules.when().item("F1_light").changed().then(event => {
|
||||
console.log(event);
|
||||
console.log(event);
|
||||
}).build("Test Rule", "My Test Rule");
|
||||
```
|
||||
|
||||
|
@ -1169,7 +1194,7 @@ Additionally, all the above triggers have the following functions:
|
|||
```javascript
|
||||
// Basic rule, when the BedroomLight1 is changed, run a custom function
|
||||
rules.when().item('BedroomLight1').changed().then(e => {
|
||||
console.log("BedroomLight1 state", e.newState)
|
||||
console.log("BedroomLight1 state", e.newState)
|
||||
}).build();
|
||||
|
||||
// Turn on the kitchen light at SUNSET
|
||||
|
|
|
@ -279,6 +279,16 @@ Example :
|
|||
logInfo("AstroActions", "{} will be positioned at elevation {} - azimuth {}",sunEvent, elevation.toString,azimuth.toString)
|
||||
```
|
||||
|
||||
### getTotalRadiation(timeStamp)
|
||||
|
||||
Retrieves the total radiation (QuantityType<Intensity>) of the sun at the requested instant.
|
||||
Thing method only applies to Sun thing type.
|
||||
|
||||
```java
|
||||
val totalRadiation = sunActions.getTotalRadiation(ZonedDateTime.now)
|
||||
logInfo("AstroActions", "Currently, the total sun radiation is {}", totalRadiation.toString)
|
||||
```
|
||||
|
||||
## Tips
|
||||
|
||||
Do not worry if for example the "astro dawn" is undefined at your location.
|
||||
|
|
|
@ -126,10 +126,10 @@ Bridge modbus:tcp:bridge "Stiebel Modbus TCP"[ host="hostname|ip", port=502, id=
|
|||
### Item Configuration
|
||||
|
||||
```java
|
||||
Number:Temperature stiebel_eltron_temperature_ffk "Temperature FFK [%.1f °C]" <temperature> { channel="modbus:heatpump:stiebelEltron:systemInformation#fek-temperature" }
|
||||
Number:Temperature stiebel_eltron_setpoint_ffk "Set point FFK [%.1f °C]" <temperature> { channel="modbus:heatpump:stiebelEltron:systemInformation#fek-temperature-setpoint" }
|
||||
Number:Dimensionless stiebel_eltron_humidity_ffk "Humidity FFK [%.1f %%]" <humidity> { channel="modbus:heatpump:stiebelEltron:systemInformation#fek-humidity" }
|
||||
Number:Temperature stiebel_eltron_dewpoint_ffk "Dew point FFK [%.1f °C]" <temperature> { channel="modbus:heatpump:stiebelEltron:systemInformation#fek-dewpoint" }
|
||||
Number:Temperature stiebel_eltron_temperature_fek "Temperature FEK [%.1f °C]" <temperature> { channel="modbus:heatpump:stiebelEltron:systemInformation#fek-temperature" }
|
||||
Number:Temperature stiebel_eltron_setpoint_fek "Set point FEK [%.1f °C]" <temperature> { channel="modbus:heatpump:stiebelEltron:systemInformation#fek-temperature-setpoint" }
|
||||
Number:Dimensionless stiebel_eltron_humidity_fek "Humidity FEK [%.1f %%]" <humidity> { channel="modbus:heatpump:stiebelEltron:systemInformation#fek-humidity" }
|
||||
Number:Temperature stiebel_eltron_dewpoint_fek "Dew point FEK [%.1f °C]" <temperature> { channel="modbus:heatpump:stiebelEltron:systemInformation#fek-dewpoint" }
|
||||
|
||||
Number:Temperature stiebel_eltron_outdoor_temp "Outdoor temperature [%.1f °C]" { channel="modbus:heatpump:stiebelEltron:systemInformation#outdoor-temperature" }
|
||||
Number:Temperature stiebel_eltron_temp_hk1 "Temperature HK1 [%.1f °C]" { channel="modbus:heatpump:stiebelEltron:systemInformation#hk1-temperature" }
|
||||
|
@ -184,10 +184,10 @@ Text label="Heat pumpt" icon="temperature" {
|
|||
Default item=stiebel_eltron_ruecklauf_temp icon="temperature"
|
||||
Default item=stiebel_eltron_temp_water icon="temperature"
|
||||
Default item=stiebel_eltron_setpoint_water icon="temperature"
|
||||
Default item=stiebel_eltron_temperature_ffk icon="temperature"
|
||||
Default item=stiebel_eltron_setpoint_ffk icon="temperature"
|
||||
Default item=stiebel_eltron_humidity_ffk icon="humidity"
|
||||
Default item=stiebel_eltron_dewpoint_ffk icon="temperature"
|
||||
Default item=stiebel_eltron_temperature_fek icon="temperature"
|
||||
Default item=stiebel_eltron_setpoint_fek icon="temperature"
|
||||
Default item=stiebel_eltron_humidity_fek icon="humidity"
|
||||
Default item=stiebel_eltron_dewpoint_fek icon="temperature"
|
||||
Default item=stiebel_eltron_source_temp icon="temperature"
|
||||
}
|
||||
Frame label="Paramters" {
|
||||
|
|
|
@ -37,7 +37,6 @@ After the ojcloud bridge is successfully initialized all thermostats will be dis
|
|||
| password | password from the OJElectronics App (required) |
|
||||
| apiKey | API key. You get the key from your local distributor. |
|
||||
| apiUrl | URL of the API endpoint. Optional, the default value should always work. |
|
||||
| refreshDelayInSeconds | Refresh interval in seconds. Optional, the default value is 30 seconds. |
|
||||
| customerId | Customer ID. Optional, the default value should always work. |
|
||||
| softwareVersion | Software version. Optional, the default value should always work. |
|
||||
|
||||
|
|
|
@ -0,0 +1,133 @@
|
|||
---
|
||||
id: speedtest
|
||||
label: Speedtest
|
||||
title: Speedtest - Bindings
|
||||
type: binding
|
||||
description: "The Speedtest Binding can be used to perform a network speed test for your openHAB instance."
|
||||
since: 3x
|
||||
logo: images/addons/speedtest.png
|
||||
install: manual
|
||||
---
|
||||
|
||||
<!-- Attention authors: Do not edit directly. Please add your changes to the appropriate source repository -->
|
||||
|
||||
{% include base.html %}
|
||||
|
||||
# Speedtest Binding
|
||||
|
||||
The Speedtest Binding can be used to perform a network speed test for your openHAB instance.
|
||||
It is based on the command line interface (CLI) version of Ookla's Speedtest (https://www.speedtest.net/apps/cli).
|
||||
|
||||
The Ookla CLI Speedtest application MUST be installed on your openHAB instance when using the Speedtest Binding.
|
||||
|
||||
## Why Ookla's Speedtest?
|
||||
|
||||
Fully supported and maintained by Ookla with the latest Speedtest technology for best results:
|
||||
|
||||
- Consistent measurement, even on high bandwidth
|
||||
- Consistent measurement, very much independent from the performance of the host system
|
||||
|
||||
## What functionality does Ookla's Speedtest offer?
|
||||
|
||||
The Speedtest Binding is using the following functionality, provided by Ookla's Speedtest:
|
||||
|
||||
- Output of an accurate timestamp per measurement
|
||||
- Output of Ping time and Jitter
|
||||
- Output of Bandwidth, transferred Bytes and elapsed time for Down-/Upload
|
||||
- Output of the used interface with Internal/External IP, MAC Address and the Internet Service Provider (ISP)
|
||||
- Output of a result ID and a result URL
|
||||
- Output of the used Server and location the Speedtest was run against
|
||||
- Possibility to pre-select a server used for testing
|
||||
|
||||
## What interfaces does the Speedtest Binding offer?
|
||||
|
||||
The Speedtest Binding provides the Ookla's Speedtest functionality via the following openHAB interface:
|
||||
|
||||
- Execute Speedtest time based or triggered
|
||||
- Provide results via openHAB Channels
|
||||
- List available Ookla Speedtest servers that can be used for testing (optional)
|
||||
|
||||
## Ookla License and Privacy Terms
|
||||
|
||||
When using this binding, you automatically accept the license and privacy terms of Ookla's Speedtest.
|
||||
You can find the latest version of those terms at the following webpages:
|
||||
|
||||
- https://www.speedtest.net/about/eula
|
||||
- https://www.speedtest.net/about/terms
|
||||
- https://www.speedtest.net/about/privacy
|
||||
|
||||
## Supported Things
|
||||
|
||||
Speedtest thing.
|
||||
|
||||
## Binding Configuration
|
||||
|
||||
For this binding to work, you MUST install Ookla's Speedtest command line tool (`speedtest` or `speedtest.exe`).
|
||||
It will not work with other versions like `speedtest-cli` or other `speedtest` variants.
|
||||
|
||||
To install Ookla's version of Speedtest, head to https://www.speedtest.net/apps/cli and follow the instructions for your Operating System.
|
||||
|
||||
## Thing Configuration
|
||||
|
||||
| Parameter | Description | Default |
|
||||
|-------------------|------------------------------------------------------------------------------------------------------------------------------|---------|
|
||||
| `refreshInterval` | How often to test network speed, in minutes | `60` |
|
||||
| `execPath` | The path of the Ookla Speedtest executable.<br/>Linux machines may leave this blank and it defaults to `/usr/bin/speedtest`. | |
|
||||
| `serverID` | Optional: A specific server that shall be used for testing. You can pick the server ID from the "Thing Properties".<br/>If this is left blank the best option will be selected by Ookla. | |
|
||||
|
||||
The `refreshInterval` parameter can also be set to `0` which means "Do not test automatically".
|
||||
This can be used if you want to use the "Trigger Test" channel in order to test via rules, or an item instead.
|
||||
|
||||
Ensure that the user that openHAB is running with, has the permissions to access and execute the executable.
|
||||
|
||||
## Properties
|
||||
|
||||
| Property | Description |
|
||||
|---------------------|------------------------------------------------------------------------------------------------------------|
|
||||
| Server List 1...10 | A List of Ookla Speedtest servers that can be used in order to specify a specific server for the Speedtest.<br/>Configure the Server ID via the `serverID` Thing Configuration Parameter. |
|
||||
|
||||
## Channels
|
||||
|
||||
| Channel | Type | Description |
|
||||
|-----------------------|---------------------------|-------------------------------------------------------------------|
|
||||
| `server` | `String` | The remote server that the Speedtest was run against |
|
||||
| `pingJitter` | `Number:Time` | Ping Jitter - the variation in the response time |
|
||||
| `pingLatency` | `Number:Time` | Ping Latency - the reaction time of your internet connection |
|
||||
| `downloadBandwidth` | `Number:DataTransferRate` | Download bandwidth, e.g. in Mbit/s |
|
||||
| `downloadBytes` | `Number:DataAmount` | Amount of data that was used for the last download bandwidth test |
|
||||
| `downloadElapsed` | `Number:Time` | Time spent for the last download bandwidth test |
|
||||
| `uploadBandwidth` | `Number:DataTransferRate` | Upload bandwidth, e.g. in Mbit/s |
|
||||
| `uploadBytes` | `Number:DataAmount` | Amount of data that was used for the last upload bandwidth test |
|
||||
| `uploadElapsed` | `Number:Time` | Time spent for the last upload bandwidth test |
|
||||
| `isp` | `String` | Your Internet Service Provider (ISP) as calculated by Ookla |
|
||||
| `interfaceInternalIp` | `String` | IP address of the internal interface that was used for the test |
|
||||
| `interfaceExternalIp` | `String` | IP address of the external interface that was used for the test |
|
||||
| `resultUrl` | `String` | The URL to the Speedtest results in HTML on the Ookla webserver |
|
||||
| `triggerTest` | `Switch` | Trigger in order to run Speedtest manually |
|
||||
|
||||
## Full Example
|
||||
|
||||
### Thing File
|
||||
|
||||
```java
|
||||
Thing speedtest:speedtest:myspeedtest "Ookla Speedtest" [ execPath="/usr/bin/speedtest", refreshInterval=60 ]
|
||||
```
|
||||
|
||||
### Item File
|
||||
|
||||
```java
|
||||
String Speedtest_Server "Server" { channel="speedtest:speedtest:myspeedtest:server" }
|
||||
Number:Time Speedtest_Ping_Jitter "Ping Jitter" { channel="speedtest:speedtest:myspeedtest:pingJitter" }
|
||||
Number:Time Speedtest_Ping_Latency "Ping Latency" { channel="speedtest:speedtest:myspeedtest:pingLatency" }
|
||||
Number:DataTransferRate Speedtest_Download_Bandwith "Download Bandwith" { channel="speedtest:speedtest:myspeedtest:downloadBandwidth" }
|
||||
Number:DataAmount Speedtest_Download_Bytes "Download Bytes" { channel="speedtest:speedtest:myspeedtest:downloadBytes" }
|
||||
Number:Time Speedtest_Download_Elapsed "Download Elapsed" { channel="speedtest:speedtest:myspeedtest:downloadElapsed" }
|
||||
Number:DataTransferRate Speedtest_Upload_Bandwith "Upload Bandwith" { channel="speedtest:speedtest:myspeedtest:uploadBandwidth" }
|
||||
Number:DataAmount Speedtest_Upload_Bytes "Upload Bytes" { channel="speedtest:speedtest:myspeedtest:uploadBytes" }
|
||||
Number:Time Speedtest_Upload_Elapsed "Upload Elapsed" { channel="speedtest:speedtest:myspeedtest:uploadElapsed" }
|
||||
String Speedtest_ISP "ISP" { channel="speedtest:speedtest:myspeedtest:isp" }
|
||||
String Speedtest_Interface_InternalIP "Internal IP Address" { channel="speedtest:speedtest:myspeedtest:interfaceInternalIp" }
|
||||
String Speedtest_Interface_ExternalIP "External IP Address" { channel="speedtest:speedtest:myspeedtest:interfaceExternalIp" }
|
||||
String Speedtest_ResultURL "Result URL" { channel="speedtest:speedtest:myspeedtest:resultUrl" }
|
||||
Switch Speedtest_TriggerTest "Trigger Test" { channel="speedtest:speedtest:myspeedtest:triggerTest" }
|
||||
```
|
|
@ -0,0 +1,964 @@
|
|||
---
|
||||
layout: documentation
|
||||
title: ZWA037 - ZWave
|
||||
---
|
||||
|
||||
{% include base.html %}
|
||||
|
||||
# ZWA037 Aeotec Illumino Dimmer Switch
|
||||
This describes the Z-Wave device *ZWA037*, manufactured by *Aeotec Limited* with the thing type UID of ```aeotec_zwa037_00_000```.
|
||||
|
||||
The device is in the category of *Wall Switch*, defining Any device attached to the wall that controls a binary status of something, for ex. a light switch.
|
||||
|
||||
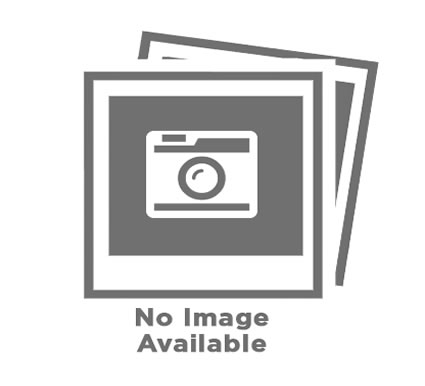
|
||||
|
||||
|
||||
The ZWA037 supports routing. This allows the device to communicate using other routing enabled devices as intermediate routers. This device is also able to participate in the routing of data between other devices in the mesh network.
|
||||
|
||||
## Overview
|
||||
|
||||
This product can be operated in any Z-Wave™ network with other Z-Wave Plus™ certified devices from other manufacturers. All non-battery operated nodes within the network will act as repeaters regardless of vendor to increase reliability of the network. Each module is designed to act as a repeater, which will re-transmit a radio frequency (RF) signal by routing the signal around obstacles and radio dead spots to ensure that the signal is received at its intended destination. ZWA037-A is a security enabled Z-Wave Plus™ device. A security Enabled Z-Wave Plus™
|
||||
|
||||
Controller must be used in order to fully utilize the product.
|
||||
|
||||
### Inclusion Information
|
||||
|
||||
Inclusion 1x tap
|
||||
|
||||
Out of network
|
||||
|
||||
Send NIF for network pairing/ inclusion (white LED quick flashes). If pairing is successful, the LED will turn to solid green for 2s, then deactivates.
