Updated external content (Jenkins build 640)
parent
72d4c28a8f
commit
8e5860b302
File diff suppressed because one or more lines are too long
|
@ -16,6 +16,21 @@ install: manual
|
|||
# JavaScript Scripting
|
||||
|
||||
This add-on provides support for JavaScript (ECMAScript 2021+) that can be used as a scripting language within automation rules.
|
||||
JavaScript scripts provide access to almost all the functionality in an openHAB runtime environment.
|
||||
|
||||
- [Creating JavaScript Scripts](#creating-javascript-scripts)
|
||||
- [Logging](#logging)
|
||||
- [Core Actions](#core-actions)
|
||||
- [itemRegistry](#itemregistry)
|
||||
- [Event Bus Actions](#event-bus-actions)
|
||||
- [Exec Actions](#exec-actions)
|
||||
- [HTTP Actions](#http-actions)
|
||||
- [Timers](#timers)
|
||||
- [Scripts Actions](#scripts-actions)
|
||||
- [Cloud Notification Actions](#cloud-notification-actions)
|
||||
- [Persistence Extensions](#persistence-extensions)
|
||||
- [Ephemeris Actions](#ephemeris-actions)
|
||||
- [Types and Units](#types-and-units)
|
||||
|
||||
## Creating JavaScript Scripts
|
||||
|
||||
|
@ -36,23 +51,215 @@ log:set DEBUG org.openhab.core.automation
|
|||
|
||||
For more information on the available APIs in scripts see the [JSR223 Scripting]({{base}}/configuration/jsr223.html) documentation.
|
||||
|
||||
## Script Examples
|
||||
The following examples show how to access common openHAB functionalities.
|
||||
|
||||
## Logging
|
||||
|
||||
JavaScript scripts provide access to almost all the functionality in an openHAB runtime environment.
|
||||
As a simple example, the following script logs "Hello, World!".
|
||||
Note that `console.log` will usually not work since the output has no terminal to display the text.
|
||||
__Please note:__ Support for `console.log` will likely be added together with a logging API in the [helper library](https://github.com/openhab/openhab-js).
|
||||
The openHAB server uses the [SLF4J](https://www.slf4j.org/) library for logging.
|
||||
|
||||
```js
|
||||
const LoggerFactory = Java.type('org.slf4j.LoggerFactory');
|
||||
|
||||
LoggerFactory.getLogger("org.openhab.core.automation.examples").info("Hello world!");
|
||||
```javascript
|
||||
let logger = Java.type('org.slf4j.LoggerFactory').getLogger('org.openhab.rule.' + this.ruleUID);
|
||||
logger.info('Hello world!');
|
||||
logger.warn('Successfully logged warning.');
|
||||
logger.error('Successfully logged error.');
|
||||
```
|
||||
|
||||
Depending on the openHAB logging configuration, you may need to prefix logger names with `org.openhab.core.automation` for them to show up in the log file (or you modify the logging configuration).
|
||||
|
||||
The script uses the [LoggerFactory](https://www.slf4j.org/apidocs/org/slf4j/Logger.html) to obtain a named logger and then logs a message like:
|
||||
|
||||
```text
|
||||
... [INFO ] [.openhab.core.automation.examples:-2 ] - Hello world!
|
||||
... [INFO ] [org.openhab.rule.<ruleId> ] - Hello world!
|
||||
... [WARN ] [org.openhab.rule.<ruleId> ] - Successfully logged warning.
|
||||
... [ERROR] [org.openhab.rule.<ruleId> ] - Successfully logged error.
|
||||
```
|
||||
|
||||
## Core Actions
|
||||
|
||||
The openHAB services, which are pre-included in the integrated JavaScript engine, must explicitely be imported.
|
||||
|
||||
__Please note:__ The [helper library](https://github.com/openhab/openhab-js) is on the way and will become the preferred API to work with openHAB.
|
||||
|
||||
```javascript
|
||||
let openhab = require('@runtime');
|
||||
```
|
||||
|
||||
### itemRegistry
|
||||
|
||||
```javascript
|
||||
let state = openhab.itemRegistry.getItem(itemName).getState();
|
||||
```
|
||||
|
||||
You can use `toString()` to convert an item's state to string or `toBigDecimal()` to convert to number.
|
||||
|
||||
### Event Bus Actions
|
||||
|
||||
```javascript
|
||||
openhab.events.sendCommand(itemName, command);
|
||||
openhab.events.postUpdate(itemName, state);
|
||||
```
|
||||
|
||||
`command` and `state` can be a string `'string'` or a number depending on the item.
|
||||
|
||||
### Exec Actions
|
||||
|
||||
Execute a command line.
|
||||
|
||||
```javascript
|
||||
openhab.Exec = Java.type('org.openhab.core.model.script.actions.Exec');
|
||||
let Duration = Java.type('java.time.Duration');
|
||||
|
||||
// Execute command line.
|
||||
openhab.Exec.executeCommandLine('echo', 'Hello World!');
|
||||
|
||||
// Execute command line with timeout.
|
||||
openhab.Exec.executeCommandLine(Duration.ofSeconds(20), 'echo', 'Hello World!');
|
||||
|
||||
// Get response from command line.
|
||||
let response = openhab.Exec.executeCommandLine('echo', 'Hello World!');
|
||||
|
||||
// Get response from command line with timeout.
|
||||
response = openhab.Exec.executeCommandLine(Duration.ofSeconds(20), 'echo', 'Hello World!');
|
||||
```
|
||||
|
||||
### HTTP Actions
|
||||
|
||||
For available actions have a look at the [HTTP Actions Docs](https://www.openhab.org/docs/configuration/actions.html#http-actions).
|
||||
|
||||
```javascript
|
||||
openhab.HTTP = Java.type('org.openhab.core.model.script.actions.HTTP');
|
||||
|
||||
// Example GET Request
|
||||
var response = openhab.HTTP.sendHttpGetRequest('<url>');
|
||||
```
|
||||
|
||||
Replace `<url>` with the request url.
|
||||
|
||||
### Timers
|
||||
|
||||
```javascript
|
||||
let ZonedDateTime = Java.type('java.time.ZonedDateTime');
|
||||
let now = ZonedDateTime.now();
|
||||
openhab.ScriptExecution = Java.type('org.openhab.core.model.script.actions.ScriptExecution');
|
||||
|
||||
// Function to run when the timer goes off.
|
||||
function timerOver () {
|
||||
logger.info('The timer is over.');
|
||||
}
|
||||
|
||||
// Create the Timer.
|
||||
this.myTimer = openhab.ScriptExecution.createTimer(now.plusSeconds(10), timerOver);
|
||||
|
||||
// Cancel the timer.
|
||||
this.myTimer.cancel();
|
||||
|
||||
// Check whether the timer is active. Returns true if the timer is active and will be executed as scheduled.
|
||||
let active = this.myTimer.isActive();
|
||||
|
||||
// Reschedule the timer.
|
||||
this.myTimer.reschedule(now.plusSeconds(5));
|
||||
```
|
||||
|
||||
### Scripts Actions
|
||||
|
||||
Call scripts created in the UI (Settings -> Scripts) with or without parameters.
|
||||
|
||||
```javascript
|
||||
openhab.scriptExtension = Java.type('org.openhab.core.automation.module.script.ScriptExtensionProvider');
|
||||
let bundleContext = Java.type('org.osgi.framework.FrameworkUtil').getBundle(openhab.scriptExtension.class).getBundleContext();
|
||||
openhab.RuleManager = bundleContext.getService(bundleContext.getServiceReference('org.openhab.core.automation.RuleManager'));
|
||||
|
||||
// Simple call.
|
||||
openhab.RuleManager.runNow('<scriptToRun>');
|
||||
|
||||
// Advanced call with arguments.
|
||||
let map = new java.util.HashMap();
|
||||
map.put('identifier1', 'value1');
|
||||
map.put('identifier2', 'value2');
|
||||
// Second argument is whether to consider the conditions, third is a Map<String, Object> (a way to pass data).
|
||||
openhab.RuleManager.runNow('<scriptToRun>', true, map);
|
||||
```
|
||||
|
||||
Replace `<scriptToRun>` with your script's (unique-)id.
|
||||
|
||||
## Cloud Notification Actions
|
||||
|
||||
Notification actions may be placed in Rules to send alerts to mobile devices registered with an [openHAB Cloud instance](https://github.com/openhab/openhab-cloud) such as [myopenHAB.org](https://myopenhab.org/).
|
||||
|
||||
For available actions have a look at the [Cloud Notification Actions Docs](https://www.openhab.org/docs/configuration/actions.html#cloud-notification-actions).
|
||||
|
||||
```javascript
|
||||
openhab.NotificationAction = Java.type('org.openhab.io.openhabcloud.NotificationAction')
|
||||
|
||||
// Example
|
||||
openhab.NotificationAction.sendNotification('<email>', '<message>'); // to a single myopenHAB user identified by e-mail
|
||||
openhab.NotificationAction.sendBroadcastNotification('<message>'); // to all myopenHAB users
|
||||
```
|
||||
|
||||
Replace `<email>` with the e-mail address of the user.