|
||||
|
||||
In network
|
||||
|
||||
Trigger to send Central Scene 1x tap scene.
|
||||
|
||||
ON/OFF control of output load.
|
||||
|
||||
### Exclusion Information
|
||||
|
||||
Exclusion 3x tap
|
||||
|
||||
In network Send NIF for network unpairing/ exclusion (purple LED flashes).
|
||||
|
||||
Out of network
|
||||
|
||||
Trigger to send Central Scene 3x tap scene.
|
||||
|
||||
### General Usage Information
|
||||
|
||||
|
||||
|
||||
## Channels
|
||||
|
||||
The following table summarises the channels available for the ZWA037 -:
|
||||
|
||||
| Channel Name | Channel ID | Channel Type | Category | Item Type |
|
||||
|--------------|------------|--------------|----------|-----------|
|
||||
| Dimmer | switch_dimmer | switch_dimmer | DimmableLight | Dimmer |
|
||||
| Scene Number | scene_number | scene_number | | Number |
|
||||
| Scene Number | scene_number | scene_number | | Number |
|
||||
| Power Restore | config_decimal | config_decimal | | Number |
|
||||
| Auto Turn Off Timer | config_decimal | config_decimal | | Number |
|
||||
| Auto Turn On Timer | config_decimal | config_decimal | | Number |
|
||||
| Instant Status Report | config_decimal | config_decimal | | Number |
|
||||
| Association Control Settings | config_decimal | config_decimal | | Number |
|
||||
| Led Indicator Settings | config_decimal | config_decimal | | Number |
|
||||
| Led Indicator Color For Output | config_decimal | config_decimal | | Number |
|
||||
| Led Indicator Color For Scene | config_decimal | config_decimal | | Number |
|
||||
| Led Brightness | config_decimal | config_decimal | | Number |
|
||||
| Single Tap behavior | config_decimal | config_decimal | | Number |
|
||||
| Double Tap behavior | config_decimal | config_decimal | | Number |
|
||||
| External Switch Scene | config_decimal | config_decimal | | Number |
|
||||
| External Switch Type | config_decimal | config_decimal | | Number |
|
||||
| Output Control | config_decimal | config_decimal | | Number |
|
||||
| Button Function | config_decimal | config_decimal | | Number |
|
||||
| Report Behavior | config_decimal | config_decimal | | Number |
|
||||
| Ramp Rate for Tap and Hub control | config_decimal | config_decimal | | Number |
|
||||
| Ramp Rate for press and hold | config_decimal | config_decimal | | Number |
|
||||
| Minimum most dim setting | config_decimal | config_decimal | | Number |
|
||||
| Maximum most dim setting | config_decimal | config_decimal | | Number |
|
||||
| Custom brightness | config_decimal | config_decimal | | Number |
|
||||
|
||||
### Dimmer
|
||||
The brightness channel allows to control the brightness of a light.
|
||||
It is also possible to switch the light on and off.
|
||||
|
||||
The ```switch_dimmer``` channel is of type ```switch_dimmer``` and supports the ```Dimmer``` item and is in the ```DimmableLight``` category.
|
||||
|
||||
### Scene Number
|
||||
Triggers when a scene button is pressed.
|
||||
|
||||
The ```scene_number``` channel is of type ```scene_number``` and supports the ```Number``` item.
|
||||
|
||||
### Scene Number
|
||||
Triggers when a scene button is pressed.
|
||||
|
||||
The ```scene_number``` channel is of type ```scene_number``` and supports the ```Number``` item.
|
||||
This channel provides the scene, and the event as a decimal value in the form ```<scene>.<event>```. The scene number is set by the device, and the event is as follows -:
|
||||
|
||||
| Event ID | Event Description |
|
||||
|----------|--------------------|
|
||||
| 0 | Single key press |
|
||||
| 1 | Key released |
|
||||
| 2 | Key held down |
|
||||
| 3 | Double keypress |
|
||||
| 4 | Tripple keypress |
|
||||
| 5 | 4 x keypress |
|
||||
| 6 | 5 x keypress |
|
||||
|
||||
### Power Restore
|
||||
0 - return to OFF
|
||||
|
||||
1 - return to ON
|
||||
|
||||
2 - returns to last known state when repowered
|
||||
|
||||
Generic class for configuration parameter.
|
||||
|
||||
The ```config_decimal``` channel is of type ```config_decimal``` and supports the ```Number``` item.
|
||||
|
||||
### Auto Turn Off Timer
|
||||
0 - disabled
|
||||
|
||||
1 - Auto turn off after 1s once turned on
|
||||
|
||||
...
|
||||
|
||||
65535 - Auto turn off after 65535s once turned on
|
||||
|
||||
Generic class for configuration parameter.
|
||||
|
||||
The ```config_decimal``` channel is of type ```config_decimal``` and supports the ```Number``` item.
|
||||
|
||||
### Auto Turn On Timer
|
||||
0 - disabled
|
||||
|
||||
1 - Auto turn on after 1s once turned off
|
||||
|
||||
...
|
||||
|
||||
65535 - Auto turn on after 65535s once turned off
|
||||
|
||||
Generic class for configuration parameter.
|
||||
|
||||
The ```config_decimal``` channel is of type ```config_decimal``` and supports the ```Number``` item.
|
||||
|
||||
### Instant Status Report
|
||||
0 - Nothing
|
||||
|
||||
1 - Basic Report
|
||||
|
||||
2 - Binary Switch Report
|
||||
|
||||
Generic class for configuration parameter.
|
||||
|
||||
The ```config_decimal``` channel is of type ```config_decimal``` and supports the ```Number``` item.
|
||||
|
||||
### Association Control Settings
|
||||
1 - Basic Set
|
||||
|
||||
2- Binary Switch SET
|
||||
|
||||
Generic class for configuration parameter.
|
||||
|
||||
The ```config_decimal``` channel is of type ```config_decimal``` and supports the ```Number``` item.
|
||||
|
||||
### Led Indicator Settings
|
||||
0 - disable completely
|
||||
|
||||
1 - Momentary blink
|
||||
|
||||
2 - LED follows status of output
|
||||
|
||||
Generic class for configuration parameter.
|
||||
|
||||
The ```config_decimal``` channel is of type ```config_decimal``` and supports the ```Number``` item.
|
||||
|
||||
### Led Indicator Color For Output
|
||||
0 - Disabled
|
||||
|
||||
1 - Red
|
||||
|
||||
2 - Blue
|
||||
|
||||
3- Green
|
||||
|
||||
4- Pink
|
||||
|
||||
5- Cyan
|
||||
|
||||
6- Purple
|
||||
|
||||
7- Orange
|
||||
|
||||
8- Yellow
|
||||
|
||||
9- White
|
||||
|
||||
Generic class for configuration parameter.
|
||||
|
||||
The ```config_decimal``` channel is of type ```config_decimal``` and supports the ```Number``` item.
|
||||
|
||||
### Led Indicator Color For Scene
|
||||
0 - Disabled
|
||||
|
||||
1 - Red
|
||||
|
||||
2 - Blue
|
||||
|
||||
3- Green
|
||||
|
||||
4- Pink
|
||||
|
||||
5- Cyan
|
||||
|
||||
6- Purple
|
||||
|
||||
7- Orange
|
||||
|
||||
8- Yellow
|
||||
|
||||
9- White
|
||||
|
||||
Generic class for configuration parameter.
|
||||
|
||||
The ```config_decimal``` channel is of type ```config_decimal``` and supports the ```Number``` item.
|
||||
|
||||
### Led Brightness
|
||||
0 - Disable
|
||||
|
||||
1 - 1%
|
||||
|
||||
...
|
||||
|
||||
60 - 60%
|
||||
|
||||
...
|
||||
|
||||
100 - 100%
|
||||
|
||||
Generic class for configuration parameter.
|
||||
|
||||
The ```config_decimal``` channel is of type ```config_decimal``` and supports the ```Number``` item.
|
||||
|
||||
### Single Tap behavior
|
||||
0 - Last brightness level
|
||||
|
||||
1- Custom value from Param 133
|
||||
|
||||
2- Max brightness from Param 132
|
||||
|
||||
3- Full brightness at 100%
|
||||
|
||||
Generic class for configuration parameter.
|
||||
|
||||
The ```config_decimal``` channel is of type ```config_decimal``` and supports the ```Number``` item.
|
||||
|
||||
### Double Tap behavior
|
||||
0 - Full brightness at 99
|
||||
|
||||
...
|
||||
|
||||
1- Custom value from Param 133
|
||||
|
||||
2- Max brightness from Param 132
|
||||
|
||||
3- disabled
|
||||
|
||||
Generic class for configuration parameter.
|
||||
|
||||
The ```config_decimal``` channel is of type ```config_decimal``` and supports the ```Number``` item.
|
||||
|
||||
### External Switch Scene
|
||||
0 - Disable
|
||||
|
||||
1 - enable
|
||||
|
||||
Generic class for configuration parameter.
|
||||
|
||||
The ```config_decimal``` channel is of type ```config_decimal``` and supports the ```Number``` item.
|
||||
|
||||
### External Switch Type
|
||||
0 - toggle on or off only
|
||||
|
||||
1 - toggle switch/1x to toggle /2x use Param 132 or 133
|
||||
|
||||
/3x toggle dimmer loop ramp -> toggle once more to
|
||||
|
||||
stop dim
|
||||
|
||||
2 - momentary switch / 1x to toggle / 2x user Param 132
|
||||
|
||||
or 133/ Press and hold to dim up and down
|
||||
|
||||
3 - momentary switch / 1x quick toggle / 2x user Param
|
||||
|
||||
132 or 133 / Press and hold to dim up and down (but
|
||||
|
||||
after a double click to full brightness, then press and
|
||||
|
||||
hold will always go down instead of increasing)
|
||||
|
||||
Generic class for configuration parameter.
|
||||
|
||||
The ```config_decimal``` channel is of type ```config_decimal``` and supports the ```Number``` item.
|
||||
|
||||
### Output Control
|
||||
0 - disable completely
|
||||
|
||||
1 - enable local control
|
||||
|
||||
2 - enable central scene
|
||||
|
||||
3 - enable local and central scene
|
||||
|
||||
Generic class for configuration parameter.
|
||||
|
||||
The ```config_decimal``` channel is of type ```config_decimal``` and supports the ```Number``` item.
|
||||
|
||||
### Button Function
|
||||
0 - up/ON, down/OFF
|
||||
|
||||
1 - down/ON, up/OFF
|
||||
|
||||
2 - toggle (up or down are the same)
|
||||
|
||||
Generic class for configuration parameter.
|
||||
|
||||
The ```config_decimal``` channel is of type ```config_decimal``` and supports the ```Number``` item.
|
||||
|
||||
### Report Behavior
|
||||
0 - report on/off status and change LED indicator when
|
||||
|
||||
parameter 121 is set to 0 or 2
|
||||
|
||||
1 - Don't report on/off status is pressed and parameter
|
||||
|
||||
121 is set to 0 or 2
|
||||
|
||||
Generic class for configuration parameter.
|
||||
|
||||
The ```config_decimal``` channel is of type ```config_decimal``` and supports the ```Number``` item.
|
||||
|
||||
### Ramp Rate for Tap and Hub control
|
||||
0 - instant...
|
||||
|
||||
2 - Ramp Rate 2s
|
||||
|
||||
...
|
||||
|
||||
99 - Ramp Rate 99s
|
||||
|
||||
Generic class for configuration parameter.
|
||||
|
||||
The ```config_decimal``` channel is of type ```config_decimal``` and supports the ```Number``` item.
|
||||
|
||||
### Ramp Rate for press and hold
|
||||
1 - Ramp Rate 1s
|
||||
|
||||
...
|
||||
|
||||
4 - Ramp Rate 4s
|
||||
|
||||
...
|
||||
|
||||
99 - Ramp Rate 99s
|
||||
|
||||
Generic class for configuration parameter.
|
||||
|
||||
The ```config_decimal``` channel is of type ```config_decimal``` and supports the ```Number``` item.
|
||||
|
||||
### Minimum most dim setting
|
||||
0 - Disable
|
||||
|
||||
1 - Minimum value is 1
|
||||
|
||||
...
|
||||
|
||||
99 - Minimum value is 99
|
||||
|
||||
Generic class for configuration parameter.
|
||||
|
||||
The ```config_decimal``` channel is of type ```config_decimal``` and supports the ```Number``` item.
|
||||
|
||||
### Maximum most dim setting
|
||||
0 - Disable
|
||||
|
||||
1 - Maximum value is 1
|
||||
|
||||
...
|
||||
|
||||
100 - Maximum value is 100
|
||||
|
||||
Generic class for configuration parameter.
|
||||
|
||||
The ```config_decimal``` channel is of type ```config_decimal``` and supports the ```Number``` item.
|
||||
|
||||
### Custom brightness
|
||||
0 - Disable
|
||||
|
||||
1 - custom brightness level is 1
|
||||
|
||||
...
|
||||
|
||||
99 - custom brightness level is 99
|
||||
|
||||
Generic class for configuration parameter.
|
||||
|
||||
The ```config_decimal``` channel is of type ```config_decimal``` and supports the ```Number``` item.
|
||||
|
||||
|
||||
|
||||
## Device Configuration
|
||||
|
||||
The following table provides a summary of the 21 configuration parameters available in the ZWA037.
|
||||
Detailed information on each parameter can be found in the sections below.
|
||||
|
||||
| Param | Name | Description |
|
||||
|-------|-------|-------------|
|
||||
| 20 | Power Restore | Status after power failure |
|
||||
| 40 | Auto Turn Off Timer | Timer that auto turn off once turned on. |
|
||||
| 41 | Auto Turn On Timer | Timer that auto turn on once turned off. |
|
||||
| 80 | Instant Status Report | The command for status report. |
|
||||
| 82 | Association Control Settings | Setting command for association group. |
|
||||
| 83 | Led Indicator Settings | Select the indicator function for output. |
|
||||
| 84 | Led Indicator Color For Output | Select the indicator color for output. |
|
||||
| 85 | Led Indicator Color For Scene | Select the indicator color for scene. |
|
||||
| 86 | Led Brightness | Setting the brightness of indicator. |
|
||||
| 110 | Single Tap behavior | Select behavior when single tap. |
|
||||
| 111 | Double Tap behavior | Select behavior when double tap. |
|
||||
| 119 | External Switch Scene | Enable or disable the scene function of external(3-way) switch. |
|
||||
| 120 | External Switch Type | Select the external (3- way) switch's type. |
|
||||
| 121 | Output Control | Enable or disable output. |
|
||||
| 122 | Button Function | Select the paddle button functions. |
|
||||
| 123 | Report Behavior | Select behavior for report and indicator when output is disable. |
|
||||
| 125 | Ramp Rate for Tap and Hub contro | Setting ramp rate when tap to on off or controlled by HUB. |
|
||||
| 126 | Ramp Rate for press and hold | Setting ramp rate when press and hold the paddle. |
|
||||
| 131 | Minimum most dim setting | Setting the minimum value of dimmer. |
|
||||
| 132 | Maximum most dim setting | Setting the maximum value of dimmer. |
|
||||
| 133 | Custom brightness | Setting user custom brightness. |
|
||||
|
||||
### Parameter 20: Power Restore
|
||||
|
||||
Status after power failure
|
||||
0 - return to OFF
|
||||
|
||||
1 - return to ON
|
||||
|
||||
2 - returns to last known state when repowered
|
||||
Values in the range 0 to 2 may be set.
|
||||
|
||||
The manufacturer defined default value is ```2```.
|
||||
|
||||
This parameter has the configuration ID ```config_20_1``` and is of type ```INTEGER```.
|
||||
|
||||
|
||||
### Parameter 40: Auto Turn Off Timer
|
||||
|
||||
Timer that auto turn off once turned on.
|
||||
0 - disabled
|
||||
|
||||
1 - Auto turn off after 1s once turned on
|
||||
|
||||
...
|
||||
|
||||
65535 - Auto turn off after 65535s once turned on
|
||||
Values in the range 0 to 65535 may be set.
|
||||
|
||||
The manufacturer defined default value is ```0```.
|
||||
|
||||
This parameter has the configuration ID ```config_40_4``` and is of type ```INTEGER```.
|
||||
|
||||
|
||||
### Parameter 41: Auto Turn On Timer
|
||||
|
||||
Timer that auto turn on once turned off.
|
||||
0 - disabled
|
||||
|
||||
1 - Auto turn on after 1s once turned off
|
||||
|
||||
...