|
||||
Replace `<message>` with the notification text.
|
||||
|
||||
## Persistence Extensions
|
||||
|
||||
For available commands have a look at [Persistence Extensions in Scripts ans Rules](https://www.openhab.org/docs/configuration/persistence.html#persistence-extensions-in-scripts-and-rules).
|
||||
|
||||
For deeper information have a look at the [Persistence Extensions JavaDoc](https://www.openhab.org/javadoc/latest/org/openhab/core/persistence/extensions/persistenceextensions).
|
||||
|
||||
```javascript
|
||||
openhab.PersistenceExtensions = Java.type('org.openhab.core.persistence.extensions.PersistenceExtensions');
|
||||
let ZonedDateTime = Java.type('java.time.ZonedDateTime');
|
||||
let now = ZonedDateTime.now();
|
||||
|
||||
// Example
|
||||
var avg = openhab.PersistenceExtensions.averageSince(itemRegistry.getItem('<item>'), now.minusMinutes(5), "influxdb");
|
||||
```
|
||||
|
||||
Replace `<persistence>` with the persistence service to use.
|
||||
Replace `<item>` with the itemname.
|
||||
|
||||
## Ephemeris Actions
|
||||
|
||||
Ephemeris is a way to determine what type of day today or a number of days before or after today is. For example, a way to determine if today is a weekend, a bank holiday, someone’s birthday, trash day, etc.
|
||||
|
||||
For available actions, have a look at the [Ephemeris Actions Docs](https://www.openhab.org/docs/configuration/actions.html#ephemeris).
|
||||
|
||||
For deeper information have a look at the [Ephemeris JavaDoc](https://www.openhab.org/javadoc/latest/org/openhab/core/model/script/actions/ephemeris).
|
||||
|
||||
```javascript
|
||||
openhab.Ephemeris = Java.type('org.openhab.core.model.script.actions.Ephemeris');
|
||||
|
||||
// Example
|
||||
let weekend = openhab.Ephemeris.isWeekend();
|
||||
```
|
||||
|
||||
## Types and Units
|
||||
|
||||
Import types from openHAB Core for type conversion and more.
|
||||
Import Units from openHAB Core for unit conversion and more.
|
||||
|
||||
```javascript
|
||||
openhab.typeOrUnit = Java.type('org.openhab.core.library.types.typeOrUnit');
|
||||
|
||||
// Example
|
||||
openhab.HSBType = Java.type('org.openhab.core.library.types.HSBType');
|
||||
let hsb = openhab.HSBType.fromRGB(4, 6, 9);
|
||||
```
|
||||
|
||||
Available types are:
|
||||
* `QuantityType`
|
||||
* `StringListType`
|
||||
* `RawType`
|
||||
* `DateTimeType`
|
||||
* `DecimalType`
|
||||
* `HSBType`
|
||||
* `PercentType`
|
||||
* `PointType`
|
||||
* `StringType`
|
||||
|
||||
Available untis are:
|
||||
* `SIUnits`
|
||||
* `ImperialUnits`
|
||||
* `MetricPrefix`
|
||||
* `Units`
|
||||
* `BinaryPrefix`
|
||||
|
|
|
@ -0,0 +1,246 @@
|
|||
---
|
||||
id: anel
|
||||
label: Anel NET-PwrCtrl
|
||||
title: Anel NET-PwrCtrl - Bindings
|
||||
type: binding
|
||||
description: "Monitor and control Anel NET-PwrCtrl devices."
|
||||
since: 3x
|
||||
logo: images/addons/anel.png
|
||||
install: manual
|
||||
---
|
||||
|
||||
<!-- Attention authors: Do not edit directly. Please add your changes to the appropriate source repository -->
|
||||
|
||||
{% include base.html %}
|
||||
|
||||
# Anel NET-PwrCtrl Binding
|
||||
|
||||
Monitor and control Anel NET-PwrCtrl devices.
|
||||
|
||||
NET-PwrCtrl devices are power sockets / relays that can be configured via browser but they can also be controlled over the network, e.g. with an Android or iPhone app - and also with openHAB via this binding.
|
||||
Some NET-PwrCtrl devices also have 8 I/O ports which can either be used to directly switch the sockets / relays, or they can be used as general input / output switches in openHAB.
|
||||
|
||||
|
||||
## Supported Things
|
||||
|
||||
There are three kinds of devices ([overview on manufacturer's homepage](https://en.anel.eu/?src=/produkte/produkte.htm)):
|
||||
|
||||
| [Anel NET-PwrCtrl HUT](https://en.anel.eu/?src=/produkte/hut_2/hut_2.htm) <br/> <sub>( _advanced-firmware_ )</sub> | [Anel NET-PwrCtrl IO](https://en.anel.eu/?src=/produkte/io/io.htm) <br/> <sub>( _advanced-firmware_ )</sub> | [Anel NET-PwrCtrl HOME](https://de.anel.eu/?src=produkte/home/home.htm) <br/> <sub>( _home_ )</sub> <br/> (only German version) |
|
||||
| --- | --- | --- |
|
||||
| [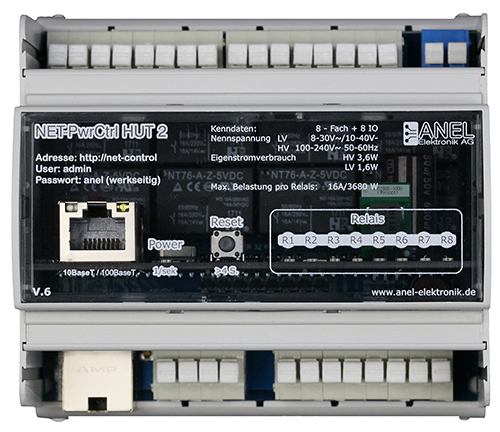](https://de.anel.eu/?src=produkte/hut_2/hut_2.htm) | [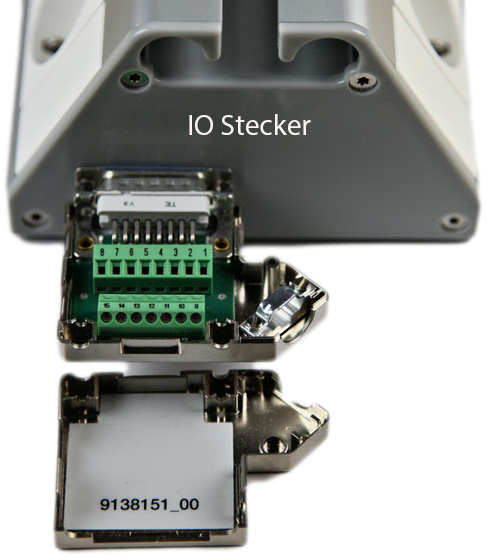](https://de.anel.eu/?src=produkte/io/io.htm) | [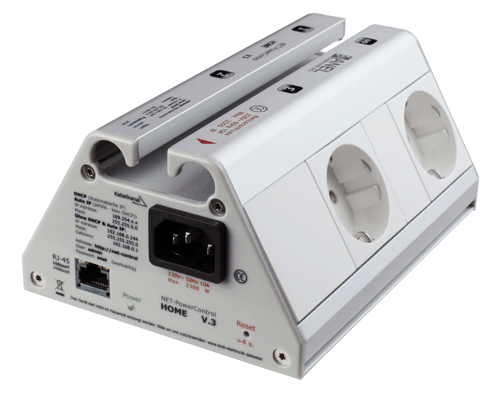](https://de.anel.eu/?src=produkte/home/home.htm) |
|
||||
|
||||
Thing type IDs:
|
||||
|
||||
* *home*: The smallest device, the _HOME_, is the only one with only three power sockets and only available in Germany.
|
||||
* *simple-firmware*: The _PRO_ and _REDUNDANT_ have eight power sockets and a similar (simplified) firmware as the _HOME_.
|
||||
* *advanced-firmware*: All others (_ADV_, _IO_, and the different _HUT_ variants) have eight power sockets / relays, eight IO ports, and an advanced firmware.
|
||||
|
||||
An [additional sensor](https://en.anel.eu/?src=/produkte/sensor_1/sensor_1.htm) may be used for monitoring temperature, humidity, and brightness.
|
||||
The sensor can be attached to a _HUT_ device via an Ethernet cable (max length is 50m).
|
||||
|
||||
|
||||
## Discovery
|
||||
|
||||
Devices can be discovered automatically if their UDP ports are configured as follows:
|
||||
|
||||
* 75 / 77 (default)
|
||||
* 750 / 770
|
||||
* 7500 / 7700
|
||||
* 7750 / 7770
|
||||
|
||||
If a device is found for a specific port (excluding the default port), the subsequent port is also scanned, e.g. 7500/7700 → 7501/7701 → 7502/7702 → etc.
|
||||
|
||||
Depending on the network switch and router devices, discovery may or may not work on wireless networks.
|
||||
It should work reliably though on local wired networks.
|
||||
|
||||
|
||||
## Thing Configuration
|
||||
|
||||
Each Thing requires the following configuration parameters.
|
||||
|
||||
| Parameter | Type | Default | Required | Description |
|
||||
|-----------------------|---------|-------------|----------|-------------|
|
||||
| Hostname / IP address | String | net-control | yes | Hostname or IP address of the device |
|
||||
| Send Port | Integer | 75 | yes | UDP port to send data to the device (in the anel web UI, it's the receive port!) |
|
||||
| Receive Port | Integer | 77 | yes | UDP port to receive data from the device (in the anel web UI, it's the send port!) |
|
||||
| User | String | user7 | yes | User to access the device (make sure it has rights to change relay / IO states!) |
|
||||
| Password | String | anel | yes | Password of the given user |
|
||||
|
||||
For multiple devices, please use exclusive UDP ports for each device.
|
||||
Ports above 1024 are recommended because they are outside the range of system ports.
|
||||
|
||||
Possible entries in your thing file could be (thing types _home_, _simple-firmware_, and _advanced-firmware_ are explained above in _Supported Things_):
|
||||
|
||||
```
|
||||
anel:home:mydevice1 [hostname="192.168.0.101", udpSendPort=7500, udpReceivePort=7700, user="user7", password="anel"]
|
||||
anel:simple-firmware:mydevice2 [hostname="192.168.0.102", udpSendPort=7501, udpReceivePort=7701, user="user7", password="anel"]
|
||||
anel:advanced-firmware:mydevice3 [hostname="192.168.0.103", udpSendPort=7502, udpReceivePort=7702, user="user7", password="anel"]
|
||||
anel:advanced-firmware:mydevice4 [hostname="192.168.0.104", udpSendPort=7503, udpReceivePort=7703, user="user7", password="anel"]
|
||||
```
|
||||
|
||||
|
||||
## Channels
|
||||
|
||||
Depending on the thing type, the following channels are available.
|
||||
|
||||
| Channel ID | Item Type | Supported Things | Read Only | Description |
|
||||
|--------------------|--------------------|-------------------|-----------|-------------|
|
||||
| prop#name | String | all | yes | Name of the device |
|
||||
| prop#temperature | Number:Temperature | simple / advanced | yes | Temperature of the integrated sensor |
|
||||
| sensor#temperature | Number:Temperature | advanced | yes | Temperature of the optional external sensor |
|
||||
| sensor#humidity | Number | advanced | yes | Humidity of the optional external sensor |
|
||||
| sensor#brightness | Number | advanced | yes | Brightness of the optional external sensor |
|
||||
| r1#name | String | all | yes | Name of relay / socket 1 |
|
||||
| r2#name | String | all | yes | Name of relay / socket 2 |
|
||||
| r3#name | String | all | yes | Name of relay / socket 3 |
|
||||
| r4#name | String | simple / advanced | yes | Name of relay / socket 4 |
|
||||
| r5#name | String | simple / advanced | yes | Name of relay / socket 5 |
|
||||
| r6#name | String | simple / advanced | yes | Name of relay / socket 6 |
|
||||
| r7#name | String | simple / advanced | yes | Name of relay / socket 7 |
|
||||
| r8#name | String | simple / advanced | yes | Name of relay / socket 8 |
|
||||
| r1#state | Switch | all | no * | State of relay / socket 1 |
|
||||
| r2#state | Switch | all | no * | State of relay / socket 2 |
|
||||
| r3#state | Switch | all | no * | State of relay / socket 3 |
|
||||
| r4#state | Switch | simple / advanced | no * | State of relay / socket 4 |
|
||||
| r5#state | Switch | simple / advanced | no * | State of relay / socket 5 |
|
||||
| r6#state | Switch | simple / advanced | no * | State of relay / socket 6 |
|
||||
| r7#state | Switch | simple / advanced | no * | State of relay / socket 7 |
|
||||
| r8#state | Switch | simple / advanced | no * | State of relay / socket 8 |
|
||||
| r1#locked | Switch | all | yes | Whether or not relay / socket 1 is locked |
|
||||
| r2#locked | Switch | all | yes | Whether or not relay / socket 2 is locked |
|
||||
| r3#locked | Switch | all | yes | Whether or not relay / socket 3 is locked |
|
||||
| r4#locked | Switch | simple / advanced | yes | Whether or not relay / socket 4 is locked |
|
||||
| r5#locked | Switch | simple / advanced | yes | Whether or not relay / socket 5 is locked |
|
||||
| r6#locked | Switch | simple / advanced | yes | Whether or not relay / socket 6 is locked |
|
||||
| r7#locked | Switch | simple / advanced | yes | Whether or not relay / socket 7 is locked |
|
||||
| r8#locked | Switch | simple / advanced | yes | Whether or not relay / socket 8 is locked |
|
||||
| io1#name | String | advanced | yes | Name of IO port 1 |
|
||||
| io2#name | String | advanced | yes | Name of IO port 2 |
|
||||
| io3#name | String | advanced | yes | Name of IO port 3 |
|
||||
| io4#name | String | advanced | yes | Name of IO port 4 |
|
||||
| io5#name | String | advanced | yes | Name of IO port 5 |
|
||||
| io6#name | String | advanced | yes | Name of IO port 6 |
|
||||
| io7#name | String | advanced | yes | Name of IO port 7 |
|
||||
| io8#name | String | advanced | yes | Name of IO port 8 |
|
||||
| io1#state | Switch | advanced | no ** | State of IO port 1 |
|
||||
| io2#state | Switch | advanced | no ** | State of IO port 2 |
|
||||
| io3#state | Switch | advanced | no ** | State of IO port 3 |
|
||||
| io4#state | Switch | advanced | no ** | State of IO port 4 |
|
||||
| io5#state | Switch | advanced | no ** | State of IO port 5 |
|
||||
| io6#state | Switch | advanced | no ** | State of IO port 6 |
|
||||
| io7#state | Switch | advanced | no ** | State of IO port 7 |
|
||||
| io8#state | Switch | advanced | no ** | State of IO port 8 |
|
||||
| io1#mode | Switch | advanced | yes | Mode of port 1: _ON_ = input, _OFF_ = output |
|
||||
| io2#mode | Switch | advanced | yes | Mode of port 2: _ON_ = input, _OFF_ = output |
|
||||
| io3#mode | Switch | advanced | yes | Mode of port 3: _ON_ = input, _OFF_ = output |
|
||||
| io4#mode | Switch | advanced | yes | Mode of port 4: _ON_ = input, _OFF_ = output |
|
||||
| io5#mode | Switch | advanced | yes | Mode of port 5: _ON_ = input, _OFF_ = output |
|
||||
| io6#mode | Switch | advanced | yes | Mode of port 6: _ON_ = input, _OFF_ = output |
|
||||
| io7#mode | Switch | advanced | yes | Mode of port 7: _ON_ = input, _OFF_ = output |
|
||||
| io8#mode | Switch | advanced | yes | Mode of port 8: _ON_ = input, _OFF_ = output |
|
||||
|
||||
\* Relay / socket state is read-only if it is locked; otherwise it is changeable.<br/>
|
||||
\** IO port state is read-only if its mode is _input_, it is changeable if its mode is _output_.