|
||||
|
||||
65535 - Auto turn on after 65535s once turned off
|
||||
Values in the range 0 to 65535 may be set.
|
||||
|
||||
The manufacturer defined default value is ```0```.
|
||||
|
||||
This parameter has the configuration ID ```config_41_4``` and is of type ```INTEGER```.
|
||||
|
||||
|
||||
### Parameter 80: Instant Status Report
|
||||
|
||||
The command for status report.
|
||||
0 - Nothing
|
||||
|
||||
1 - Basic Report
|
||||
|
||||
2 - Binary Switch Report
|
||||
Values in the range 0 to 2 may be set.
|
||||
|
||||
The manufacturer defined default value is ```2```.
|
||||
|
||||
This parameter has the configuration ID ```config_80_1``` and is of type ```INTEGER```.
|
||||
|
||||
|
||||
### Parameter 82: Association Control Settings
|
||||
|
||||
Setting command for association group.
|
||||
1 - Basic Set
|
||||
|
||||
2- Binary Switch SET
|
||||
Values in the range 1 to 2 may be set.
|
||||
|
||||
The manufacturer defined default value is ```1```.
|
||||
|
||||
This parameter has the configuration ID ```config_82_1``` and is of type ```INTEGER```.
|
||||
|
||||
|
||||
### Parameter 83: Led Indicator Settings
|
||||
|
||||
Select the indicator function for output.
|
||||
0 - disable completely
|
||||
|
||||
1 - Momentary blink
|
||||
|
||||
2 - LED follows status of output
|
||||
Values in the range 0 to 2 may be set.
|
||||
|
||||
The manufacturer defined default value is ```2```.
|
||||
|
||||
This parameter has the configuration ID ```config_83_1``` and is of type ```INTEGER```.
|
||||
|
||||
|
||||
### Parameter 84: Led Indicator Color For Output
|
||||
|
||||
Select the indicator color for output.
|
||||
0 - Disabled
|
||||
|
||||
1 - Red
|
||||
|
||||
2 - Blue
|
||||
|
||||
3- Green
|
||||
|
||||
4- Pink
|
||||
|
||||
5- Cyan
|
||||
|
||||
6- Purple
|
||||
|
||||
7- Orange
|
||||
|
||||
8- Yellow
|
||||
|
||||
9- White
|
||||
Values in the range 0 to 9 may be set.
|
||||
|
||||
The manufacturer defined default value is ```9```.
|
||||
|
||||
This parameter has the configuration ID ```config_84_1``` and is of type ```INTEGER```.
|
||||
|
||||
|
||||
### Parameter 85: Led Indicator Color For Scene
|
||||
|
||||
Select the indicator color for scene.
|
||||
0 - Disabled
|
||||
|
||||
1 - Red
|
||||
|
||||
2 - Blue
|
||||
|
||||
3- Green
|
||||
|
||||
4- Pink
|
||||
|
||||
5- Cyan
|
||||
|
||||
6- Purple
|
||||
|
||||
7- Orange
|
||||
|
||||
8- Yellow
|
||||
|
||||
9- White
|
||||
Values in the range 0 to 9 may be set.
|
||||
|
||||
The manufacturer defined default value is ```2```.
|
||||
|
||||
This parameter has the configuration ID ```config_85_1``` and is of type ```INTEGER```.
|
||||
|
||||
|
||||
### Parameter 86: Led Brightness
|
||||
|
||||
Setting the brightness of indicator.
|
||||
0 - Disable
|
||||
|
||||
1 - 1%
|
||||
|
||||
...
|
||||
|
||||
60 - 60%
|
||||
|
||||
...
|
||||
|
||||
100 - 100%
|
||||
Values in the range 0 to 100 may be set.
|
||||
|
||||
The manufacturer defined default value is ```60```.
|
||||
|
||||
This parameter has the configuration ID ```config_86_1``` and is of type ```INTEGER```.
|
||||
|
||||
|
||||
### Parameter 110: Single Tap behavior
|
||||
|
||||
Select behavior when single tap.
|
||||
0 - Last brightness level
|
||||
|
||||
1- Custom value from Param 133
|
||||
|
||||
2- Max brightness from Param 132
|
||||
|
||||
3- Full brightness at 100%
|
||||
Values in the range 0 to 3 may be set.
|
||||
|
||||
The manufacturer defined default value is ```0```.
|
||||
|
||||
This parameter has the configuration ID ```config_110_1``` and is of type ```INTEGER```.
|
||||
|
||||
|
||||
### Parameter 111: Double Tap behavior
|
||||
|
||||
Select behavior when double tap.
|
||||
0 - Full brightness at 99
|
||||
|
||||
...
|
||||
|
||||
1- Custom value from Param 133
|
||||
|
||||
2- Max brightness from Param 132
|
||||
|
||||
3- disabled
|
||||
Values in the range 0 to 3 may be set.
|
||||
|
||||
The manufacturer defined default value is ```0```.
|
||||
|
||||
This parameter has the configuration ID ```config_111_1``` and is of type ```INTEGER```.
|
||||
|
||||
|
||||
### Parameter 119: External Switch Scene
|
||||
|
||||
Enable or disable the scene function of external(3-way) switch.
|
||||
0 - Disable
|
||||
|
||||
1 - enable
|
||||
Values in the range 0 to 1 may be set.
|
||||
|
||||
The manufacturer defined default value is ```1```.
|
||||
|
||||
This parameter has the configuration ID ```config_119_1``` and is of type ```INTEGER```.
|
||||
|
||||
|
||||
### Parameter 120: External Switch Type
|
||||
|
||||
Select the external (3- way) switch's type.
|
||||
0 - toggle on or off only
|
||||
|
||||
1 - toggle switch/1x to toggle /2x use Param 132 or 133
|
||||
|
||||
/3x toggle dimmer loop ramp -> toggle once more to
|
||||
|
||||
stop dim
|
||||
|
||||
2 - momentary switch / 1x to toggle / 2x user Param 132
|
||||
|
||||
or 133/ Press and hold to dim up and down
|
||||
|
||||
3 - momentary switch / 1x quick toggle / 2x user Param
|
||||
|
||||
132 or 133 / Press and hold to dim up and down (but
|
||||
|
||||
after a double click to full brightness, then press and
|
||||
|
||||
hold will always go down instead of increasing)
|
||||
Values in the range 0 to 3 may be set.
|
||||
|
||||
The manufacturer defined default value is ```1```.
|
||||
|
||||
This parameter has the configuration ID ```config_120_1``` and is of type ```INTEGER```.
|
||||
|
||||
|
||||
### Parameter 121: Output Control
|
||||
|
||||
Enable or disable output.
|
||||
0 - disable completely
|
||||
|
||||
1 - enable local control
|
||||
|
||||
2 - enable central scene
|
||||
|
||||
3 - enable local and central scene
|
||||
Values in the range 0 to 3 may be set.
|
||||
|
||||
The manufacturer defined default value is ```3```.
|
||||
|
||||
This parameter has the configuration ID ```config_121_1``` and is of type ```INTEGER```.
|
||||
|
||||
|
||||
### Parameter 122: Button Function
|
||||
|
||||
Select the paddle button functions.
|
||||
0 - up/ON, down/OFF
|
||||
|
||||
1 - down/ON, up/OFF
|
||||
|
||||
2 - toggle (up or down are the same)
|
||||
Values in the range 0 to 2 may be set.
|
||||
|
||||
The manufacturer defined default value is ```0```.
|
||||
|
||||
This parameter has the configuration ID ```config_122_1``` and is of type ```INTEGER```.
|
||||
|
||||
|
||||
### Parameter 123: Report Behavior
|
||||
|
||||
Select behavior for report and indicator when output is disable.
|
||||
0 - report on/off status and change LED indicator when
|
||||
|
||||
parameter 121 is set to 0 or 2
|
||||
|
||||
1 - Don't report on/off status is pressed and parameter
|
||||
|
||||
121 is set to 0 or 2
|
||||
Values in the range 0 to 1 may be set.
|
||||
|
||||
The manufacturer defined default value is ```0```.
|
||||
|
||||
This parameter has the configuration ID ```config_123_1``` and is of type ```INTEGER```.
|
||||
|
||||
|
||||
### Parameter 125: Ramp Rate for Tap and Hub contro
|
||||
|
||||
Setting ramp rate when tap to on off or controlled by HUB.
|
||||
0 - instant...
|
||||
|
||||
2 - Ramp Rate 2s
|
||||
|
||||
...
|
||||
|
||||
99 - Ramp Rate 99s
|
||||
Values in the range 0 to 99 may be set.
|
||||
|
||||
The manufacturer defined default value is ```2```.
|
||||
|
||||
This parameter has the configuration ID ```config_125_1``` and is of type ```INTEGER```.
|
||||
|
||||
|
||||
### Parameter 126: Ramp Rate for press and hold
|
||||
|
||||
Setting ramp rate when press and hold the paddle.
|
||||
1 - Ramp Rate 1s
|
||||
|
||||
...
|
||||
|
||||
4 - Ramp Rate 4s
|
||||
|
||||
...
|
||||
|
||||
99 - Ramp Rate 99s
|
||||
Values in the range 1 to 99 may be set.
|
||||
|
||||
The manufacturer defined default value is ```4```.
|
||||
|
||||
This parameter has the configuration ID ```config_126_1``` and is of type ```INTEGER```.
|
||||
|
||||
|
||||
### Parameter 131: Minimum most dim setting
|
||||
|
||||
Setting the minimum value of dimmer.
|
||||
0 - Disable
|
||||
|
||||
1 - Minimum value is 1
|
||||
|
||||
...
|
||||
|
||||
99 - Minimum value is 99
|
||||
Values in the range 0 to 99 may be set.
|
||||
|
||||
The manufacturer defined default value is ```0```.
|
||||
|
||||
This parameter has the configuration ID ```config_131_1``` and is of type ```INTEGER```.
|
||||
|
||||
|
||||
### Parameter 132: Maximum most dim setting
|
||||
|
||||
Setting the maximum value of dimmer.
|
||||
0 - Disable
|
||||
|
||||
1 - Maximum value is 1
|
||||
|
||||
...
|
||||
|
||||
100 - Maximum value is 100
|
||||
Values in the range 0 to 100 may be set.
|
||||
|
||||
The manufacturer defined default value is ```100```.
|
||||
|
||||
This parameter has the configuration ID ```config_132_1``` and is of type ```INTEGER```.
|
||||
|
||||
|
||||
### Parameter 133: Custom brightness
|
||||
|
||||
Setting user custom brightness.
|
||||
0 - Disable
|
||||
|
||||
1 - custom brightness level is 1
|
||||
|
||||
...
|
||||
|
||||
99 - custom brightness level is 99
|
||||
Values in the range 0 to 99 may be set.
|
||||
|
||||
The manufacturer defined default value is ```0```.
|
||||
|
||||
This parameter has the configuration ID ```config_133_1``` and is of type ```INTEGER```.
|
||||
|
||||
|
||||
## Association Groups
|
||||
|
||||
Association groups allow the device to send unsolicited reports to the controller, or other devices in the network. Using association groups can allow you to eliminate polling, providing instant feedback of a device state change without unnecessary network traffic.
|
||||
|
||||
The ZWA037 supports 8 association groups.
|
||||
|
||||
### Group 1: Lifeline
|
||||
|
||||
The Lifeline association group reports device status to a hub and is not designed to control other devices directly. When using the Lineline group with a hub, in most cases, only the lifeline group will need to be configured and normally the hub will perform this automatically during the device initialisation.
|
||||
General: Lifeline
|
||||
Device Reset Locally Notification:
|
||||
|
||||
Issued when Factory Reset is performed.
|
||||
|
||||
Indicator Report:
|
||||
|
||||
Issued when included successfully.
|
||||
|
||||
Switch Multilevel Report:
|
||||
|
||||
Issued when output level is changed. (Determined by Parameter 80).
|
||||
|
||||
Basic Report:
|
||||
|
||||
Issued when output level is changed (Determined by Parameter80).
|
||||
|
||||
Central Scene Notification:
|
||||
|
||||
Issued when button press or hold or release. (Determined by Parameter
|
||||
|
||||
121).
|
||||
|
||||
Association group 1 supports 5 nodes.
|
||||
|
||||
### Group 2: Control: Key 1
|
||||
|
||||
Top button set ON/OFF
|
||||
Basic Set or Switch Binary Set: Issued when Top button press. (The command class is determined by Parameter 82)
|
||||
|
||||
Association group 2 supports 5 nodes.
|
||||
|
||||
### Group 3: Control: Key 1
|
||||
|
||||
Top button multilevel set
|
||||
Switch Multilevel Set:
|
||||
|
||||
Issued when Top button press or hold and release.
|
||||
|
||||
Association group 3 supports 5 nodes.
|
||||
|
||||
### Group 4: Control: Key 2
|
||||
|
||||
Bottom button set ON/OFF
|
||||
Basic Set or Switch Binary Set:
|
||||
|
||||
Issued when Bottom button press. (The command class is determined
|
||||
|
||||
by Parameter 82)
|
||||
|
||||
Association group 4 supports 5 nodes.
|
||||
|
||||
### Group 6: Control: Key 3
|
||||
|
||||
External switch set ON/OFF
|
||||
Basic Set or Switch Binary Set:
|
||||
|
||||
Issued when External Switch press. (The command class is determined
|
||||
|
||||
by Parameter 82).
|
||||
|
||||
Association group 6 supports 5 nodes.
|
||||
|
||||
### Group 7: Control: Key 3
|
||||
|
||||
External switch multilevel set
|
||||
Switch Multilevel Set:
|
||||
|
||||
Issued when External Switch press or hold and release.
|
||||
|
||||
Association group 7 supports 5 nodes.
|
||||
|
||||
### Group 8: Control: Key 4
|
||||
|
||||
Top and bottom button set ON/OFF
|
||||
Basic Set or Switch Binary Set:
|
||||
|
||||
Issued when Top/ Bottom button press. (The command class is
|
||||
|
||||
determined by Parameter 82).
|
||||
|
||||
Association group 8 supports 5 nodes.
|
||||
|
||||
### Group 9: Control: Key 4
|
||||
|
||||
Top and bottom button set multilevel set
|
||||
Switch Multilevel Set:
|
||||
|
||||
Issued when Top/Bottom button press or hold and release.
|
||||
|
||||
Association group 9 supports 5 nodes.
|
||||
|
||||
## Technical Information
|
||||
|
||||
### Endpoints
|
||||
|
||||
#### Endpoint 0
|
||||
|
||||
| Command Class | Comment |
|
||||
|---------------|---------|
|
||||
| COMMAND_CLASS_NO_OPERATION_V1| |
|
||||
| COMMAND_CLASS_BASIC_V1| |
|
||||
| COMMAND_CLASS_SWITCH_MULTILEVEL_V3| |
|
||||
| COMMAND_CLASS_SCENE_ACTIVATION_V1| |
|
||||
| COMMAND_CLASS_SCENE_ACTUATOR_CONF_V1| |
|
||||
| COMMAND_CLASS_TRANSPORT_SERVICE_V1| |
|
||||
| COMMAND_CLASS_ASSOCIATION_GRP_INFO_V1| |
|
||||
| COMMAND_CLASS_DEVICE_RESET_LOCALLY_V1| |
|
||||
| COMMAND_CLASS_CENTRAL_SCENE_V3| |
|
||||
| COMMAND_CLASS_ZWAVEPLUS_INFO_V1| |
|
||||
| COMMAND_CLASS_SUPERVISION_V1| |
|
||||
| COMMAND_CLASS_CONFIGURATION_V1| |
|
||||
| COMMAND_CLASS_MANUFACTURER_SPECIFIC_V1| |
|
||||
| COMMAND_CLASS_POWERLEVEL_V1| |
|
||||
| COMMAND_CLASS_FIRMWARE_UPDATE_MD_V1| |
|
||||
| COMMAND_CLASS_ASSOCIATION_V2| |
|
||||
| COMMAND_CLASS_VERSION_V2| |
|
||||
| COMMAND_CLASS_INDICATOR_V3| |
|
||||
| COMMAND_CLASS_MULTI_CHANNEL_ASSOCIATION_V3| |
|
||||
| COMMAND_CLASS_SECURITY_2_V1| |
|
||||
|
||||
### Documentation Links
|
||||
|
||||
* [Device Manual](https://opensmarthouse.org/zwavedatabase/1555/reference/ES_-_illumino_Dimmer_Switch_US_1.pdf)
|
||||
|
||||
---
|
||||
|
||||
Did you spot an error in the above definition or want to improve the content?