|
||||
|
||||
|
||||
## Full Example
|
||||
|
||||
`.things` file:
|
||||
|
||||
```
|
||||
Thing anel:advanced-firmware:anel1 "Anel1" [hostname="192.168.0.100", udpSendPort=7500, udpReceivePort=7700, user="user7", password="anel"]
|
||||
```
|
||||
|
||||
`.items` file:
|
||||
|
||||
```
|
||||
// device properties
|
||||
String anel1name "Anel1 Name" {channel="anel:advanced-firmware:anel1:prop#name"}
|
||||
Number:Temperature anel1temperature "Anel1 Temperature" {channel="anel:advanced-firmware:anel1:prop#temperature"}
|
||||
|
||||
// external sensor properties
|
||||
Number:Temperature anel1sensorTemperature "Anel1 Sensor Temperature" {channel="anel:advanced-firmware:anel1:sensor#temperature"}
|
||||
Number anel1sensorHumidity "Anel1 Sensor Humidity" {channel="anel:advanced-firmware:anel1:sensor#humidity"}
|
||||
Number anel1sensorBrightness "Anel1 Sensor Brightness" {channel="anel:advanced-firmware:anel1:sensor#brightness"}
|
||||
|
||||
// relay names and states
|
||||
String anel1relay1name "Anel1 Relay1 name" {channel="anel:advanced-firmware:anel1:r1#name"}
|
||||
Switch anel1relay1locked "Anel1 Relay1 locked" {channel="anel:advanced-firmware:anel1:r1#locked"}
|
||||
Switch anel1relay1state "Anel1 Relay1" {channel="anel:advanced-firmware:anel1:r1#state"}
|
||||
Switch anel1relay2state "Anel1 Relay2" {channel="anel:advanced-firmware:anel1:r2#state"}
|
||||
Switch anel1relay3state "Anel1 Relay3" {channel="anel:advanced-firmware:anel1:r3#state"}
|
||||
Switch anel1relay4state "Anel1 Relay4" {channel="anel:advanced-firmware:anel1:r4#state"}
|
||||
Switch anel1relay5state "Light Bedroom" {channel="anel:advanced-firmware:anel1:r5#state"}
|
||||
Switch anel1relay6state "Doorbell" {channel="anel:advanced-firmware:anel1:r6#state"}
|
||||
Switch anel1relay7state "Socket TV" {channel="anel:advanced-firmware:anel1:r7#state"}
|
||||
Switch anel1relay8state "Socket Terrace" {channel="anel:advanced-firmware:anel1:r8#state"}
|
||||
|
||||
// IO port names and states
|
||||
String anel1io1name "Anel1 IO1 name" {channel="anel:advanced-firmware:anel1:io1#name"}
|
||||
Switch anel1io1mode "Anel1 IO1 mode" {channel="anel:advanced-firmware:anel1:io1#mode"}
|
||||
Switch anel1io1state "Anel1 IO1" {channel="anel:advanced-firmware:anel1:io1#state"}
|
||||
Switch anel1io2state "Anel1 IO2" {channel="anel:advanced-firmware:anel1:io2#state"}
|
||||
Switch anel1io3state "Anel1 IO3" {channel="anel:advanced-firmware:anel1:io3#state"}
|
||||
Switch anel1io4state "Anel1 IO4" {channel="anel:advanced-firmware:anel1:io4#state"}
|
||||
Switch anel1io5state "Switch Bedroom" {channel="anel:advanced-firmware:anel1:io5#state"}
|
||||
Switch anel1io6state "Doorbell" {channel="anel:advanced-firmware:anel1:io6#state"}
|
||||
Switch anel1io7state "Switch Office" {channel="anel:advanced-firmware:anel1:io7#state"}
|
||||
Switch anel1io8state "Reed Contact Door" {channel="anel:advanced-firmware:anel1:io8#state"}
|
||||
```
|
||||
|
||||
`.sitemap` file:
|
||||
|
||||
```
|
||||
sitemap anel label="Anel NET-PwrCtrl" {
|
||||
Frame label="Device and Sensor" {
|
||||
Text item=anel1name label="Anel1 Name"
|
||||
Text item=anel1temperature label="Anel1 Temperature [%.1f °C]"
|
||||
Text item=anel1sensorTemperature label="Anel1 Sensor Temperature [%.1f °C]"
|
||||
Text item=anel1sensorHumidity label="Anel1 Sensor Humidity [%.1f]"
|
||||
Text item=anel1sensorBrightness label="Anel1 Sensor Brightness [%.1f]"
|
||||
}
|
||||
Frame label="Relays" {
|
||||
Text item=anel1relay1name label="Relay 1 name" labelcolor=[anel1relay1locked==ON="green",anel1relay1locked==OFF="maroon"]
|
||||
Switch item=anel1relay1state
|
||||
Switch item=anel1relay2state
|
||||
Switch item=anel1relay3state
|
||||
Switch item=anel1relay4state
|
||||
Switch item=anel1relay5state
|
||||
Switch item=anel1relay6state
|
||||
Switch item=anel1relay7state
|
||||
Switch item=anel1relay8state
|
||||
}
|
||||
Frame label="IO Ports" {
|
||||
Text item=anel1io1name label="IO 1 name" labelcolor=[anel1io1mode==OFF="green",anel1io1mode==ON="maroon"]
|
||||
Switch item=anel1io1state
|
||||
Switch item=anel1io2state
|
||||
Switch item=anel1io3state
|
||||
Switch item=anel1io4state
|
||||
Switch item=anel1io5state
|
||||
Switch item=anel1io6state
|
||||
Switch item=anel1io7state
|
||||
Switch item=anel1io8state
|
||||
}
|
||||
}
|
||||
```
|
||||
|
||||
The relay / IO port names are rarely useful because you probably set similar (static) labels for the state items.<br/>
|
||||
The locked state / IO mode is also rarely relevant in practice, because it typically doesn't change.
|
||||
|
||||
`.rules` file:
|
||||
|
||||
```
|
||||
rule "doorbell only at daytime"
|
||||
when Item anel1io6state changed then
|
||||
if (now.getHoursOfDay >= 6 && now.getHoursOfDay <= 22) {
|
||||
anel1relay6state.sendCommand(if (anel1io6state.state != ON) ON else OFF)
|
||||
}
|
||||
someNotificationItem.sendCommand("Someone just rang the doorbell")
|
||||
end
|
||||
```
|
||||
|
||||
|
||||
## Reference Documentation
|
||||
|
||||
The UDP protocol of Anel devices is explained [here](https://forum.anel.eu/viewtopic.php?f=16&t=207).
|
||||
|
|
@ -143,15 +143,15 @@ Channels available for each appliance type are listed below.
|
|||
|
||||
| Program | Description |
|
||||
|---------|-------------------------------------|
|
||||
| 26 | Pots & Pans |
|
||||
| 27 | Clean Machine |
|
||||
| 28 | Economy |
|
||||
| 26 | Intensive |
|
||||
| 27 | Maintenance programme |
|
||||
| 28 | ECO |
|
||||
| 30 | Normal |
|
||||
| 32 | Sensor Wash |
|
||||
| 34 | Energy Saver |
|
||||
| 35 | China & Crystal |
|
||||
| 32 | Automatic |
|
||||
| 34 | SolarSave |
|
||||
| 35 | Gentle |
|
||||
| 36 | Extra Quiet |
|
||||
| 37 | SaniWash |
|
||||
| 37 | Hygiene |
|
||||
| 38 | QuickPowerWash |
|
||||
| 42 | Tall items |
|
||||
|
||||
|
@ -297,6 +297,7 @@ See oven.
|
|||
| Program | Description |
|
||||
|---------|-------------------------------------|
|
||||
| 10 | Automatic Plus |
|
||||
| 20 | Cottons |
|
||||
| 23 | Cottons hygiene |
|
||||
| 30 | Minimum iron |
|
||||
| 31 | Gentle minimum iron |
|
||||
|
@ -329,11 +330,11 @@ See oven.
|
|||
| 513 | 1 | Programme running |
|
||||
| 514 | 2 | Drying |
|
||||
| 515 | 3 | Drying Machine iron |
|
||||
| 516 | 4 | Drying Hand iron (1) |
|
||||
| 516 | 4 | Drying Hand iron (2) |
|
||||
| 517 | 5 | Drying Normal |
|
||||
| 518 | 6 | Drying Normal+ |
|
||||
| 519 | 7 | Cooling down |
|
||||
| 520 | 8 | Drying Hand iron (2) |
|
||||
| 520 | 8 | Drying Hand iron (1) |
|
||||
| 522 | 10 | Finished |
|
||||
|
||||
#### Washing Machine
|
||||
|
@ -353,7 +354,7 @@ See oven.
|
|||
| finish | DateTime | Read | Time to finish the program running on the appliance |
|
||||
| door | Contact | Read | Current state of the door of the appliance |
|
||||
| switch | Switch | Write | Switch the appliance on or off |
|
||||
| target | Number:Temperature | Read | Temperature of the selected program |
|
||||
| target | Number:Temperature | Read | Temperature of the selected program (10 °C = cold) |
|
||||
| spinningspeed | String | Read | Spinning speed in the program running on the appliance |
|
||||
| powerConsumption | Number:Power | Read | Power consumption by the currently running program on the appliance |
|
||||
| waterConsumption | Number:Volume | Read | Water consumption by the currently running program on the appliance |
|
||||
|
|
|
@ -195,14 +195,14 @@ Currently the miio binding supports more than 300 different models.
|
|||
| Device | ThingType | Device Model | Supported | Remark |
|
||||
|------------------------------|------------------|------------------------|-----------|------------|
|
||||
| AUX Smart Air Conditioner | miio:unsupported | aux.aircondition.v1 | No | |
|
||||
| Mi Air Frying Pan | miio:basic | [careli.fryer.maf01](#careli-fryer-maf01) | Yes | Identified manual actions for execution<br />`action{"did":"air-fryer-start-cook","siid":2,"aiid":1,"in":[]}`<br />`action{"did":"air-fryer-cancel-cooking","siid":2,"aiid":2,"in":[]}`<br />`action{"did":"air-fryer-pause","siid":2,"aiid":3,"in":[]}`<br />`action{"did":"custom-start-cook","siid":3,"aiid":1,"in":[1.0, 2.0, 3.0, 4.0, 5.0, 6.0, 7.0]}`<br />`action{"did":"custom-resume-cook","siid":3,"aiid":2,"in":[]}`<br />Please test and feedback if they are working to they can be linked to a channel.<br />Experimental support. Please report back if all channels are functional. Preferably share the debug log of property refresh and command responses |
|
||||
| Mi Smart Air Fryer (3.5L) | miio:basic | [careli.fryer.maf02](#careli-fryer-maf02) | Yes | Identified manual actions for execution<br />`action{"did":"air-fryer-start-cook","siid":2,"aiid":1,"in":[]}`<br />`action{"did":"air-fryer-cancel-cooking","siid":2,"aiid":2,"in":[]}`<br />`action{"did":"air-fryer-pause","siid":2,"aiid":3,"in":[]}`<br />`action{"did":"custom-start-custom-cook","siid":3,"aiid":1,"in":[1.0, 3.0, 4.0, 5.0, 6.0, 7.0]}`<br />`action{"did":"custom-resume-cooking","siid":3,"aiid":2,"in":[]}`<br />Please test and feedback if they are working to they can be linked to a channel.<br />Experimental support. Please report back if all channels are functional. Preferably share the debug log of property refresh and command responses |
|
||||
| Mi Air Frying Pan | miio:basic | [careli.fryer.maf03](#careli-fryer-maf03) | Yes | Identified manual actions for execution<br />`action{"did":"air-fryer-start-cook","siid":2,"aiid":1,"in":[]}`<br />`action{"did":"air-fryer-cancel-cooking","siid":2,"aiid":2,"in":[]}`<br />`action{"did":"air-fryer-pause","siid":2,"aiid":3,"in":[]}`<br />`action{"did":"custom-start-cook","siid":3,"aiid":1,"in":[1.0, 2.0, 3.0, 4.0, 5.0, 6.0, 7.0]}`<br />`action{"did":"custom-resume-cook","siid":3,"aiid":2,"in":[]}`<br />Please test and feedback if they are working to they can be linked to a channel.<br />Experimental support. Please report back if all channels are functional. Preferably share the debug log of property refresh and command responses |
|
||||
| Qingping Air Monitor Lite | miio:basic | [cgllc.airm.cgdn1](#cgllc-airm-cgdn1) | Yes | Identified manual actions for execution<br />`action{"did":"settings-set-start-time","siid":9,"aiid":2,"in":[2.0]}`<br />`action{"did":"settings-set-end-time","siid":9,"aiid":3,"in":[3.0]}`<br />`action{"did":"settings-set-frequency","siid":9,"aiid":4,"in":[4.0]}`<br />`action{"did":"settings-set-screen-off","siid":9,"aiid":5,"in":[5.0]}`<br />`action{"did":"settings-set-device-off","siid":9,"aiid":6,"in":[6.0]}`<br />`action{"did":"settings-set-temp-unit","siid":9,"aiid":7,"in":[7.0]}`<br />Please test and feedback if they are working to they can be linked to a channel. |
|
||||
| Mi Air Frying Pan | miio:basic | [careli.fryer.maf01](#careli-fryer-maf01) | Yes | Identified manual actions for execution<br />`action{"did":"air-fryer-start-cook","siid":2,"aiid":1,"in":[]}`<br />`action{"did":"air-fryer-cancel-cooking","siid":2,"aiid":2,"in":[]}`<br />`action{"did":"air-fryer-pause","siid":2,"aiid":3,"in":[]}`<br />`action{"did":"custom-start-cook","siid":3,"aiid":1,"in":[1.0, 2.0, 3.0, 4.0, 5.0, 6.0, 7.0]}`<br />`action{"did":"custom-resume-cook","siid":3,"aiid":2,"in":[]}`<br />Please test and feedback if they are working so they can be linked to a channel.<br />Experimental support. Please report back if all channels are functional. Preferably share the debug log of property refresh and command responses |
|
||||
| Mi Smart Air Fryer (3.5L) | miio:basic | [careli.fryer.maf02](#careli-fryer-maf02) | Yes | Identified manual actions for execution<br />`action{"did":"air-fryer-start-cook","siid":2,"aiid":1,"in":[]}`<br />`action{"did":"air-fryer-cancel-cooking","siid":2,"aiid":2,"in":[]}`<br />`action{"did":"air-fryer-pause","siid":2,"aiid":3,"in":[]}`<br />`action{"did":"custom-start-custom-cook","siid":3,"aiid":1,"in":[1.0, 3.0, 4.0, 5.0, 6.0, 7.0]}`<br />`action{"did":"custom-resume-cooking","siid":3,"aiid":2,"in":[]}`<br />Please test and feedback if they are working so they can be linked to a channel.<br />Experimental support. Please report back if all channels are functional. Preferably share the debug log of property refresh and command responses |
|
||||
| Mi Air Frying Pan | miio:basic | [careli.fryer.maf03](#careli-fryer-maf03) | Yes | Identified manual actions for execution<br />`action{"did":"air-fryer-start-cook","siid":2,"aiid":1,"in":[]}`<br />`action{"did":"air-fryer-cancel-cooking","siid":2,"aiid":2,"in":[]}`<br />`action{"did":"air-fryer-pause","siid":2,"aiid":3,"in":[]}`<br />`action{"did":"custom-start-cook","siid":3,"aiid":1,"in":[1.0, 2.0, 3.0, 4.0, 5.0, 6.0, 7.0]}`<br />`action{"did":"custom-resume-cook","siid":3,"aiid":2,"in":[]}`<br />Please test and feedback if they are working so they can be linked to a channel.<br />Experimental support. Please report back if all channels are functional. Preferably share the debug log of property refresh and command responses |
|
||||
| Qingping Air Monitor Lite | miio:basic | [cgllc.airm.cgdn1](#cgllc-airm-cgdn1) | Yes | Identified manual actions for execution<br />`action{"did":"settings-set-start-time","siid":9,"aiid":2,"in":[2.0]}`<br />`action{"did":"settings-set-end-time","siid":9,"aiid":3,"in":[3.0]}`<br />`action{"did":"settings-set-frequency","siid":9,"aiid":4,"in":[4.0]}`<br />`action{"did":"settings-set-screen-off","siid":9,"aiid":5,"in":[5.0]}`<br />`action{"did":"settings-set-device-off","siid":9,"aiid":6,"in":[6.0]}`<br />`action{"did":"settings-set-temp-unit","siid":9,"aiid":7,"in":[7.0]}`<br />Please test and feedback if they are working so they can be linked to a channel. |
|
||||
| Mi Multifunction Air Monitor | miio:basic | [cgllc.airmonitor.b1](#cgllc-airmonitor-b1) | Yes | |
|
||||
| Qingping Air Monitor | miio:basic | [cgllc.airmonitor.s1](#cgllc-airmonitor-s1) | Yes | |
|
||||
| Mi Universal Remote | miio:unsupported | chuangmi.ir.v2 | No | |
|
||||
| Mi Smart Power Plug 2 (Wi-Fi and Bluetooth Gateway) | miio:basic | [chuangmi.plug.212a01](#chuangmi-plug-212a01) | Yes | Experimental support. Please report back if all channels are functional. Preferably share the debug log of property refresh and command responses |
|
||||
| Mi Smart Power Plug 2 (Wi-Fi and Bluetooth Gateway) | miio:basic | [chuangmi.plug.212a01](#chuangmi-plug-212a01) | Yes | |
|
||||
| Mi Smart Plug WiFi | miio:basic | [chuangmi.plug.hmi205](#chuangmi-plug-hmi205) | Yes | |
|
||||
| Mi Smart Plug (WiFi) | miio:basic | [chuangmi.plug.hmi206](#chuangmi-plug-hmi206) | Yes | |
|
||||
| Mi Smart Wi-Fi Plug (Bluetooth Gateway) | miio:basic | [chuangmi.plug.hmi208](#chuangmi-plug-hmi208) | Yes | |
|
||||
|
@ -224,19 +224,19 @@ Currently the miio binding supports more than 300 different models.