|
||||
You can [contribute to the database here](https://opensmarthouse.org/zwavedatabase/1555).
|
|
@ -61,12 +61,12 @@ Detailed information on each parameter can be found in the sections below.
|
|||
| Param | Name | Description |
|
||||
|-------|-------|-------------|
|
||||
| 3 | LED Light | Adjust LED light settings |
|
||||
| 7 | Dim Rate Adjustments (From Z-Wave Controller) | Number of steps or levels |
|
||||
| 8 | Dim Rate Adjustments (From Z-Wave Controller) | Timing of steps |
|
||||
| 9 | Dim Rate Adjustments (When Manually Controlled) | Number of steps or levels |
|
||||
| 10 | Dim Rate Adjustments (When Manually Controlled) | Timing of steps |
|
||||
| 11 | Dim Rate Adjustments (When Receiving All-On/Off Commands) | Number of steps or levels |
|
||||
| 12 | Dim Rate Adjustments (When Receiving All-On/Off Command | Timing of steps |
|
||||
| 7 | Dimmer Steps (Z-Wave) | Number of Dimmer Steps |
|
||||
| 8 | Dimmer Rate (Z-Wave) | Dimmer Steps Rate |
|
||||
| 9 | Dimmer Steps (Manual Control) | Number of Dimmer Steps |
|
||||
| 10 | Dimmer Rate (Manual Control) | Dimmer Steps Rate |
|
||||
| 11 | Dimmer Steps (All-On/All-Off) | Number Dimmer Steps |
|
||||
| 12 | Dimmer Rate (All-On/All-Off) | Dimmer Steps Rate |
|
||||
| | Switch All Mode | Set the mode for the switch when receiving SWITCH ALL commands |
|
||||
|
||||
### Parameter 3: LED Light
|
||||
|
@ -77,18 +77,18 @@ The following option values may be configured -:
|
|||
|
||||
| Value | Description |
|
||||
|--------|-------------|
|
||||
| 0 | default |
|
||||
| 1 | LED On when light is turned On |
|
||||
| 2 | LED always Off |
|
||||
| 0 | On when load is off |
|
||||
| 1 | On when load is on |
|
||||
| 2 | Alwasy off |
|
||||
|
||||
The manufacturer defined default value is ```0``` (default).
|
||||
The manufacturer defined default value is ```0``` (On when load is off).
|
||||
|
||||
This parameter has the configuration ID ```config_3_1``` and is of type ```INTEGER```.
|
||||
|
||||
|
||||
### Parameter 7: Dim Rate Adjustments (From Z-Wave Controller)
|
||||
### Parameter 7: Dimmer Steps (Z-Wave)
|
||||
|
||||
Number of steps or levels
|
||||
Number of Dimmer Steps
|
||||
|
||||
Values in the range 1 to 99 may be set.
|
||||
|
||||
|
@ -97,9 +97,9 @@ The manufacturer defined default value is ```1```.
|
|||
This parameter has the configuration ID ```config_7_1``` and is of type ```INTEGER```.
|
||||
|
||||
|
||||
### Parameter 8: Dim Rate Adjustments (From Z-Wave Controller)
|
||||
### Parameter 8: Dimmer Rate (Z-Wave)
|
||||
|
||||
Timing of steps
|
||||
Dimmer Steps Rate
|
||||
|
||||
Values in the range 1 to 255 may be set.
|
||||
|
||||
|
@ -108,9 +108,9 @@ The manufacturer defined default value is ```3```.
|
|||
This parameter has the configuration ID ```config_8_2``` and is of type ```INTEGER```.
|
||||
|
||||
|
||||
### Parameter 9: Dim Rate Adjustments (When Manually Controlled)
|
||||
### Parameter 9: Dimmer Steps (Manual Control)
|
||||
|
||||
Number of steps or levels
|
||||
Number of Dimmer Steps
|
||||
|
||||
Values in the range 1 to 99 may be set.
|
||||
|
||||
|
@ -119,9 +119,9 @@ The manufacturer defined default value is ```1```.
|
|||
This parameter has the configuration ID ```config_9_1``` and is of type ```INTEGER```.
|
||||
|
||||
|
||||
### Parameter 10: Dim Rate Adjustments (When Manually Controlled)
|
||||
### Parameter 10: Dimmer Rate (Manual Control)
|
||||
|
||||
Timing of steps
|
||||
Dimmer Steps Rate
|
||||
|
||||
Values in the range 1 to 255 may be set.
|
||||
|
||||
|
@ -130,9 +130,9 @@ The manufacturer defined default value is ```3```.
|
|||
This parameter has the configuration ID ```config_10_2``` and is of type ```INTEGER```.
|
||||
|
||||
|
||||
### Parameter 11: Dim Rate Adjustments (When Receiving All-On/Off Commands)
|
||||
### Parameter 11: Dimmer Steps (All-On/All-Off)
|
||||
|
||||
Number of steps or levels
|
||||
Number Dimmer Steps
|
||||
|
||||
Values in the range 1 to 99 may be set.
|
||||
|
||||
|
@ -141,9 +141,9 @@ The manufacturer defined default value is ```1```.
|
|||
This parameter has the configuration ID ```config_11_1``` and is of type ```INTEGER```.
|
||||
|
||||
|
||||
### Parameter 12: Dim Rate Adjustments (When Receiving All-On/Off Command
|
||||
### Parameter 12: Dimmer Rate (All-On/All-Off)
|
||||
|
||||
Timing of steps
|
||||
Dimmer Steps Rate
|
||||
|
||||
Values in the range 1 to 255 may be set.
|
||||
|
||||
|
@ -180,16 +180,18 @@ Z-Wave Plus Lifeline
|
|||
|
||||
Association group 1 supports 5 nodes.
|
||||
|
||||
### Group 2: Association Group 2
|
||||
### Group 2: Local Load
|
||||
|
||||
Supports Basic Set and is controlled with the local load
|
||||
|
||||
|
||||
Association group 2 supports 5 nodes.
|
||||
|
||||
### Group 3: Association Group 3
|
||||
### Group 3: Double Tap
|
||||
|
||||
Supports Basic Set and is controlled by double pressing the button
|
||||
|
||||
|
||||
Association group 3 supports 5 nodes.
|
||||
|
||||
## Technical Information
|
||||
|
|
|
@ -221,20 +221,22 @@ The 56590 supports 3 association groups.
|
|||
### Group 1: Lifeline
|
||||
|
||||
The Lifeline association group reports device status to a hub and is not designed to control other devices directly. When using the Lineline group with a hub, in most cases, only the lifeline group will need to be configured and normally the hub will perform this automatically during the device initialisation.
|
||||
Association Group 1 supports Lifeline, Multi-level Switch report
|
||||
Z-Wave Plus Lifeline
|
||||
|
||||
|
||||
Association group 1 supports 5 nodes.
|
||||
|
||||
### Group 2: Basic Set, controlled with local load
|
||||
### Group 2: Single Press
|
||||
|
||||
Basic Set - Controlled with the local load
|
||||
|
||||
Association Group 2 supports Basic Set and is controlled with the local load
|
||||
|
||||
Association group 2 supports 5 nodes.
|
||||
|
||||
### Group 3: Basic set, controlled by double pressing ON/OFF
|
||||
### Group 3: Double Tap
|
||||
|
||||
Basic Set - controlled by double pressing the ON/OFF button
|
||||
|
||||
Association Group 3 supports Basic Set and is controlled by double pressing the ON/OFF button
|
||||
|
||||
Association group 3 supports 5 nodes.
|
||||
|
||||
|
|
|
@ -71,85 +71,85 @@ Detailed information on each parameter can be found in the sections below.
|
|||
|
||||
| Param | Name | Description |
|
||||
|-------|-------|-------------|
|
||||
| 3 | LED Configuration | Set LED to desired status setting |
|
||||
| 4 | Invert Switch | Reverse the default On/Off setting |
|
||||
| 6 | Dim Rate | Slows down the rate at which the device ramps up and down |
|
||||
| 16 | Switch Mode | Mimics operation of a standard switch. No ramping up or down in operation |
|
||||
| 19 | Alternate Exclusion | When programmed, the default exclusion process will not exclude device from network. Prevents accidental removal of device from network |
|
||||
| 30 | Minimum Dim Threshold | User can custom set a Minimum dim level other than 1%. To be used when performance in lower range is not suitable in normal operation |
|
||||
| 31 | Maximum Dim Threshold | User can custom set a Maximum dim level other than 99%. To be used when performance in upper range is not suitable in normal operation |
|
||||
| 32 | Brightness Level | Dimmer will turn ON to this level |
|
||||
| 3 | LED Indicator | Set LED to desired status setting |
|
||||
| 4 | Inverted Orientation | Invert Switch Orientation |
|
||||
| 6 | Dim Rate | Adjust dimming rate |
|
||||
| 16 | Switch Mode | Mimics operation of a binary switch. |
|
||||
| 19 | Alternate Exclusion | Change from default exclusion setting. Prevents accidental removal of device from network |
|
||||
| 30 | Minimum Dim Threshold | Set custom minimum dimmer threshold |
|
||||
| 31 | Maximum Dim Threshold | Set custom maximum dimmer threshold |
|
||||
| 32 | Default Brightness Level | Dimmer will turn ON to this level |
|
||||
| 84 | Reset to Factory Default | Reset to Factory Default |
|
||||
|
||||
### Parameter 3: LED Configuration
|
||||
### Parameter 3: LED Indicator
|
||||
|
||||
Set LED to desired status setting
|
||||
Set LED to desired status setting
|
||||
Set LED to desired status settingThis is an advanced parameter and will therefore not show in the user interface without entering advanced mode.
|
||||
The following option values may be configured, in addition to values in the range 0 to 3 -:
|
||||
|
||||
| Value | Description |
|
||||
|--------|-------------|
|
||||
| 0 | LED is Off when switch is Off, and LED is On when switch is On |
|
||||
| 1 | LED is On when switch is Off, LED is off when switch is ON (guidelight |
|
||||
| 2 | LED is Off at all times |
|
||||
| 3 | LED is On at all times |
|
||||
| 0 | On when load is off |
|
||||
| 1 | On when load is on |
|
||||
| 2 | Always off |
|
||||
| 3 | Always on |
|
||||
|
||||
The manufacturer defined default value is ```0``` (LED is Off when switch is Off, and LED is On when switch is On).
|
||||
The manufacturer defined default value is ```0``` (On when load is off).
|
||||
|
||||
This parameter has the configuration ID ```config_3_1``` and is of type ```INTEGER```.
|
||||
|
||||
|
||||
### Parameter 4: Invert Switch
|
||||
|
||||
Reverse the default On/Off setting
|
||||
### Parameter 4: Inverted Orientation
|
||||
|
||||
Invert Switch Orientation
|
||||
This is an advanced parameter and will therefore not show in the user interface without entering advanced mode.
|
||||
The following option values may be configured, in addition to values in the range 0 to 1 -:
|
||||
|
||||
| Value | Description |
|
||||
|--------|-------------|
|
||||
| 0 | Mount the switch in the designed orientation |
|
||||
| 0 | Disable |
|
||||
| 1 | Mount the switch upside down |
|
||||
|
||||
The manufacturer defined default value is ```0``` (Mount the switch in the designed orientation).
|
||||
The manufacturer defined default value is ```0``` (Disable).
|
||||
|
||||
This parameter has the configuration ID ```config_4_1``` and is of type ```INTEGER```.
|
||||
|
||||
|
||||
### Parameter 6: Dim Rate
|
||||
|
||||
Slows down the rate at which the device ramps up and down
|
||||
|
||||
Adjust dimming rate
|
||||
This is an advanced parameter and will therefore not show in the user interface without entering advanced mode.
|
||||
The following option values may be configured, in addition to values in the range 0 to 1 -:
|
||||
|
||||
| Value | Description |
|
||||
|--------|-------------|
|
||||
| 0 | Normal Rate |
|
||||
| 1 | Slow dim rate |
|
||||
| 0 | Dim Quickly |
|
||||
| 1 | Dim Slowly |
|
||||
|
||||
The manufacturer defined default value is ```0``` (Normal Rate).
|
||||
The manufacturer defined default value is ```0``` (Dim Quickly).
|
||||
|
||||
This parameter has the configuration ID ```config_6_1``` and is of type ```INTEGER```.
|
||||
|
||||
|
||||
### Parameter 16: Switch Mode
|
||||
|
||||
Mimics operation of a standard switch. No ramping up or down in operation
|
||||
|
||||
Mimics operation of a binary switch.
|
||||
This is an advanced parameter and will therefore not show in the user interface without entering advanced mode.
|
||||
The following option values may be configured, in addition to values in the range 0 to 1 -:
|
||||
|
||||
| Value | Description |
|
||||
|--------|-------------|
|
||||
| 0 | Disabled |
|
||||
| 1 | Enabled |
|
||||
| 0 | Disable |
|
||||
| 1 | Enable |
|
||||
|
||||
The manufacturer defined default value is ```0``` (Disabled).
|
||||
The manufacturer defined default value is ```0``` (Disable).
|
||||
|
||||
This parameter has the configuration ID ```config_16_1``` and is of type ```INTEGER```.
|
||||
|
||||
|
||||
### Parameter 19: Alternate Exclusion
|
||||
|
||||
When programmed, the default exclusion process will not exclude device from network. Prevents accidental removal of device from network
|
||||
Change from default exclusion setting. Prevents accidental removal of device from network
|
||||
|
||||
The following option values may be configured, in addition to values in the range 0 to 1 -:
|
||||
|
||||
|
@ -165,7 +165,7 @@ This parameter has the configuration ID ```config_19_1``` and is of type ```INTE
|
|||
|
||||
### Parameter 30: Minimum Dim Threshold
|
||||
|
||||
User can custom set a Minimum dim level other than 1%. To be used when performance in lower range is not suitable in normal operation
|
||||
Set custom minimum dimmer threshold
|
||||
|
||||
Values in the range 1 to 99 may be set.
|
||||
|
||||
|
@ -176,7 +176,7 @@ This parameter has the configuration ID ```config_30_1``` and is of type ```INTE
|
|||
|
||||
### Parameter 31: Maximum Dim Threshold
|
||||
|
||||
User can custom set a Maximum dim level other than 99%. To be used when performance in upper range is not suitable in normal operation
|
||||
Set custom maximum dimmer threshold
|
||||
|
||||
Values in the range 1 to 99 may be set.
|
||||
|
||||
|
@ -185,7 +185,7 @@ The manufacturer defined default value is ```99```.
|
|||
This parameter has the configuration ID ```config_31_1``` and is of type ```INTEGER```.
|
||||
|
||||
|
||||
### Parameter 32: Brightness Level
|
||||
### Parameter 32: Default Brightness Level
|
||||
|
||||
Dimmer will turn ON to this level
|
||||
|
||||
|
@ -216,7 +216,7 @@ This parameter has the configuration ID ```config_84_1``` and is of type ```INTE
|
|||
|
||||
Association groups allow the device to send unsolicited reports to the controller, or other devices in the network. Using association groups can allow you to eliminate polling, providing instant feedback of a device state change without unnecessary network traffic.
|
||||
|
||||
The ZW3012 supports 1 association group.
|
||||
The ZW3012 supports 3 association groups.
|
||||
|
||||
### Group 1: Lifeline
|
||||
|
||||
|
@ -226,6 +226,18 @@ Z-Wave Plus Lifeline
|
|||
|
||||
Association group 1 supports 5 nodes.
|
||||
|
||||
### Group 2: Single Press
|
||||
|
||||
Basic Set - Controlled with the local load
|
||||
|
||||
Association group 2 supports 5 nodes.
|
||||
|
||||
### Group 3: Double Tap
|
||||
|
||||
Basic Set - Controlled by pressing On/Off
|
||||
|
||||
Association group 3 supports 5 nodes.
|
||||
|
||||
## Technical Information
|
||||
|
||||
### Endpoints
|
||||
|
|
|
@ -23,6 +23,8 @@ From a hardware side we’ve upgraded our Z-Wave module for better distance and
|
|||
|
||||
From a firmware side, this switch has it all (literally). Scene control, notifications (flashes if your garage door is left open) and can be configured on any HUB.
|
||||
|
||||
Updated instructions here: https://community.inovelli.com/t/resources-red-series-dimmer-switch-manual/7306#
|
||||
|
||||
### Inclusion Information
|
||||
|
||||
Once the inclusion process has started press the config button 3X and the LED bar will flash blue. If the switch was included successfully the bar will turn GREEN, however if the switch was not included successfully it will turn RED.