|
|||
| Mi Smart Humidifier | miio:basic | [deerma.humidifier.mjjsq](#deerma-humidifier-mjjsq) | Yes | |
|
||||
| Mi Fresh Air Ventilator A1-150 | miio:basic | [dmaker.airfresh.a1](#dmaker-airfresh-a1) | Yes | |
|
||||
| Mi Fresh Air Ventilator | miio:basic | [dmaker.airfresh.t2017](#dmaker-airfresh-t2017) | Yes | |
|
||||
| Mi Smart Standing Fan 2 Lite | miio:basic | [dmaker.fan.1c](#dmaker-fan-1c) | Yes | Identified manual actions for execution<br />`action{"did":"fan-toggle","siid":2,"aiid":1,"in":[]}`<br />Please test and feedback if they are working to they can be linked to a channel.<br />Experimental support. Please report back if all channels are functional. Preferably share the debug log of property refresh and command responses |
|
||||
| Mi Smart Standing Fan 2 Lite | miio:basic | [dmaker.fan.1c](#dmaker-fan-1c) | Yes | Identified manual actions for execution<br />`action{"did":"fan-toggle","siid":2,"aiid":1,"in":[]}`<br />Please test and feedback if they are working so they can be linked to a channel.<br />Experimental support. Please report back if all channels are functional. Preferably share the debug log of property refresh and command responses |
|
||||
| Mi Smart Standing Fan 1X | miio:basic | [dmaker.fan.p5](#dmaker-fan-p5) | Yes | |
|
||||
| Mi Smart Standing Fan 1C | miio:basic | [dmaker.fan.p8](#dmaker-fan-p8) | Yes | Identified manual actions for execution<br />`action{"did":"fan-toggle","siid":2,"aiid":1,"in":[]}`<br />Please test and feedback if they are working to they can be linked to a channel.<br />Experimental support. Please report back if all channels are functional. Preferably share the debug log of property refresh and command responses |
|
||||
| Mi Smart Standing Fan 1C | miio:basic | [dmaker.fan.p8](#dmaker-fan-p8) | Yes | Identified manual actions for execution<br />`action{"did":"fan-toggle","siid":2,"aiid":1,"in":[]}`<br />Please test and feedback if they are working so they can be linked to a channel.<br />Experimental support. Please report back if all channels are functional. Preferably share the debug log of property refresh and command responses |
|
||||
| Mi Smart Tower Fan | miio:basic | [dmaker.fan.p9](#dmaker-fan-p9) | Yes | |
|
||||
| Mi Smart Standing Fan 2 | miio:basic | [dmaker.fan.p10](#dmaker-fan-p10) | Yes | |
|
||||
| Mi Smart Standing Fan Pro | miio:basic | [dmaker.fan.p15](#dmaker-fan-p15) | Yes | Identified manual actions for execution<br />`action{"did":"off-delay-time-toggle","siid":3,"aiid":1,"in":[]}`<br />Please test and feedback if they are working to they can be linked to a channel. |
|
||||
| Mi Smart Standing Fan 2 | miio:basic | [dmaker.fan.p18](#dmaker-fan-p18) | Yes | Identified manual actions for execution<br />`action{"did":"fan-toggle","siid":2,"aiid":1,"in":[]}`<br />Please test and feedback if they are working to they can be linked to a channel.<br />Experimental support. Please report back if all channels are functional. Preferably share the debug log of property refresh and command responses |
|
||||
| Mi Robot Vacuum Mop 1C STYTJ01ZHM | miio:basic | [dreame.vacuum.mc1808](#dreame-vacuum-mc1808) | Yes | Identified manual actions for execution<br />`action{"did":"battery-start-charge","siid":2,"aiid":1,"in":[]}`<br />`action{"did":"vacuum-start-sweep","siid":3,"aiid":1,"in":[]}`<br />`action{"did":"vacuum-stop-sweeping","siid":3,"aiid":2,"in":[]}`<br />`action{"did":"brush-cleaner-reset-brush-life","siid":26,"aiid":1,"in":[]}`<br />`action{"did":"filter-reset-filter-life","siid":27,"aiid":1,"in":[]}`<br />`action{"did":"brush-cleaner-reset-brush-life","siid":28,"aiid":1,"in":[]}`<br />`action{"did":"clean-start-clean","siid":18,"aiid":1,"in":[]}`<br />`action{"did":"clean-stop-clean","siid":18,"aiid":2,"in":[]}`<br />`action{"did":"remote-start-remote","siid":21,"aiid":1,"in":[1.0, 2.0]}`<br />`action{"did":"remote-stop-remote","siid":21,"aiid":2,"in":[]}`<br />`action{"did":"remote-exit-remote","siid":21,"aiid":3,"in":[]}`<br />`action{"did":"map-map-req","siid":23,"aiid":1,"in":[2.0]}`<br />`action{"did":"audio-position","siid":24,"aiid":1,"in":[]}`<br />`action{"did":"audio-set-voice","siid":24,"aiid":2,"in":[]}`<br />`action{"did":"audio-play-sound","siid":24,"aiid":3,"in":[]}`<br />Please test and feedback if they are working to they can be linked to a channel. |
|
||||
| Dreame Robot Vacuum-Mop F9 | miio:basic | [dreame.vacuum.p2008](#dreame-vacuum-p2008) | Yes | Identified manual actions for execution<br />`action{"did":"vacuum-start-sweep","siid":2,"aiid":1,"in":[]}`<br />`action{"did":"vacuum-stop-sweeping","siid":2,"aiid":2,"in":[]}`<br />`action{"did":"battery-start-charge","siid":3,"aiid":1,"in":[]}`<br />`action{"did":"brush-cleaner-reset-brush-life","siid":9,"aiid":1,"in":[]}`<br />`action{"did":"brush-cleaner-reset-brush-life","siid":10,"aiid":1,"in":[]}`<br />`action{"did":"filter-reset-filter-life","siid":11,"aiid":1,"in":[]}`<br />`action{"did":"vacuum-extend-start-clean","siid":4,"aiid":1,"in":[10.0]}`<br />`action{"did":"vacuum-extend-stop-clean","siid":4,"aiid":2,"in":[]}`<br />`action{"did":"map-map-req","siid":6,"aiid":1,"in":[2.0]}`<br />`action{"did":"map-update-map","siid":6,"aiid":2,"in":[4.0]}`<br />`action{"did":"audio-position","siid":7,"aiid":1,"in":[]}`<br />`action{"did":"audio-play-sound","siid":7,"aiid":2,"in":[]}`<br />`action{"did":"time-delete-timer","siid":8,"aiid":1,"in":[3.0]}`<br />Please test and feedback if they are working to they can be linked to a channel.<br />Experimental support. Please report back if all channels are functional. Preferably share the debug log of property refresh and command responses |
|
||||
| Mi Smart Standing Fan Pro | miio:basic | [dmaker.fan.p15](#dmaker-fan-p15) | Yes | Identified manual actions for execution<br />`action{"did":"off-delay-time-toggle","siid":3,"aiid":1,"in":[]}`<br />Please test and feedback if they are working so they can be linked to a channel. |
|
||||
| Mi Smart Standing Fan 2 | miio:basic | [dmaker.fan.p18](#dmaker-fan-p18) | Yes | Identified manual actions for execution<br />`action{"did":"fan-toggle","siid":2,"aiid":1,"in":[]}`<br />Please test and feedback if they are working so they can be linked to a channel.<br />Experimental support. Please report back if all channels are functional. Preferably share the debug log of property refresh and command responses |
|
||||
| Mi Robot Vacuum Mop 1C STYTJ01ZHM | miio:basic | [dreame.vacuum.mc1808](#dreame-vacuum-mc1808) | Yes | Identified manual actions for execution<br />`action{"did":"battery-start-charge","siid":2,"aiid":1,"in":[]}`<br />`action{"did":"vacuum-start-sweep","siid":3,"aiid":1,"in":[]}`<br />`action{"did":"vacuum-stop-sweeping","siid":3,"aiid":2,"in":[]}`<br />`action{"did":"brush-cleaner-reset-brush-life","siid":26,"aiid":1,"in":[]}`<br />`action{"did":"filter-reset-filter-life","siid":27,"aiid":1,"in":[]}`<br />`action{"did":"brush-cleaner-reset-brush-life","siid":28,"aiid":1,"in":[]}`<br />`action{"did":"clean-start-clean","siid":18,"aiid":1,"in":[]}`<br />`action{"did":"clean-stop-clean","siid":18,"aiid":2,"in":[]}`<br />`action{"did":"remote-start-remote","siid":21,"aiid":1,"in":[1.0, 2.0]}`<br />`action{"did":"remote-stop-remote","siid":21,"aiid":2,"in":[]}`<br />`action{"did":"remote-exit-remote","siid":21,"aiid":3,"in":[]}`<br />`action{"did":"map-map-req","siid":23,"aiid":1,"in":[2.0]}`<br />`action{"did":"audio-position","siid":24,"aiid":1,"in":[]}`<br />`action{"did":"audio-set-voice","siid":24,"aiid":2,"in":[]}`<br />`action{"did":"audio-play-sound","siid":24,"aiid":3,"in":[]}`<br />Please test and feedback if they are working so they can be linked to a channel. |
|
||||
| Dreame Robot Vacuum-Mop F9 | miio:basic | [dreame.vacuum.p2008](#dreame-vacuum-p2008) | Yes | Identified manual actions for execution<br />`action{"did":"vacuum-start-sweep","siid":2,"aiid":1,"in":[]}`<br />`action{"did":"vacuum-stop-sweeping","siid":2,"aiid":2,"in":[]}`<br />`action{"did":"battery-start-charge","siid":3,"aiid":1,"in":[]}`<br />`action{"did":"brush-cleaner-reset-brush-life","siid":9,"aiid":1,"in":[]}`<br />`action{"did":"brush-cleaner-reset-brush-life","siid":10,"aiid":1,"in":[]}`<br />`action{"did":"filter-reset-filter-life","siid":11,"aiid":1,"in":[]}`<br />`action{"did":"vacuum-extend-start-clean","siid":4,"aiid":1,"in":[10.0]}`<br />`action{"did":"vacuum-extend-stop-clean","siid":4,"aiid":2,"in":[]}`<br />`action{"did":"map-map-req","siid":6,"aiid":1,"in":[2.0]}`<br />`action{"did":"map-update-map","siid":6,"aiid":2,"in":[4.0]}`<br />`action{"did":"audio-position","siid":7,"aiid":1,"in":[]}`<br />`action{"did":"audio-play-sound","siid":7,"aiid":2,"in":[]}`<br />`action{"did":"time-delete-timer","siid":8,"aiid":1,"in":[3.0]}`<br />Please test and feedback if they are working so they can be linked to a channel.<br />Experimental support. Please report back if all channels are functional. Preferably share the debug log of property refresh and command responses |
|
||||
| Dreame Robot Vacuum D9 | miio:basic | [dreame.vacuum.p2009](#dreame-vacuum-p2009) | Yes | Identified manual actions for execution not linked in the database >`action{"did":"vacuum-extend-start-clean","siid":4,"aiid":1,"in":[10.0]}`<br />`action{"did":"vacuum-extend-stop-clean","siid":4,"aiid":2,"in":[]}`<br />`action{"did":"map-map-req","siid":6,"aiid":1,"in":[2.0]}`<br />`action{"did":"map-update-map","siid":6,"aiid":2,"in":[4.0]}`<br />`action{"did":"audio-position","siid":7,"aiid":1,"in":[]}`<br />`action{"did":"audio-play-sound","siid":7,"aiid":2,"in":[]}`<br />`action{"did":"time-delete-timer","siid":8,"aiid":1,"in":[3.0]}`<br /> |
|
||||
| Trouver Robot LDS Vacuum-Mop Finder | miio:basic | [dreame.vacuum.p2036](#dreame-vacuum-p2036) | Yes | Identified manual actions for execution not linked in the database >`action{"did":"vacuum-extend-start-clean","siid":4,"aiid":1,"in":[10.0]}`<br />`action{"did":"vacuum-extend-stop-clean","siid":4,"aiid":2,"in":[]}`<br />`action{"did":"map-map-req","siid":6,"aiid":1,"in":[2.0]}`<br />`action{"did":"map-update-map","siid":6,"aiid":2,"in":[4.0]}`<br />`action{"did":"audio-position","siid":7,"aiid":1,"in":[]}`<br />`action{"did":"audio-play-sound","siid":7,"aiid":2,"in":[]}`<br />`action{"did":"time-delete-timer","siid":8,"aiid":1,"in":[3.0]}`<br /> |
|
||||
| Mi Robot Vacuum-Mop 2 Pro+ | miio:basic | [dreame.vacuum.p2041o](#dreame-vacuum-p2041o) | Yes | Identified manual actions for execution<br />`action{"did":"vacuum-start-sweep","siid":2,"aiid":1,"in":[]}`<br />`action{"did":"vacuum-stop-sweeping","siid":2,"aiid":2,"in":[]}`<br />`action{"did":"battery-start-charge","siid":3,"aiid":1,"in":[]}`<br />`action{"did":"brush-cleaner-reset-brush-life","siid":9,"aiid":1,"in":[]}`<br />`action{"did":"brush-cleaner-reset-brush-life","siid":10,"aiid":1,"in":[]}`<br />`action{"did":"filter-reset-filter-life","siid":11,"aiid":1,"in":[]}`<br />`action{"did":"vacuum-extend-start-clean","siid":4,"aiid":1,"in":[10.0]}`<br />`action{"did":"vacuum-extend-stop-clean","siid":4,"aiid":2,"in":[]}`<br />`action{"did":"map-map-req","siid":6,"aiid":1,"in":[2.0]}`<br />`action{"did":"map-update-map","siid":6,"aiid":2,"in":[4.0]}`<br />`action{"did":"audio-position","siid":7,"aiid":1,"in":[]}`<br />`action{"did":"audio-play-sound","siid":7,"aiid":2,"in":[]}`<br />`action{"did":"time-delete-timer","siid":8,"aiid":1,"in":[3.0]}`<br />Please test and feedback if they are working to they can be linked to a channel.<br />Experimental support. Please report back if all channels are functional. Preferably share the debug log of property refresh and command responses |
|
||||
| MOVA Z500 Robot Vacuum and Mop Cleaner | miio:basic | [dreame.vacuum.p2156o](#dreame-vacuum-p2156o) | Yes | Identified manual actions for execution<br />`action{"did":"vacuum-start-sweep","siid":2,"aiid":1,"in":[]}`<br />`action{"did":"vacuum-stop-sweeping","siid":2,"aiid":2,"in":[]}`<br />`action{"did":"battery-start-charge","siid":3,"aiid":1,"in":[]}`<br />`action{"did":"brush-cleaner-reset-brush-life","siid":9,"aiid":1,"in":[]}`<br />`action{"did":"brush-cleaner-reset-brush-life","siid":10,"aiid":1,"in":[]}`<br />`action{"did":"filter-reset-filter-life","siid":11,"aiid":1,"in":[]}`<br />`action{"did":"vacuum-extend-start-clean","siid":4,"aiid":1,"in":[10.0]}`<br />`action{"did":"vacuum-extend-stop-clean","siid":4,"aiid":2,"in":[]}`<br />`action{"did":"map-map-req","siid":6,"aiid":1,"in":[2.0]}`<br />`action{"did":"map-update-map","siid":6,"aiid":2,"in":[4.0]}`<br />`action{"did":"audio-position","siid":7,"aiid":1,"in":[]}`<br />`action{"did":"audio-play-sound","siid":7,"aiid":2,"in":[]}`<br />`action{"did":"time-delete-timer","siid":8,"aiid":1,"in":[3.0]}`<br />Please test and feedback if they are working to they can be linked to a channel.<br />Experimental support. Please report back if all channels are functional. Preferably share the debug log of property refresh and command responses |
|
||||
| Mi Robot Vacuum-Mop 2 Pro+ | miio:basic | [dreame.vacuum.p2041o](#dreame-vacuum-p2041o) | Yes | Identified manual actions for execution<br />`action{"did":"vacuum-start-sweep","siid":2,"aiid":1,"in":[]}`<br />`action{"did":"vacuum-stop-sweeping","siid":2,"aiid":2,"in":[]}`<br />`action{"did":"battery-start-charge","siid":3,"aiid":1,"in":[]}`<br />`action{"did":"brush-cleaner-reset-brush-life","siid":9,"aiid":1,"in":[]}`<br />`action{"did":"brush-cleaner-reset-brush-life","siid":10,"aiid":1,"in":[]}`<br />`action{"did":"filter-reset-filter-life","siid":11,"aiid":1,"in":[]}`<br />`action{"did":"vacuum-extend-start-clean","siid":4,"aiid":1,"in":[10.0]}`<br />`action{"did":"vacuum-extend-stop-clean","siid":4,"aiid":2,"in":[]}`<br />`action{"did":"map-map-req","siid":6,"aiid":1,"in":[2.0]}`<br />`action{"did":"map-update-map","siid":6,"aiid":2,"in":[4.0]}`<br />`action{"did":"audio-position","siid":7,"aiid":1,"in":[]}`<br />`action{"did":"audio-play-sound","siid":7,"aiid":2,"in":[]}`<br />`action{"did":"time-delete-timer","siid":8,"aiid":1,"in":[3.0]}`<br />Please test and feedback if they are working so they can be linked to a channel.<br />Experimental support. Please report back if all channels are functional. Preferably share the debug log of property refresh and command responses |
|
||||
| MOVA Z500 Robot Vacuum and Mop Cleaner | miio:basic | [dreame.vacuum.p2156o](#dreame-vacuum-p2156o) | Yes | Identified manual actions for execution<br />`action{"did":"vacuum-start-sweep","siid":2,"aiid":1,"in":[]}`<br />`action{"did":"vacuum-stop-sweeping","siid":2,"aiid":2,"in":[]}`<br />`action{"did":"battery-start-charge","siid":3,"aiid":1,"in":[]}`<br />`action{"did":"brush-cleaner-reset-brush-life","siid":9,"aiid":1,"in":[]}`<br />`action{"did":"brush-cleaner-reset-brush-life","siid":10,"aiid":1,"in":[]}`<br />`action{"did":"filter-reset-filter-life","siid":11,"aiid":1,"in":[]}`<br />`action{"did":"vacuum-extend-start-clean","siid":4,"aiid":1,"in":[10.0]}`<br />`action{"did":"vacuum-extend-stop-clean","siid":4,"aiid":2,"in":[]}`<br />`action{"did":"map-map-req","siid":6,"aiid":1,"in":[2.0]}`<br />`action{"did":"map-update-map","siid":6,"aiid":2,"in":[4.0]}`<br />`action{"did":"audio-position","siid":7,"aiid":1,"in":[]}`<br />`action{"did":"audio-play-sound","siid":7,"aiid":2,"in":[]}`<br />`action{"did":"time-delete-timer","siid":8,"aiid":1,"in":[3.0]}`<br />Please test and feedback if they are working so they can be linked to a channel.<br />Experimental support. Please report back if all channels are functional. Preferably share the debug log of property refresh and command responses |
|
||||
| MOVA L600 Robot Vacuum and Mop Cleaner | miio:basic | [dreame.vacuum.p2157](#dreame-vacuum-p2157) | Yes | Identified manual actions for execution not linked in the database >`action{"did":"vacuum-extend-start-clean","siid":4,"aiid":1,"in":[10.0]}`<br />`action{"did":"vacuum-extend-stop-clean","siid":4,"aiid":2,"in":[]}`<br />`action{"did":"map-map-req","siid":6,"aiid":1,"in":[2.0]}`<br />`action{"did":"map-update-map","siid":6,"aiid":2,"in":[4.0]}`<br />`action{"did":"audio-position","siid":7,"aiid":1,"in":[]}`<br />`action{"did":"audio-play-sound","siid":7,"aiid":2,"in":[]}`<br />`action{"did":"time-delete-timer","siid":8,"aiid":1,"in":[3.0]}`<br /> |
|
||||
| HUIZUO ARIES For Bedroom | miio:basic | [huayi.light.ari013](#huayi-light-ari013) | Yes | Experimental support. Please report back if all channels are functional. Preferably share the debug log of property refresh and command responses |
|
||||
| HUIZUO ARIES For Living Room | miio:basic | [huayi.light.aries](#huayi-light-aries) | Yes | Experimental support. Please report back if all channels are functional. Preferably share the debug log of property refresh and command responses |
|
||||
|
@ -272,10 +272,10 @@ Currently the miio binding supports more than 300 different models.