|
||||
|
@ -468,13 +470,21 @@ This parameter has the configuration ID ```config_51_1``` and is of type ```INTE
|
|||
### Parameter 52: Enable "smart bulb" mode
|
||||
|
||||
Enable "smart bulb" mode
|
||||
Smart bulb mode: If set to 1, power will output maximum % when dimmer is on to optimize performance with smart bulbs.
|
||||
The following option values may be configured, in addition to values in the range 0 to 1 -:
|
||||
Switch Mode
|
||||
0 = Normal operation
|
||||
|
||||
1 = On /Off Mode - Switch will either be in the state of 0% power or 99% power, not allowing dimming. Useful for non-dimmable bulbs or other unique loads
|
||||
|
||||
2 = Smart Bulb - Switch always at 99% for use with loads like an Inovelli Bulb or Philips Hue Bulb. Use in conjunction with associations or scenes to achieve an awesome smart bulb experience
|
||||
|
||||
Requires firmware 1.47 and above.
|
||||
The following option values may be configured, in addition to values in the range 0 to 2 -:
|
||||
|
||||
| Value | Description |
|
||||
|--------|-------------|
|
||||
| 0 | normal bulb |
|
||||
| 1 | smart bulb |
|
||||
| 1 | On/Off Mode |
|
||||
| 2 | Smart Bulb |
|
||||
|
||||
The manufacturer defined default value is ```0``` (normal bulb).
|
||||
|
||||
|
|
|
@ -28,6 +28,8 @@ All parts together represents a smart home controller for Z-Wave.
|
|||
|
||||
The entire infrastructure is maintained and developed by [Z-Wave.Me](https://www.z-wave.me/) with the help of a large community.
|
||||
|
||||
A detailed step-by-step description of the binding configuration is also available on the [Z-Wave.Me Support site](https://help.z-wave.me/en/knowledge_base/art/149/cat/88/)
|
||||
|
||||
Please note the **known issues** below.
|
||||
|
||||
### Approach
|
||||
|
|
|
@ -6,23 +6,23 @@
|
|||
|
||||
<channel-type id="fek-temperature-type">
|
||||
<item-type>Number:Temperature</item-type>
|
||||
<label>FFK Temperature</label>
|
||||
<label>FEK Temperature</label>
|
||||
<state readOnly="true" pattern="%.1f %unit%"/>
|
||||
</channel-type>
|
||||
|
||||
<channel-type id="fek-temperature-setpoint-type">
|
||||
<item-type>Number:Temperature</item-type>
|
||||
<label>FFK Temperature Set Point</label>
|
||||
<label>FEK Temperature Set Point</label>
|
||||
<state readOnly="true" pattern="%.1f %unit%"/>
|
||||
</channel-type>
|
||||
<channel-type id="fek-humidity-type">
|
||||
<item-type>Number:Dimensionless</item-type>
|
||||
<label>FFK Humidity</label>
|
||||
<label>FEK Humidity</label>
|
||||
<state readOnly="true" pattern="%.1f %unit%"/>
|
||||
</channel-type>
|
||||
<channel-type id="fek-dewpoint-type">
|
||||
<item-type>Number:Temperature</item-type>
|
||||
<label>FFK Dewpoint</label>
|
||||
<label>FEK Dewpoint</label>
|
||||
<state readOnly="true" pattern="%.1f %unit%"/>
|
||||
</channel-type>
|
||||
<channel-type id="outdoor-temperature-type">
|
||||
|
|
|
@ -22,16 +22,10 @@
|
|||
</parameter>
|
||||
<parameter name="apiUrl" type="text" required="true">
|
||||
<label>API-URL</label>
|
||||
<description>URL to cloud API-service.</description>
|
||||
<description>URL to cloud API-service and Socket-Notification.</description>
|
||||
<context>url</context>
|
||||
<advanced>true</advanced>
|
||||
<default>https://OWD5-OJ001-App.ojelectronics.com/api</default>
|
||||
</parameter>
|
||||
<parameter name="refreshDelayInSeconds" type="integer" required="true" min="15" unit="s">
|
||||
<label>Refresh Delay</label>
|
||||
<description>Refresh delay in seconds.</description>
|
||||
<advanced>true</advanced>
|
||||
<default>30</default>
|
||||
<default>https://OWD5-OJ001-App.ojelectronics.com</default>
|
||||
</parameter>
|
||||
<parameter name="customerId" type="integer" required="true">
|
||||
<label>Customer ID</label>
|
||||
|
|
|
@ -0,0 +1,158 @@
|
|||
<?xml version="1.0" encoding="UTF-8"?>
|
||||
<thing:thing-descriptions bindingId="speedtest"
|
||||
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
|
||||
xmlns:thing="https://openhab.org/schemas/thing-description/v1.0.0"
|
||||
xsi:schemaLocation="https://openhab.org/schemas/thing-description/v1.0.0 https://openhab.org/schemas/thing-description-1.0.0.xsd">
|
||||
|
||||
<thing-type id="speedtest">
|
||||
<label>Speedtest Binding</label>
|
||||
<description>Binding for Ookla Speedtest (https://www.speedtest.net/)</description>
|
||||
<channels>
|
||||
<channel id="server" typeId="server"/>
|
||||
|
||||
<channel id="pingJitter" typeId="pingJitter"/>
|
||||
<channel id="pingLatency" typeId="pingLatency"/>
|
||||
|
||||
<channel id="downloadBandwidth" typeId="downloadBandwidth"/>
|
||||
<channel id="downloadBytes" typeId="downloadBytes"/>
|
||||
<channel id="downloadElapsed" typeId="downloadElapsed"/>
|
||||
|
||||
<channel id="uploadBandwidth" typeId="uploadBandwidth"/>
|
||||
<channel id="uploadBytes" typeId="uploadBytes"/>
|
||||
<channel id="uploadElapsed" typeId="uploadElapsed"/>
|
||||
|
||||
<channel id="isp" typeId="isp"/>
|
||||
|
||||
<channel id="interfaceInternalIp" typeId="interfaceInternalIp"/>
|
||||
<channel id="interfaceExternalIp" typeId="interfaceExternalIp"/>
|
||||
|
||||
<channel id="resultUrl" typeId="resultUrl"/>
|
||||
|
||||
<channel id="triggerTest" typeId="triggerTest"/>
|
||||
</channels>
|
||||
<properties>
|
||||
<property name="Server List 1"></property>
|
||||
<property name="Server List 2"></property>
|
||||
<property name="Server List 3"></property>
|
||||
<property name="Server List 4"></property>
|
||||
<property name="Server List 5"></property>
|
||||
<property name="Server List 6"></property>
|
||||
<property name="Server List 7"></property>
|
||||
<property name="Server List 8"></property>
|
||||
<property name="Server List 9"></property>
|
||||
<property name="Server List 10"></property>
|
||||
</properties>
|
||||
<config-description>
|
||||
<parameter-group name="config-info">
|
||||
<label>Speedtest Configuration</label>
|
||||
</parameter-group>
|
||||
<parameter name="refreshInterval" type="integer" required="true" groupName="config-info">
|
||||
<label>Refresh Interval</label>
|
||||
<description>How often to test network speed</description>
|
||||
<options>
|
||||
<option value="15">15 minutes</option>
|
||||
<option value="30">30 minutes</option>
|
||||
<option value="60">Every Hour</option>
|
||||
<option value="360">Every 6 Hours</option>
|
||||
<option value="720">Every 12 Hours</option>
|
||||
<option value="1440">Once a Day</option>
|
||||
<option value="0">Don't test automatically</option>
|
||||
</options>
|
||||
<default>60</default>
|
||||
</parameter>
|
||||
<parameter name="execPath" type="text" required="false" groupName="config-info">
|
||||
<label>Executable Path</label>
|
||||
<description>The path of the Ookla Speedtest executable. Linux machines may leave this blank and it defaults to
|
||||
`/usr/bin/speedtest`.</description>
|
||||
</parameter>
|
||||
<parameter name="serverID" type="text" required="false" groupName="config-info">
|
||||
<label>Server ID</label>
|
||||
<description>Optional: A specific server that shall be used for testing. You can pick the server ID from the "Thing
|
||||
Properties". If this is left blank the best option will be selected by Ookla.</description>
|
||||
</parameter>
|
||||
</config-description>
|
||||
</thing-type>
|
||||
<channel-type id="server">
|
||||
<item-type>String</item-type>
|
||||
<label>Server</label>
|
||||
<description>The remote server that the Speedtest was run against</description>
|
||||
<state readOnly="true"></state>
|
||||
</channel-type>
|
||||
<channel-type id="pingJitter" advanced="true">
|
||||
<item-type>Number:Time</item-type>
|
||||
<label>Ping Jitter</label>
|
||||
<description>Ping Jitter - the variation in the response time</description>
|
||||
<state readOnly="true" pattern="%.2f ms"></state>
|
||||
</channel-type>
|
||||
<channel-type id="pingLatency">
|
||||
<item-type>Number:Time</item-type>
|
||||
<label>Ping Latency</label>
|
||||
<description>Ping Latency - the reaction time of your internet connection</description>
|
||||
<state readOnly="true" pattern="%.2f ms"></state>
|
||||
</channel-type>
|
||||
<channel-type id="downloadBandwidth">
|
||||
<item-type>Number:DataTransferRate</item-type>
|
||||
<label>Download Bandwidth</label>
|
||||
<description>Download bandwidth, e.g. in Mbit/s</description>
|
||||
<state readOnly="true" pattern="%.2f Mbit/s"></state>
|
||||
</channel-type>
|
||||
<channel-type id="downloadBytes" advanced="true">
|
||||
<item-type>Number:DataAmount</item-type>
|
||||
<label>Downloaded Bytes</label>
|
||||
<description>Amount of data that was used for the last upload bandwidth test</description>
|
||||
<state readOnly="true" pattern="%.0f MB"></state>
|
||||
</channel-type>
|
||||
<channel-type id="downloadElapsed" advanced="true">
|
||||
<item-type>Number:Time</item-type>
|
||||
<label>Download Elapsed Time</label>
|
||||
<description>Time spent for the last download bandwidth test</description>
|
||||
<state readOnly="true" pattern="%.2f s"></state>
|
||||
</channel-type>
|
||||
<channel-type id="uploadBandwidth">
|
||||
<item-type>Number:DataTransferRate</item-type>
|
||||
<label>Upload Bandwidth</label>
|
||||
<description>Upload bandwidth, e.g. in Mbit/s</description>
|
||||
<state readOnly="true" pattern="%.2f Mbit/s"></state>
|
||||
</channel-type>
|
||||
<channel-type id="uploadBytes" advanced="true">
|
||||
<item-type>Number:DataAmount</item-type>
|
||||
<label>Uploaded Bytes</label>
|
||||
<description>Amount of data that was used for the last upload bandwidth test</description>
|
||||
<state readOnly="true" pattern="%.0f MB"></state>
|
||||
</channel-type>
|
||||
<channel-type id="uploadElapsed" advanced="true">
|
||||
<item-type>Number:Time</item-type>
|
||||
<label>Upload Elapsed Time</label>
|
||||
<description>Time spent for the last upload bandwidth test</description>
|
||||
<state readOnly="true" pattern="%.2f s"></state>
|
||||
</channel-type>
|
||||
<channel-type id="isp">
|
||||
<item-type>String</item-type>
|
||||
<label>ISP</label>
|
||||
<description>Your Internet Service Provider (ISP) as calculated by Ookla</description>
|
||||
<state readOnly="true"></state>
|
||||
</channel-type>
|
||||
<channel-type id="interfaceInternalIp" advanced="true">
|
||||
<item-type>String</item-type>
|
||||
<label>Interface Internal IP</label>
|
||||
<description>IP address of the internal interface that was used for the test</description>
|
||||
<state readOnly="true"></state>
|
||||
</channel-type>
|
||||
<channel-type id="interfaceExternalIp" advanced="true">
|
||||
<item-type>String</item-type>
|
||||
<label>Interface External IP</label>
|
||||
<description>IP address of the external interface that was used for the test</description>
|
||||
<state readOnly="true"></state>
|
||||
</channel-type>
|
||||
<channel-type id="resultUrl">
|
||||
<item-type>String</item-type>
|
||||
<label>Result URL</label>
|
||||
<description>The URL to the Speedtest results in HTML on the Ookla webserver</description>
|
||||
<state readOnly="true"></state>
|
||||
</channel-type>
|
||||
<channel-type id="triggerTest" advanced="true">
|
||||
<item-type>Switch</item-type>
|
||||
<label>Trigger Test</label>
|
||||
<description>Trigger in order to run Speedtest manually</description>
|
||||
</channel-type>
|
||||
</thing:thing-descriptions>
|
|
@ -0,0 +1,597 @@
|
|||
<?xml version="1.0" encoding="UTF-8"?>
|
||||
<thing:thing-descriptions bindingId="zwave"
|
||||
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
|
||||
xmlns:thing="https://openhab.org/schemas/thing-description/v1.0.0"
|
||||
xsi:schemaLocation="https://openhab.org/schemas/thing-description/v1.0.0
|
||||
https://openhab.org/schemas/thing-description/v1.0.0">
|
||||
|
||||
<thing-type id="aeotec_zwa037_00_000" listed="false">
|
||||
<label>ZWA037 Aeotec Illumino Dimmer Switch</label>
|
||||
<description><![CDATA[
|
||||
Aeotec Illumino Dimmer Switch<br /> <h1>Overview</h1><p>This product can be operated in any Z-Wave™ network with other Z-Wave Plus™ certified devices from other manufacturers. All non-battery operated nodes within the network will act as repeaters regardless of vendor to increase reliability of the network. Each module is designed to act as a repeater, which will re-transmit a radio frequency (RF) signal by routing the signal around obstacles and radio dead spots to ensure that the signal is received at its intended destination. ZWA037-A is a security enabled Z-Wave Plus™ device. A security Enabled Z-Wave Plus™</p><p>Controller must be used in order to fully utilize the product. </p> <br /> <h2>Inclusion Information</h2><p>Inclusion 1x tap</p><p>Out of network</p><p>Send NIF for network pairing/ inclusion (white LED quick flashes). If pairing is successful, the LED will turn to solid green for 2s, then deactivates.</p><p>In network</p><p>Trigger to send Central Scene 1x tap scene.</p><p>ON/OFF control of output load. </p> <br /> <h2>Exclusion Information</h2><p>Exclusion 3x tap</p><p>In network Send NIF for network unpairing/ exclusion (purple LED flashes).</p><p>Out of network</p><p>Trigger to send Central Scene 3x tap scene. </p> <br /> <h2>Wakeup Information</h2><p><br /></p>
|
||||
]]></description>
|
||||
<category>WallSwitch</category>
|
||||
|
||||
<!-- CHANNEL DEFINITIONS -->
|
||||
<channels>
|
||||
<channel id="switch_dimmer" typeId="switch_dimmer">
|
||||
<label>Dimmer</label>
|
||||
<properties>
|
||||
<property name="binding:*:PercentType">COMMAND_CLASS_SWITCH_MULTILEVEL</property>
|
||||
<property name="binding:Command:OnOffType">COMMAND_CLASS_SWITCH_MULTILEVEL</property>
|
||||
</properties>
|
||||
</channel>
|
||||
<channel id="scene_number" typeId="scene_number">
|
||||
<label>Scene Number</label>
|
||||
<properties>
|
||||
<property name="binding:*:DecimalType">COMMAND_CLASS_SCENE_ACTIVATION</property>
|
||||
</properties>
|
||||
</channel>
|
||||
<channel id="scene_number" typeId="scene_number">
|
||||
<label>Scene Number</label>
|
||||
<properties>
|
||||
<property name="binding:*:DecimalType">COMMAND_CLASS_CENTRAL_SCENE</property>
|
||||
</properties>
|
||||
</channel>
|
||||
<channel id="config_decimal_param20" typeId="config_decimal">
|
||||
<label>Power Restore</label>
|
||||
<properties>
|
||||
<property name="binding:*:DecimalType">COMMAND_CLASS_CONFIGURATION;paramter=20</property>
|
||||
</properties>
|
||||
</channel>
|
||||
<channel id="config_decimal_param40" typeId="config_decimal">
|
||||
<label>Auto Turn Off Timer</label>
|
||||
<properties>
|
||||
<property name="binding:*:DecimalType">COMMAND_CLASS_CONFIGURATION;parameter=40</property>
|
||||
</properties>
|
||||
</channel>
|
||||
<channel id="config_decimal_param41" typeId="config_decimal">
|
||||
<label>Auto Turn On Timer</label>
|
||||
<properties>
|
||||
<property name="binding:*:DecimalType">COMMAND_CLASS_CONFIGURATION;parameter=41</property>
|
||||
</properties>
|
||||
</channel>
|
||||
<channel id="config_decimal_param80" typeId="config_decimal">
|
||||
<label>Instant Status Report</label>
|
||||
<properties>
|
||||
<property name="binding:*:DecimalType">COMMAND_CLASS_CONFIGURATION;parameter=80</property>
|
||||
</properties>
|
||||
</channel>
|
||||
<channel id="config_decimal_param82" typeId="config_decimal">
|
||||
<label>Association Control Settings</label>
|
||||
<properties>
|
||||
<property name="binding:*:DecimalType">COMMAND_CLASS_CONFIGURATION;parameter=82</property>
|
||||
</properties>
|
||||
</channel>
|
||||
<channel id="config_decimal_param83" typeId="config_decimal">
|
||||
<label>Led Indicator Settings</label>
|
||||
<properties>
|
||||
<property name="binding:*:DecimalType">COMMAND_CLASS_CONFIGURATION;parameter=83</property>
|
||||
</properties>
|
||||
</channel>
|
||||
<channel id="config_decimal_param84" typeId="config_decimal">
|
||||
<label>Led Indicator Color For Output</label>
|
||||
<properties>
|
||||
<property name="binding:*:DecimalType">COMMAND_CLASS_CONFIGURATION;parameter=84</property>
|
||||
</properties>
|
||||
</channel>
|
||||
<channel id="config_decimal_param85" typeId="config_decimal">
|
||||
<label>Led Indicator Color For Scene</label>
|
||||
<properties>
|
||||
<property name="binding:*:DecimalType">COMMAND_CLASS_CONFIGURATION;parameter=85</property>
|
||||
</properties>
|
||||
</channel>
|
||||
<channel id="config_decimal_param86" typeId="config_decimal">
|
||||
<label>Led Brightness</label>
|
||||
<properties>
|
||||
<property name="binding:*:DecimalType">COMMAND_CLASS_CONFIGURATION;parameter=86</property>
|
||||
</properties>
|
||||
</channel>
|
||||
<channel id="config_decimal_param110" typeId="config_decimal">
|
||||
<label>Single Tap behavior</label>
|
||||
<properties>
|
||||
<property name="binding:*:DecimalType">COMMAND_CLASS_CONFIGURATION;parameter=110</property>
|
||||
</properties>
|
||||
</channel>
|
||||
<channel id="config_decimal_param111" typeId="config_decimal">
|
||||
<label>Double Tap behavior</label>
|
||||
<properties>
|
||||
<property name="binding:*:DecimalType">COMMAND_CLASS_CONFIGURATION;parameter=111</property>
|
||||
</properties>
|
||||
</channel>
|
||||
<channel id="config_decimal_param119" typeId="config_decimal">
|
||||
<label>External Switch Scene</label>
|
||||
<properties>
|
||||
<property name="binding:*:DecimalType">COMMAND_CLASS_CONFIGURATION;parameter=119</property>
|
||||
</properties>
|
||||
</channel>
|
||||
<channel id="config_decimal_param120" typeId="config_decimal">
|
||||
<label>External Switch Type</label>
|
||||
<properties>
|
||||
<property name="binding:*:DecimalType">COMMAND_CLASS_CONFIGURATION;paramater=120</property>
|
||||
</properties>
|
||||
</channel>
|
||||
<channel id="config_decimal_param121" typeId="config_decimal">
|
||||
<label>Output Control</label>
|
||||
<properties>
|
||||
<property name="binding:*:DecimalType">COMMAND_CLASS_CONFIGURATION;parameter=121</property>
|
||||
</properties>
|
||||
</channel>
|
||||
<channel id="config_decimal_param122" typeId="config_decimal">
|
||||
<label>Button Function</label>
|
||||
<properties>
|
||||
<property name="binding:*:DecimalType">COMMAND_CLASS_CONFIGURATION;parameter=122</property>
|
||||
</properties>
|
||||
</channel>
|
||||
<channel id="config_decimal_param123" typeId="config_decimal">
|
||||
<label>Report Behavior</label>
|
||||
<properties>
|
||||
<property name="binding:*:DecimalType">COMMAND_CLASS_CONFIGURATION;parameter=123</property>
|
||||
</properties>
|
||||
</channel>
|
||||
<channel id="config_decimal_param125" typeId="config_decimal">
|
||||
<label>Ramp Rate for Tap and Hub control</label>
|
||||
<properties>
|
||||
<property name="binding:*:DecimalType">COMMAND_CLASS_CONFIGURATION;parameter=125</property>
|
||||
</properties>
|
||||
</channel>
|
||||
<channel id="config_decimal_param126" typeId="config_decimal">
|
||||
<label>Ramp Rate for press and hold</label>
|
||||
<properties>
|
||||
<property name="binding:*:DecimalType">COMMAND_CLASS_CONFIGURATION;parameter=126</property>
|
||||
</properties>
|
||||
</channel>
|
||||
<channel id="config_decimal_param131" typeId="config_decimal">
|
||||
<label>Minimum most dim setting</label>
|
||||
<properties>
|
||||
<property name="binding:*:DecimalType">COMMAND_CLASS_CONFIGURATION;parameter=131</property>
|
||||
</properties>
|
||||
</channel>
|
||||
<channel id="config_decimal_param132" typeId="config_decimal">
|
||||
<label>Maximum most dim setting</label>
|
||||
<properties>
|
||||
<property name="binding:*:DecimalType">COMMAND_CLASS_CONFIGURATION;paramater=132</property>
|
||||
</properties>
|
||||
</channel>
|
||||
<channel id="config_decimal_param133" typeId="config_decimal">
|
||||
<label>Custom brightness</label>
|
||||
<properties>
|
||||
<property name="binding:*:DecimalType">COMMAND_CLASS_CONFIGURATION;parameter=133</property>
|
||||
</properties>
|
||||
</channel>
|
||||
</channels>
|
||||
|
||||
<!-- DEVICE PROPERTY DEFINITIONS -->
|
||||
<properties>
|
||||
<property name="vendor">Aeotec Limited</property>
|
||||
<property name="modelId">ZWA037</property>
|
||||
<property name="manufacturerId">0371</property>
|
||||
<property name="manufacturerRef">0103:0025</property>
|
||||
<property name="dbReference">1555</property>
|
||||
<property name="defaultAssociations">1</property>
|
||||
</properties>
|
||||
|
||||
<!-- CONFIGURATION DESCRIPTIONS -->
|
||||
<config-description>
|
||||
|
||||
<!-- GROUP DEFINITIONS -->
|
||||
<parameter-group name="configuration">
|
||||
<context>setup</context>
|
||||
<label>Configuration Parameters</label>
|
||||
</parameter-group>
|
||||
|
||||
<parameter-group name="association">
|
||||
<context>link</context>
|
||||
<label>Association Groups</label>
|
||||
</parameter-group>
|
||||
|
||||
<!-- PARAMETER DEFINITIONS -->
|
||||
<parameter name="config_20_1" type="integer" groupName="configuration">
|
||||
<label>20: Power Restore</label>
|
||||
<description><![CDATA[
|
||||
Status after power failure<br /> <h1>Overview</h1><p>0 - return to OFF</p><p>1 - return to ON</p><p>2 - returns to last known state when repowered </p>
|
||||
]]></description>
|
||||
<default>2</default>
|
||||
<limitToOptions>false</limitToOptions>
|
||||
</parameter>
|
||||
|
||||
<parameter name="config_40_4" type="integer" groupName="configuration">
|
||||
<label>40: Auto Turn Off Timer</label>
|
||||
<description><![CDATA[
|
||||
Timer that auto turn off once turned on.<br /> <h1>Overview</h1><p>0 - disabled</p><p>1 - Auto turn off after 1s once turned on</p><p>...</p><p>65535 - Auto turn off after 65535s once turned on</p>
|
||||
]]></description>
|
||||
<default>0</default>
|
||||
<limitToOptions>false</limitToOptions>
|
||||
</parameter>
|
||||
|
||||
<parameter name="config_41_4" type="integer" groupName="configuration">
|
||||
<label>41: Auto Turn On Timer</label>
|
||||
<description><![CDATA[
|
||||
Timer that auto turn on once turned off.<br /> <h1>Overview</h1><p>0 - disabled</p><p>1 - Auto turn on after 1s once turned off</p><p>...</p><p>65535 - Auto turn on after 65535s once turned off</p>
|
||||
]]></description>
|
||||
<default>0</default>
|
||||
<limitToOptions>false</limitToOptions>
|
||||
</parameter>
|
||||
|
||||
<parameter name="config_80_1" type="integer" groupName="configuration">
|
||||
<label>80: Instant Status Report</label>
|
||||
<description><![CDATA[
|
||||
The command for status report.<br /> <h1>Overview</h1><p>0 - Nothing</p><p>1 - Basic Report</p><p>2 - Binary Switch Report</p>
|
||||
]]></description>
|
||||
<default>2</default>
|
||||
<limitToOptions>false</limitToOptions>
|
||||
</parameter>
|
||||
|
||||
<parameter name="config_82_1" type="integer" groupName="configuration">
|
||||
<label>82: Association Control Settings</label>
|
||||
<description><![CDATA[
|
||||
Setting command for association group.<br /> <h1>Overview</h1><p> 1 - Basic Set</p><p>2- Binary Switch SET </p>
|
||||
]]></description>
|
||||
<default>1</default>
|
||||
<limitToOptions>false</limitToOptions>
|
||||
</parameter>
|
||||
|
||||
<parameter name="config_83_1" type="integer" groupName="configuration">
|
||||
<label>83: Led Indicator Settings</label>
|
||||
<description><![CDATA[
|
||||
Select the indicator function for output.<br /> <h1>Overview</h1><p>0 - disable completely</p><p>1 - Momentary blink</p><p>2 - LED follows status of output</p>
|
||||
]]></description>
|
||||
<default>2</default>
|
||||
<limitToOptions>false</limitToOptions>
|
||||
</parameter>
|
||||
|
||||
<parameter name="config_84_1" type="integer" groupName="configuration">
|
||||
<label>84: Led Indicator Color For Output</label>
|
||||
<description><![CDATA[
|
||||
Select the indicator color for output.<br /> <h1>Overview</h1><p>0 - Disabled</p><p>1 - Red</p><p>2 - Blue</p><p>3- Green</p><p>4- Pink</p><p>5- Cyan</p><p>6- Purple</p><p>7- Orange</p><p>8- Yellow</p><p>9- White</p>
|
||||
]]></description>
|
||||
<default>9</default>
|
||||
<limitToOptions>false</limitToOptions>
|
||||
</parameter>
|
||||
|
||||
<parameter name="config_85_1" type="integer" groupName="configuration">
|
||||
<label>85: Led Indicator Color For Scene</label>
|
||||
<description><![CDATA[
|
||||
Select the indicator color for scene.<br /> <h1>Overview</h1><p>0 - Disabled</p><p>1 - Red</p><p>2 - Blue</p><p>3- Green</p><p>4- Pink</p><p>5- Cyan</p><p>6- Purple</p><p>7- Orange</p><p>8- Yellow</p><p>9- White </p>
|
||||
]]></description>
|
||||
<default>2</default>
|
||||
<limitToOptions>false</limitToOptions>
|
||||
</parameter>
|
||||
|
||||
<parameter name="config_86_1" type="integer" groupName="configuration">
|
||||
<label>86: Led Brightness</label>
|
||||
<description><![CDATA[
|
||||
Setting the brightness of indicator.<br /> <h1>Overview</h1><p>0 - Disable</p><p>1 - 1%</p><p>...</p><p>60 - 60%</p><p>...</p><p>100 - 100%</p>
|
||||
]]></description>
|
||||
<default>60</default>
|
||||
<limitToOptions>false</limitToOptions>
|
||||
</parameter>
|
||||
|
||||
<parameter name="config_110_1" type="integer" groupName="configuration">
|
||||
<label>110: Single Tap behavior</label>
|
||||
<description><![CDATA[
|
||||
Select behavior when single tap.<br /> <h1>Overview</h1><p>0 - Last brightness level</p><p>1- Custom value from Param 133</p><p>2- Max brightness from Param 132</p><p>3- Full brightness at 100% </p>
|
||||
]]></description>
|
||||
<default>0</default>
|
||||
<limitToOptions>false</limitToOptions>
|
||||
</parameter>
|
||||
|
||||
<parameter name="config_111_1" type="integer" groupName="configuration">
|
||||
<label>111: Double Tap behavior</label>
|
||||
<description><![CDATA[
|
||||
Select behavior when double tap.<br /> <h1>Overview</h1><p>0 - Full brightness at 99</p><p>...</p><p>1- Custom value from Param 133</p><p>2- Max brightness from Param 132</p><p>3- disabled </p>
|
||||
]]></description>
|
||||
<default>0</default>
|
||||
<limitToOptions>false</limitToOptions>
|
||||
</parameter>
|
||||
|
||||
<parameter name="config_119_1" type="integer" groupName="configuration">
|
||||
<label>119: External Switch Scene</label>
|
||||
<description><![CDATA[
|
||||
Enable or disable the scene function of external(3-way) switch.<br /> <h1>Overview</h1><p>0 - Disable</p><p>1 - enable </p>
|
||||
]]></description>
|
||||
<default>1</default>
|
||||
<limitToOptions>false</limitToOptions>
|
||||
</parameter>
|
||||
|
||||
<parameter name="config_120_1" type="integer" groupName="configuration">
|
||||
<label>120: External Switch Type</label>
|
||||
<description><![CDATA[
|
||||
Select the external (3- way) switch's type.<br /> <h1>Overview</h1><p>0 - toggle on or off only</p><p>1 - toggle switch/1x to toggle /2x use Param 132 or 133</p><p>/3x toggle dimmer loop ramp -> toggle once more to</p><p>stop dim</p><p>2 - momentary switch / 1x to toggle / 2x user Param 132</p><p>or 133/ Press and hold to dim up and down</p><p>3 - momentary switch / 1x quick toggle / 2x user Param</p><p>132 or 133 / Press and hold to dim up and down (but</p><p>after a double click to full brightness, then press and</p><p>hold will always go down instead of increasing)</p>
|
||||
]]></description>
|
||||
<default>1</default>
|
||||
<limitToOptions>false</limitToOptions>
|
||||
</parameter>
|
||||
|
||||
<parameter name="config_121_1" type="integer" groupName="configuration">
|
||||
<label>121: Output Control</label>
|
||||
<description><![CDATA[
|
||||
Enable or disable output.<br /> <h1>Overview</h1><p>0 - disable completely</p><p>1 - enable local control</p><p>2 - enable central scene</p><p>3 - enable local and central scene</p>
|
||||
]]></description>
|
||||
<default>3</default>
|
||||
<limitToOptions>false</limitToOptions>
|
||||
</parameter>
|
||||
|
||||
<parameter name="config_122_1" type="integer" groupName="configuration">
|
||||
<label>122: Button Function</label>
|
||||
<description><![CDATA[
|
||||
Select the paddle button functions.<br /> <h1>Overview</h1><p>0 - up/ON, down/OFF</p><p>1 - down/ON, up/OFF</p><p>2 - toggle (up or down are the same) </p>
|
||||
]]></description>
|
||||
<default>0</default>
|
||||
<limitToOptions>false</limitToOptions>
|
||||
</parameter>
|
||||
|
||||
<parameter name="config_123_1" type="integer" groupName="configuration">
|
||||
<label>123: Report Behavior</label>
|
||||
<description><![CDATA[
|
||||
Select behavior for report and indicator when output is disable.<br /> <h1>Overview</h1><p>0 - report on/off status and change LED indicator when</p><p>parameter 121 is set to 0 or 2</p><p>1 - Don't report on/off status is pressed and parameter</p><p>121 is set to 0 or 2 </p>
|
||||
]]></description>
|
||||
<default>0</default>
|
||||
<limitToOptions>false</limitToOptions>
|
||||
</parameter>
|
||||
|
||||
<parameter name="config_125_1" type="integer" groupName="configuration">
|
||||
<label>125: Ramp Rate for Tap and Hub contro</label>
|
||||
<description><![CDATA[
|
||||
Setting ramp rate when tap to on off or controlled by HUB.<br /> <h1>Overview</h1><p>0 - instant...</p><p>2 - Ramp Rate 2s</p><p>...</p><p>99 - Ramp Rate 99s</p>
|
||||
]]></description>
|
||||
<default>2</default>
|
||||
<limitToOptions>false</limitToOptions>
|
||||
</parameter>
|
||||
|
||||
<parameter name="config_126_1" type="integer" groupName="configuration">
|
||||
<label>126: Ramp Rate for press and hold</label>
|
||||
<description><![CDATA[
|
||||
Setting ramp rate when press and hold the paddle.<br /> <h1>Overview</h1><p>1 - Ramp Rate 1s</p><p>...</p><p>4 - Ramp Rate 4s</p><p>...</p><p>99 - Ramp Rate 99s </p>
|
||||
]]></description>
|
||||
<default>4</default>
|
||||
<limitToOptions>false</limitToOptions>
|
||||
</parameter>
|
||||
|
||||
<parameter name="config_131_1" type="integer" groupName="configuration">
|
||||
<label>131: Minimum most dim setting</label>
|
||||
<description><![CDATA[
|
||||
Setting the minimum value of dimmer.<br /> <h1>Overview</h1><p>0 - Disable</p><p>1 - Minimum value is 1</p><p>...</p><p>99 - Minimum value is 99</p>
|
||||
]]></description>
|
||||
<default>0</default>
|
||||
<limitToOptions>false</limitToOptions>
|
||||
</parameter>
|
||||
|
||||
<parameter name="config_132_1" type="integer" groupName="configuration">
|
||||
<label>132: Maximum most dim setting</label>
|
||||
<description><![CDATA[
|
||||
Setting the maximum value of dimmer.<br /> <h1>Overview</h1><p>0 - Disable</p><p>1 - Maximum value is 1</p><p>...</p><p>100 - Maximum value is 100 </p>
|
||||
]]></description>
|
||||
<default>100</default>
|
||||
<limitToOptions>false</limitToOptions>
|
||||
</parameter>
|
||||
|
||||
<parameter name="config_133_1" type="integer" groupName="configuration">
|
||||
<label>133: Custom brightness</label>
|
||||
<description><![CDATA[
|
||||
Setting user custom brightness.<br /> <h1>Overview</h1><p>0 - Disable</p><p>1 - custom brightness level is 1</p><p>...</p><p>99 - custom brightness level is 99</p>
|
||||
]]></description>
|
||||
<default>0</default>
|
||||
<limitToOptions>false</limitToOptions>
|
||||
</parameter>
|
||||
|
||||
<!-- ASSOCIATION DEFINITIONS -->
|
||||
<parameter name="group_1" type="text" groupName="association" multiple="true">
|
||||
<label>1: Lifeline</label>
|
||||
<description><![CDATA[
|
||||
General: Lifeline<br /> <h1>Overview</h1><p>Device Reset Locally Notification:</p><p>Issued when Factory Reset is performed.</p><p>Indicator Report:</p><p>Issued when included successfully.</p><p>Switch Multilevel Report:</p><p>Issued when output level is changed. (Determined by Parameter 80).</p><p>Basic Report:</p><p>Issued when output level is changed (Determined by Parameter80).</p><p>Central Scene Notification:</p><p>Issued when button press or hold or release. (Determined by Parameter</p><p>121). </p>
|
||||
]]></description>
|
||||
<multipleLimit>5</multipleLimit>
|
||||
</parameter>
|
||||
|
||||
<parameter name="group_2" type="text" groupName="association" multiple="true">
|
||||
<label>2: Control: Key 1</label>
|
||||
<description><![CDATA[
|
||||
Top button set ON/OFF<br /> <h1>Overview</h1><p>Basic Set or Switch Binary Set: Issued when Top button press. (The command class is determined by Parameter 82) <br /></p>
|
||||
]]></description>
|
||||
<multipleLimit>5</multipleLimit>
|
||||
</parameter>
|
||||
|
||||
<parameter name="group_3" type="text" groupName="association" multiple="true">
|
||||
<label>3: Control: Key 1</label>
|
||||
<description><![CDATA[
|
||||
Top button multilevel set<br /> <h1>Overview</h1><p>Switch Multilevel Set:</p><p>Issued when Top button press or hold and release. </p>
|
||||
]]></description>
|
||||
<multipleLimit>5</multipleLimit>
|
||||
</parameter>
|
||||
|
||||
<parameter name="group_4" type="text" groupName="association" multiple="true">
|
||||
<label>4: Control: Key 2</label>
|
||||
<description><![CDATA[
|
||||
Bottom button set ON/OFF<br /> <h1>Overview</h1><p>Basic Set or Switch Binary Set:</p><p>Issued when Bottom button press. (The command class is determined</p><p>by Parameter 82)</p>
|
||||
]]></description>
|
||||
<multipleLimit>5</multipleLimit>
|
||||
</parameter>
|
||||
|
||||
<parameter name="group_6" type="text" groupName="association" multiple="true">
|
||||
<label>6: Control: Key 3</label>
|
||||
<description><![CDATA[
|
||||
External switch set ON/OFF<br /> <h1>Overview</h1><p>Basic Set or Switch Binary Set:</p><p>Issued when External Switch press. (The command class is determined</p><p>by Parameter 82). </p>
|
||||
]]></description>
|
||||
<multipleLimit>5</multipleLimit>
|
||||
</parameter>
|
||||
|
||||
<parameter name="group_7" type="text" groupName="association" multiple="true">
|
||||
<label>7: Control: Key 3</label>
|
||||
<description><![CDATA[
|
||||
External switch multilevel set<br /> <h1>Overview</h1><p>Switch Multilevel Set:</p><p>Issued when External Switch press or hold and release.</p>
|
||||
]]></description>
|
||||
<multipleLimit>5</multipleLimit>
|
||||
</parameter>
|
||||
|
||||
<parameter name="group_8" type="text" groupName="association" multiple="true">
|
||||
<label>8: Control: Key 4</label>
|
||||
<description><![CDATA[
|
||||
Top and bottom button set ON/OFF<br /> <h1>Overview</h1><p>Basic Set or Switch Binary Set:</p><p>Issued when Top/ Bottom button press. (The command class is</p><p>determined by Parameter 82). </p>
|
||||
]]></description>
|
||||
<multipleLimit>5</multipleLimit>
|
||||
</parameter>
|
||||
|
||||
<parameter name="group_9" type="text" groupName="association" multiple="true">
|
||||
<label>9: Control: Key 4</label>
|
||||
<description><![CDATA[
|
||||
Top and bottom button set multilevel set<br /> <h1>Overview</h1><p>Switch Multilevel Set:</p><p>Issued when Top/Bottom button press or hold and release.</p>
|
||||
]]></description>
|
||||
<multipleLimit>5</multipleLimit>
|
||||
</parameter>
|
||||
|
||||
<!-- STATIC DEFINITIONS -->
|
||||
<parameter name="node_id" type="integer" min="1" max="232" readOnly="true" required="true">
|
||||
<label>Node ID</label>
|
||||
<advanced>true</advanced>
|
||||
</parameter>
|
||||
|
||||
</config-description>
|
||||
|
||||
</thing-type>
|
||||
|
||||
<channel-type id="aeotec_zwa037_00_000_config_decimal_param40">
|
||||
<item-type>Number</item-type>
|
||||
<label>Auto Turn Off Timer</label>
|
||||
<description>Timer that auto turn off once turned on.</description>
|
||||
<state pattern="%s">
|
||||
</state>
|
||||
</channel-type>
|
||||
|
||||
<channel-type id="aeotec_zwa037_00_000_config_decimal_param41">
|
||||
<item-type>Number</item-type>
|
||||
<label>Auto Turn On Timer</label>
|
||||
<description>Timer that auto turn on once turned off.</description>
|
||||
<state pattern="%s">
|
||||
</state>
|
||||
</channel-type>
|
||||
|
||||
<channel-type id="aeotec_zwa037_00_000_config_decimal_param80">
|
||||
<item-type>Number</item-type>
|
||||
<label>Instant Status Report</label>
|
||||
<description>The command for status report.</description>
|
||||
<state pattern="%s">
|
||||
</state>
|
||||
</channel-type>
|
||||
|
||||
<channel-type id="aeotec_zwa037_00_000_config_decimal_param82">
|
||||
<item-type>Number</item-type>
|
||||
<label>Association Control Settings</label>
|
||||
<description>Setting command for association group.</description>
|
||||
<state pattern="%s">
|
||||
</state>
|
||||
</channel-type>
|
||||
|
||||
<channel-type id="aeotec_zwa037_00_000_config_decimal_param83">
|
||||
<item-type>Number</item-type>
|
||||
<label>Led Indicator Settings</label>
|
||||
<description>Select the indicator function for output.</description>
|
||||
<state pattern="%s">
|
||||
</state>
|
||||
</channel-type>
|
||||
|
||||
<channel-type id="aeotec_zwa037_00_000_config_decimal_param84">
|
||||
<item-type>Number</item-type>
|
||||
<label>Led Indicator Color For Output</label>
|
||||
<description>Select the indicator color for output.</description>
|
||||
<state pattern="%s">
|
||||
</state>
|
||||
</channel-type>
|
||||
|
||||
<channel-type id="aeotec_zwa037_00_000_config_decimal_param85">
|
||||
<item-type>Number</item-type>
|
||||
<label>Led Indicator Color For Scene</label>
|
||||
<description>Select the indicator color for scene.</description>
|
||||
<state pattern="%s">
|
||||
</state>
|
||||
</channel-type>
|
||||
|
||||
<channel-type id="aeotec_zwa037_00_000_config_decimal_param86">
|
||||
<item-type>Number</item-type>
|
||||
<label>Led Brightness</label>
|
||||
<description>Setting the brightness of indicator.</description>
|
||||
<state pattern="%s">
|
||||
</state>
|
||||
</channel-type>
|
||||
|
||||
<channel-type id="aeotec_zwa037_00_000_config_decimal_param110">
|
||||
<item-type>Number</item-type>
|
||||
<label>Single Tap behavior</label>
|
||||
<description>Select behavior when single tap.</description>
|
||||
<state pattern="%s">
|
||||
</state>
|
||||
</channel-type>
|
||||
|
||||
<channel-type id="aeotec_zwa037_00_000_config_decimal_param111">
|
||||
<item-type>Number</item-type>
|
||||
<label>Double Tap behavior</label>
|
||||
<description>Select behavior when double tap.</description>
|
||||
<state pattern="%s">
|
||||
</state>
|
||||
</channel-type>
|
||||
|
||||
<channel-type id="aeotec_zwa037_00_000_config_decimal_param119">
|
||||
<item-type>Number</item-type>
|
||||
<label>External Switch Scene</label>
|
||||
<description>Enable or disable the scene function of external(3-way) switch.</description>
|
||||
<state pattern="%s">
|
||||
</state>
|
||||
</channel-type>
|
||||
|
||||
<channel-type id="aeotec_zwa037_00_000_config_decimal_param121">
|
||||
<item-type>Number</item-type>
|
||||
<label>Output Control</label>
|
||||
<description>Enable or disable output.</description>
|
||||
<state pattern="%s">
|
||||
</state>
|
||||
</channel-type>
|
||||
|
||||
<channel-type id="aeotec_zwa037_00_000_config_decimal_param122">
|
||||
<item-type>Number</item-type>
|
||||
<label>Button Function</label>
|
||||
<description>Select the paddle button functions.</description>
|
||||
<state pattern="%s">
|
||||
</state>
|
||||
</channel-type>
|
||||
|
||||
<channel-type id="aeotec_zwa037_00_000_config_decimal_param123">
|
||||
<item-type>Number</item-type>
|
||||
<label>Report Behavior</label>
|
||||
<description>Select behavior for report and indicator when output is disable.</description>
|
||||
<state pattern="%s">
|
||||
</state>
|
||||
</channel-type>
|
||||
|
||||
<channel-type id="aeotec_zwa037_00_000_config_decimal_param125">
|
||||
<item-type>Number</item-type>
|
||||
<label>Ramp Rate for Tap and Hub contro</label>
|
||||
<description>Setting ramp rate when tap to on off or controlled by HUB.</description>
|
||||
<state pattern="%s">
|
||||
</state>
|
||||
</channel-type>
|
||||
|
||||
<channel-type id="aeotec_zwa037_00_000_config_decimal_param126">
|
||||
<item-type>Number</item-type>
|
||||
<label>Ramp Rate for press and hold</label>
|
||||
<description>Setting ramp rate when press and hold the paddle.</description>
|
||||
<state pattern="%s">
|
||||
</state>
|
||||
</channel-type>
|
||||
|
||||
<channel-type id="aeotec_zwa037_00_000_config_decimal_param131">
|
||||
<item-type>Number</item-type>
|
||||
<label>Minimum most dim setting</label>
|
||||
<description>Setting the minimum value of dimmer.</description>
|
||||
<state pattern="%s">
|
||||
</state>
|
||||
</channel-type>
|
||||
|
||||
<channel-type id="aeotec_zwa037_00_000_config_decimal_param133">
|
||||
<item-type>Number</item-type>
|
||||
<label>Custom brightness</label>
|
||||
<description>Setting user custom brightness.</description>
|
||||
<state pattern="%s">
|
||||
</state>
|
||||
</channel-type>
|
||||
|
||||
</thing:thing-descriptions>
|
|
@ -36,7 +36,7 @@ Plug-in Smart Dimmer<br /> <h1>Overview</h1><p>Jasco Z-Wave Plug-In Smart Dimmer
|
|||
<property name="manufacturerId">0063</property>
|
||||
<property name="manufacturerRef">5044:3039</property>
|
||||
<property name="dbReference">1516</property>
|
||||
<property name="defaultAssociations">1</property>
|
||||
<property name="defaultAssociations">1,3</property>
|
||||
</properties>
|
||||
|
||||
<!-- CONFIGURATION DESCRIPTIONS -->
|
||||
|
@ -61,51 +61,63 @@ Adjust LED light settings<br /> <h1>Overview</h1><p>When shipped from the factor
|
|||
]]></description>
|
||||
<default>0</default>
|
||||
<options>
|
||||
<option value="0">default</option>
|
||||
<option value="1">LED On when light is turned On</option>
|
||||
<option value="2">LED always Off</option>
|
||||
<option value="0">On when load is off</option>
|
||||
<option value="1">On when load is on</option>
|
||||
<option value="2">Alwasy off</option>
|
||||
</options>
|
||||
<limitToOptions>false</limitToOptions>
|
||||
</parameter>
|
||||
|
||||
<parameter name="config_7_1" type="integer" groupName="configuration">
|
||||
<label>7: Dim Rate Adjustments (From Z-Wave Controller)</label>
|
||||
<description>Number of steps or levels</description>
|
||||
<label>7: Dimmer Steps (Z-Wave)</label>
|
||||
<description><![CDATA[
|
||||
Number of Dimmer Steps<br /> <h1>Overview</h1><p><br /></p>
|
||||
]]></description>
|
||||
<default>1</default>
|
||||
<limitToOptions>false</limitToOptions>
|
||||
</parameter>
|
||||
|
||||
<parameter name="config_8_2" type="integer" groupName="configuration">
|
||||
<label>8: Dim Rate Adjustments (From Z-Wave Controller)</label>
|
||||
<description>Timing of steps</description>
|
||||
<label>8: Dimmer Rate (Z-Wave)</label>
|
||||
<description><![CDATA[
|
||||
Dimmer Steps Rate<br /> <h1>Overview</h1><p><br /></p>
|
||||
]]></description>
|
||||
<default>3</default>
|
||||
<limitToOptions>false</limitToOptions>
|
||||
</parameter>
|
||||
|
||||
<parameter name="config_9_1" type="integer" groupName="configuration">
|
||||
<label>9: Dim Rate Adjustments (When Manually Controlled)</label>
|
||||
<description>Number of steps or levels</description>
|
||||
<label>9: Dimmer Steps (Manual Control)</label>
|
||||
<description><![CDATA[
|
||||
Number of Dimmer Steps<br /> <h1>Overview</h1><p><br /></p>
|
||||
]]></description>
|
||||
<default>1</default>
|
||||
<limitToOptions>false</limitToOptions>
|
||||
</parameter>
|
||||
|
||||
<parameter name="config_10_2" type="integer" groupName="configuration">
|
||||
<label>10: Dim Rate Adjustments (When Manually Controlled)</label>
|
||||
<description>Timing of steps</description>
|
||||
<label>10: Dimmer Rate (Manual Control)</label>
|
||||
<description><![CDATA[
|
||||
Dimmer Steps Rate<br /> <h1>Overview</h1><p><br /></p>
|
||||
]]></description>
|
||||
<default>3</default>
|
||||
<limitToOptions>false</limitToOptions>
|
||||
</parameter>
|
||||
|
||||
<parameter name="config_11_1" type="integer" groupName="configuration">
|
||||
<label>11: Dim Rate Adjustments (When Receiving All-On/Off Commands)</label>
|
||||
<description>Number of steps or levels</description>
|
||||
<label>11: Dimmer Steps (All-On/All-Off)</label>
|
||||
<description><![CDATA[
|
||||
Number Dimmer Steps<br /> <h1>Overview</h1><p><br /></p>
|
||||
]]></description>
|
||||
<default>1</default>
|
||||
<limitToOptions>false</limitToOptions>
|
||||
</parameter>
|
||||
|
||||
<parameter name="config_12_2" type="integer" groupName="configuration">
|
||||
<label>12: Dim Rate Adjustments (When Receiving All-On/Off Command</label>
|
||||
<description>Timing of steps</description>
|
||||
<label>12: Dimmer Rate (All-On/All-Off)</label>
|
||||
<description><![CDATA[
|
||||
Dimmer Steps Rate<br /> <h1>Overview</h1><p><br /></p>
|
||||
]]></description>
|
||||
<default>3</default>
|
||||
<limitToOptions>false</limitToOptions>
|
||||
</parameter>
|
||||
|
@ -120,14 +132,18 @@ Z-Wave Plus Lifeline<br /> <h1>Overview</h1><p><br /></p>
|
|||
</parameter>
|
||||
|
||||
<parameter name="group_2" type="text" groupName="association" multiple="true">
|
||||
<label>2: Association Group 2</label>
|
||||
<description>Supports Basic Set and is controlled with the local load</description>
|
||||
<label>2: Local Load</label>
|
||||
<description><![CDATA[
|
||||
Supports Basic Set and is controlled with the local load<br /> <h1>Overview</h1><p><br /></p>
|
||||
]]></description>
|
||||
<multipleLimit>5</multipleLimit>
|
||||
</parameter>
|
||||
|
||||
<parameter name="group_3" type="text" groupName="association" multiple="true">
|
||||
<label>3: Association Group 3</label>
|
||||
<description>Supports Basic Set and is controlled by double pressing the button</description>
|
||||
<label>3: Double Tap</label>
|
||||
<description><![CDATA[
|
||||
Supports Basic Set and is controlled by double pressing the button<br /> <h1>Overview</h1><p><br /></p>
|
||||
]]></description>
|
||||
<multipleLimit>5</multipleLimit>
|
||||
</parameter>
|
||||
|
||||
|
|
|
@ -36,7 +36,7 @@ In-Wall No Neutral Smart Dimmer<br /> <h1>Overview</h1><p>In-Wall No Neutral Sma
|
|||
<property name="manufacturerId">0063</property>
|
||||
<property name="manufacturerRef">4944:3334</property>
|
||||
<property name="dbReference">1498</property>
|
||||
<property name="defaultAssociations">1</property>
|
||||
<property name="defaultAssociations">1,3</property>
|
||||
</properties>
|
||||
|
||||
<!-- CONFIGURATION DESCRIPTIONS -->
|
||||
|
@ -141,20 +141,24 @@ In-Wall No Neutral Smart Dimmer<br /> <h1>Overview</h1><p>In-Wall No Neutral Sma
|
|||
<parameter name="group_1" type="text" groupName="association" multiple="true">
|
||||
<label>1: Lifeline</label>
|
||||
<description><![CDATA[
|
||||
Association Group 1 supports Lifeline, Multi-level Switch report<br /> <h1>Overview</h1><p><br /></p>
|
||||
Z-Wave Plus Lifeline<br /> <h1>Overview</h1><p><br /></p>
|
||||
]]></description>
|
||||
<multipleLimit>5</multipleLimit>
|
||||
</parameter>
|
||||
|
||||
<parameter name="group_2" type="text" groupName="association" multiple="true">
|
||||
<label>2: Basic Set, controlled with local load</label>
|
||||
<description>Association Group 2 supports Basic Set and is controlled with the local load</description>
|
||||
<label>2: Single Press</label>
|
||||
<description><![CDATA[
|
||||
Basic Set - Controlled with the local load<br /> <h1>Overview</h1><p><br /></p>
|
||||
]]></description>
|
||||
<multipleLimit>5</multipleLimit>
|
||||
</parameter>
|
||||
|
||||
<parameter name="group_3" type="text" groupName="association" multiple="true">
|
||||
<label>3: Basic set, controlled by double pressing ON/OFF</label>
|
||||
<description>Association Group 3 supports Basic Set and is controlled by double pressing the ON/OFF button</description>
|
||||
<label>3: Double Tap</label>
|
||||
<description><![CDATA[
|
||||
Basic Set - controlled by double pressing the ON/OFF button<br /> <h1>Overview</h1><p><br /></p>
|
||||
]]></description>
|
||||
<multipleLimit>5</multipleLimit>
|
||||
</parameter>
|
||||
|
||||
|
|
|
@ -36,7 +36,7 @@ In-Wall No Neutral Smart Dimmer<br /> <h1>Overview</h1><p>In-Wall No Neutral Sma
|
|||
<property name="manufacturerId">0063</property>
|
||||
<property name="manufacturerRef">4944:3333,4944:3334</property>
|
||||
<property name="dbReference">1344</property>
|
||||
<property name="defaultAssociations">1</property>
|
||||
<property name="defaultAssociations">1,3</property>
|
||||
</properties>
|
||||
|
||||
<!-- CONFIGURATION DESCRIPTIONS -->
|
||||
|
@ -55,59 +55,63 @@ In-Wall No Neutral Smart Dimmer<br /> <h1>Overview</h1><p>In-Wall No Neutral Sma
|
|||
|
||||
<!-- PARAMETER DEFINITIONS -->
|
||||
<parameter name="config_3_1" type="integer" groupName="configuration">
|
||||
<label>3: LED Configuration</label>
|
||||
<label>3: LED Indicator</label>
|
||||
<description><![CDATA[
|
||||
Set LED to desired status setting<br /> <h1>Overview</h1><p>Set LED to desired status setting</p>
|
||||
]]></description>
|
||||
<default>0</default>
|
||||
<options>
|
||||
<option value="0">LED is Off when switch is Off, and LED is On when switch is On</option>
|
||||
<option value="1">LED is On when switch is Off, LED is off when switch is ON (guidelight</option>
|
||||
<option value="2">LED is Off at all times</option>
|
||||
<option value="3">LED is On at all times</option>
|
||||
<option value="0">On when load is off</option>
|
||||
<option value="1">On when load is on</option>
|
||||
<option value="2">Always off</option>
|
||||
<option value="3">Always on</option>
|
||||
</options>
|
||||
<advanced>true</advanced>
|
||||
</parameter>
|
||||
|
||||
<parameter name="config_4_1" type="integer" groupName="configuration">
|
||||
<label>4: Invert Switch</label>
|
||||
<label>4: Inverted Orientation</label>
|
||||
<description><![CDATA[
|
||||
Reverse the default On/Off setting<br /> <h1>Overview</h1><p><br /></p>
|
||||
Invert Switch Orientation<br /> <h1>Overview</h1><p><br /></p>
|
||||
]]></description>
|
||||
<default>0</default>
|
||||
<options>
|
||||
<option value="0">Mount the switch in the designed orientation</option>
|
||||
<option value="0">Disable</option>
|
||||
<option value="1">Mount the switch upside down</option>
|
||||
</options>
|
||||
<advanced>true</advanced>
|
||||
</parameter>
|
||||
|
||||
<parameter name="config_6_1" type="integer" groupName="configuration">
|
||||
<label>6: Dim Rate</label>
|
||||
<description><![CDATA[
|
||||
Slows down the rate at which the device ramps up and down<br /> <h1>Overview</h1><p><br /></p>
|
||||
Adjust dimming rate<br /> <h1>Overview</h1><p><br /></p>
|
||||
]]></description>
|
||||
<default>0</default>
|
||||
<options>
|
||||
<option value="0">Normal Rate</option>
|
||||
<option value="1">Slow dim rate</option>
|
||||
<option value="0">Dim Quickly</option>
|
||||
<option value="1">Dim Slowly</option>
|
||||
</options>
|
||||
<advanced>true</advanced>
|
||||
</parameter>
|
||||
|
||||
<parameter name="config_16_1" type="integer" groupName="configuration">
|
||||
<label>16: Switch Mode</label>
|
||||
<description><![CDATA[
|
||||
Mimics operation of a standard switch. No ramping up or down in operation<br /> <h1>Overview</h1><p><br /></p>
|
||||
Mimics operation of a binary switch.<br /> <h1>Overview</h1><p><br /></p>
|
||||
]]></description>
|
||||
<default>0</default>
|
||||
<options>
|
||||
<option value="0">Disabled</option>
|
||||
<option value="1">Enabled</option>
|
||||
<option value="0">Disable</option>
|
||||
<option value="1">Enable</option>
|
||||
</options>
|
||||
<advanced>true</advanced>
|
||||
</parameter>
|
||||
|
||||
<parameter name="config_19_1" type="integer" groupName="configuration">
|
||||
<label>19: Alternate Exclusion</label>
|
||||
<description><![CDATA[
|
||||
When programmed, the default exclusion process will not exclude device from network. Prevents accidental removal of device from network<br /> <h1>Overview</h1><p><br /></p>
|
||||
Change from default exclusion setting. Prevents accidental removal of device from network<br /> <h1>Overview</h1><p><br /></p>
|
||||
]]></description>
|
||||
<default>0</default>
|
||||
<options>
|
||||
|
@ -118,21 +122,27 @@ When programmed, the default exclusion process will not exclude device from netw
|
|||
|
||||
<parameter name="config_30_1" type="integer" groupName="configuration">
|
||||
<label>30: Minimum Dim Threshold</label>
|
||||
<description>User can custom set a Minimum dim level other than 1%. To be used when performance in lower range is not suitable in normal operation</description>
|
||||
<description><![CDATA[
|
||||
Set custom minimum dimmer threshold<br /> <h1>Overview</h1><p><br /></p>
|
||||
]]></description>
|
||||
<default>1</default>
|
||||
<limitToOptions>false</limitToOptions>
|
||||
</parameter>
|
||||
|
||||
<parameter name="config_31_1" type="integer" groupName="configuration">
|
||||
<label>31: Maximum Dim Threshold</label>
|
||||
<description>User can custom set a Maximum dim level other than 99%. To be used when performance in upper range is not suitable in normal operation</description>
|
||||
<description><![CDATA[
|
||||
Set custom maximum dimmer threshold<br /> <h1>Overview</h1><p><br /></p>
|
||||
]]></description>
|
||||
<default>99</default>
|
||||
<limitToOptions>false</limitToOptions>
|
||||
</parameter>
|
||||
|
||||
<parameter name="config_32_1" type="integer" groupName="configuration">
|
||||
<label>32: Brightness Level</label>
|
||||
<description>Dimmer will turn ON to this level</description>
|
||||
<label>32: Default Brightness Level</label>
|
||||
<description><![CDATA[
|
||||
Dimmer will turn ON to this level<br /> <h1>Overview</h1><p><br /></p>
|
||||
]]></description>
|
||||
<default>99</default>
|
||||
<limitToOptions>false</limitToOptions>
|
||||
</parameter>
|
||||
|
@ -158,6 +168,18 @@ Z-Wave Plus Lifeline<br /> <h1>Overview</h1><p><br /></p>
|
|||
<multipleLimit>5</multipleLimit>
|
||||
</parameter>
|
||||
|
||||
<parameter name="group_2" type="text" groupName="association" multiple="true">
|
||||
<label>2: Single Press</label>
|
||||
<description>Basic Set - Controlled with the local load</description>
|
||||
<multipleLimit>5</multipleLimit>
|
||||
</parameter>
|
||||
|
||||
<parameter name="group_3" type="text" groupName="association" multiple="true">
|
||||
<label>3: Double Tap</label>
|
||||
<description>Basic Set - Controlled by pressing On/Off</description>
|
||||
<multipleLimit>5</multipleLimit>
|
||||
</parameter>
|
||||
|
||||
<!-- STATIC DEFINITIONS -->
|
||||
<parameter name="node_id" type="integer" min="1" max="232" readOnly="true" required="true">
|
||||
<label>Node ID</label>
|
||||
|
|
|
@ -8,7 +8,7 @@
|
|||
<thing-type id="inovelli_lzw31sn_00_000" listed="false">
|
||||
<label>LZW31-SN Red Series Dimmer</label>
|
||||
<description><![CDATA[
|
||||
Red Series Dimmer<br /> <h1>Overview</h1><p>Inovelli’s new dimmer switch is packed with features and designed with your house in mind.</p> <p>From a hardware side we’ve upgraded our Z-Wave module for better distance and there is no longer a need for a neutral wire. The switch can be used in any 3-Way or 4-Way setting and allows you to use either an auxiliary switch, a smart switch or your existing dumb switch (NOTE: this only applies if a neutral wire is installed. If there is no neutral wire, the switch only works in a single-pole setting).</p> <p>From a firmware side, this switch has it all (literally). Scene control, notifications (flashes if your garage door is left open) and can be configured on any HUB.</p> <br /> <h2>Inclusion Information</h2><p>Once the inclusion process has started press the config button 3X and the LED bar will flash blue. If the switch was included successfully the bar will turn GREEN, however if the switch was not included successfully it will turn RED.</p> <br /> <h2>Exclusion Information</h2><p>Press the Config Button 3X</p> <br /> <h2>Wakeup Information</h2><p><br /></p>
|
||||
Red Series Dimmer<br /> <h1>Overview</h1><p>Inovelli’s new dimmer switch is packed with features and designed with your house in mind.</p> <p>From a hardware side we’ve upgraded our Z-Wave module for better distance and there is no longer a need for a neutral wire. The switch can be used in any 3-Way or 4-Way setting and allows you to use either an auxiliary switch, a smart switch or your existing dumb switch (NOTE: this only applies if a neutral wire is installed. If there is no neutral wire, the switch only works in a single-pole setting).</p> <p>From a firmware side, this switch has it all (literally). Scene control, notifications (flashes if your garage door is left open) and can be configured on any HUB.</p><p>Updated instructions here: https://community.inovelli.com/t/resources-red-series-dimmer-switch-manual/7306#</p> <br /> <h2>Inclusion Information</h2><p>Once the inclusion process has started press the config button 3X and the LED bar will flash blue. If the switch was included successfully the bar will turn GREEN, however if the switch was not included successfully it will turn RED.</p> <br /> <h2>Exclusion Information</h2><p>Press the Config Button 3X</p> <br /> <h2>Wakeup Information</h2><p><br /></p>
|
||||
]]></description>
|
||||
<category>WallSwitch</category>
|
||||
|
||||
|
@ -309,12 +309,13 @@ Instant On<br /> <h1>Overview</h1><p>Enable instant on (ie: disable 700ms delay
|
|||
<parameter name="config_52_1" type="integer" groupName="configuration">
|
||||
<label>52: Enable "smart bulb" mode</label>
|
||||
<description><![CDATA[
|
||||
Enable "smart bulb" mode<br /> <h1>Overview</h1><p>Smart bulb mode: If set to 1, power will output maximum % when dimmer is on to optimize performance with smart bulbs.</p>
|
||||
Enable "smart bulb" mode<br /> <h1>Overview</h1><p>Switch Mode<br />0 = Normal operation</p><p>1 = On /Off Mode - Switch will either be in the state of 0% power or 99% power, not allowing dimming. Useful for non-dimmable bulbs or other unique loads</p><p>2 = Smart Bulb - Switch always at 99% for use with loads like an Inovelli Bulb or Philips Hue Bulb. Use in conjunction with associations or scenes to achieve an awesome smart bulb experience</p><p>Requires firmware 1.47 and above.</p>
|
||||
]]></description>
|
||||
<default>0</default>
|
||||
<options>
|
||||
<option value="0">normal bulb</option>
|
||||
<option value="1">smart bulb</option>
|
||||
<option value="1">On/Off Mode</option>
|
||||
<option value="2">Smart Bulb</option>
|
||||
</options>
|
||||
</parameter>
|
||||
|
||||
|
|
Loading…
Reference in New Issue