|
|||
| Midea Air Conditioner v2 | miio:unsupported | midea.aircondition.v2 | No | |
|
||||
| Midea AC-Cool Golden | miio:unsupported | midea.aircondition.xa1 | No | |
|
||||
| Mi Robot Vacuum-Mop Essential | miio:basic | [mijia.vacuum.v2](#mijia-vacuum-v2) | Yes | This device may be overwhelmed if refresh is too frequent, slowing down the responses. Suggest to increase refresh time to 120 seconds |
|
||||
| Mijia Smart Pet Water Dispenser | miio:basic | [mmgg.pet_waterer.s1](#mmgg-pet_waterer-s1) | Yes | Identified manual actions for execution<br />`action{"did":"filter-reset-filter-life","siid":3,"aiid":1,"in":[]}`<br />`action{"did":"filter-cotton-reset-cotton-life","siid":5,"aiid":1,"in":[]}`<br />`action{"did":"remain-clean-time-reset-clean-time","siid":6,"aiid":1,"in":[]}`<br />Please test and feedback if they are working to they can be linked to a channel.<br />Experimental support. Please report back if all channels are functional. Preferably share the debug log of property refresh and command responses |
|
||||
| Mijia Smart Pet Water Dispenser | miio:basic | [mmgg.pet_waterer.s2](#mmgg-pet_waterer-s2) | Yes | Identified manual actions for execution<br />`action{"did":"filter-reset-filter-life","siid":3,"aiid":1,"in":[]}`<br />`action{"did":"filter-cotton-reset-cotton-life","siid":5,"aiid":1,"in":[]}`<br />`action{"did":"remain-clean-time-reset-clean-time","siid":6,"aiid":1,"in":[]}`<br />Please test and feedback if they are working to they can be linked to a channel.<br />Experimental support. Please report back if all channels are functional. Preferably share the debug log of property refresh and command responses |
|
||||
| Mijia Smart Pet Water Dispenser | miio:basic | [mmgg.pet_waterer.s3](#mmgg-pet_waterer-s3) | Yes | Identified manual actions for execution<br />`action{"did":"filter-reset-filter-life","siid":3,"aiid":1,"in":[]}`<br />`action{"did":"filter-cotton-reset-cotton-life","siid":5,"aiid":1,"in":[]}`<br />`action{"did":"remain-clean-time-reset-clean-time","siid":6,"aiid":1,"in":[]}`<br />Please test and feedback if they are working to they can be linked to a channel.<br />Experimental support. Please report back if all channels are functional. Preferably share the debug log of property refresh and command responses |
|
||||
| XIAOWAN Smart Pet Water Dispenser | miio:basic | [mmgg.pet_waterer.s4](#mmgg-pet_waterer-s4) | Yes | Identified manual actions for execution<br />`action{"did":"filter-reset-filter-life","siid":3,"aiid":1,"in":[]}`<br />`action{"did":"filter-cotton-reset-cotton-life","siid":5,"aiid":1,"in":[]}`<br />`action{"did":"remain-clean-time-reset-clean-time","siid":6,"aiid":1,"in":[]}`<br />Please test and feedback if they are working to they can be linked to a channel.<br />Experimental support. Please report back if all channels are functional. Preferably share the debug log of property refresh and command responses |
|
||||
| Mijia Smart Pet Water Dispenser | miio:basic | [mmgg.pet_waterer.s1](#mmgg-pet_waterer-s1) | Yes | Identified manual actions for execution<br />`action{"did":"filter-reset-filter-life","siid":3,"aiid":1,"in":[]}`<br />`action{"did":"filter-cotton-reset-cotton-life","siid":5,"aiid":1,"in":[]}`<br />`action{"did":"remain-clean-time-reset-clean-time","siid":6,"aiid":1,"in":[]}`<br />Please test and feedback if they are working so they can be linked to a channel.<br />Experimental support. Please report back if all channels are functional. Preferably share the debug log of property refresh and command responses |
|
||||
| Mijia Smart Pet Water Dispenser | miio:basic | [mmgg.pet_waterer.s2](#mmgg-pet_waterer-s2) | Yes | Identified manual actions for execution<br />`action{"did":"filter-reset-filter-life","siid":3,"aiid":1,"in":[]}`<br />`action{"did":"filter-cotton-reset-cotton-life","siid":5,"aiid":1,"in":[]}`<br />`action{"did":"remain-clean-time-reset-clean-time","siid":6,"aiid":1,"in":[]}`<br />Please test and feedback if they are working so they can be linked to a channel.<br />Experimental support. Please report back if all channels are functional. Preferably share the debug log of property refresh and command responses |
|
||||
| Mijia Smart Pet Water Dispenser | miio:basic | [mmgg.pet_waterer.s3](#mmgg-pet_waterer-s3) | Yes | Identified manual actions for execution<br />`action{"did":"filter-reset-filter-life","siid":3,"aiid":1,"in":[]}`<br />`action{"did":"filter-cotton-reset-cotton-life","siid":5,"aiid":1,"in":[]}`<br />`action{"did":"remain-clean-time-reset-clean-time","siid":6,"aiid":1,"in":[]}`<br />Please test and feedback if they are working so they can be linked to a channel.<br />Experimental support. Please report back if all channels are functional. Preferably share the debug log of property refresh and command responses |
|
||||
| XIAOWAN Smart Pet Water Dispenser | miio:basic | [mmgg.pet_waterer.s4](#mmgg-pet_waterer-s4) | Yes | Identified manual actions for execution<br />`action{"did":"filter-reset-filter-life","siid":3,"aiid":1,"in":[]}`<br />`action{"did":"filter-cotton-reset-cotton-life","siid":5,"aiid":1,"in":[]}`<br />`action{"did":"remain-clean-time-reset-clean-time","siid":6,"aiid":1,"in":[]}`<br />Please test and feedback if they are working so they can be linked to a channel.<br />Experimental support. Please report back if all channels are functional. Preferably share the debug log of property refresh and command responses |
|
||||
| MR.BOND | miio:basic | [mrbond.airer.m1pro](#mrbond-airer-m1pro) | Yes | Experimental support. Please report back if all channels are functional. Preferably share the debug log of property refresh and command responses |
|
||||
| MR.BOND | miio:basic | [mrbond.airer.m1s](#mrbond-airer-m1s) | Yes | Experimental support. Please report back if all channels are functional. Preferably share the debug log of property refresh and command responses |
|
||||
| MR.BOND | miio:basic | [mrbond.airer.m1super](#mrbond-airer-m1super) | Yes | Experimental support. Please report back if all channels are functional. Preferably share the debug log of property refresh and command responses |
|
||||
|
@ -361,7 +361,7 @@ Currently the miio binding supports more than 300 different models.
|
|||
| Viomi Cleaning Robot V-RVCLM21B | miio:basic | [viomi.vacuum.v6](#viomi-vacuum-v6) | Yes | |
|
||||
| Mi Robot Vacuum-Mop P | miio:basic | [viomi.vacuum.v7](#viomi-vacuum-v7) | Yes | |
|
||||
| Mi Robot Vacuum-Mop P | miio:basic | [viomi.vacuum.v8](#viomi-vacuum-v8) | Yes | |
|
||||
| Viomi S9 | miio:basic | [viomi.vacuum.v18](#viomi-vacuum-v18) | Yes | Identified manual actions for execution<br />`action{"did":"vacuum-start-sweep","siid":2,"aiid":1,"in":[]}`<br />`action{"did":"vacuum-stop-sweeping","siid":2,"aiid":2,"in":[]}`<br />`action{"did":"vacuum-pause","siid":2,"aiid":3,"in":[]}`<br />`action{"did":"vacuum-start-charge","siid":2,"aiid":4,"in":[]}`<br />`action{"did":"vacuum-stop-massage","siid":2,"aiid":5,"in":[]}`<br />`action{"did":"vacuum-start-mop","siid":2,"aiid":6,"in":[]}`<br />`action{"did":"vacuum-start-only-sweep","siid":2,"aiid":7,"in":[]}`<br />`action{"did":"vacuum-start-sweep-mop","siid":2,"aiid":8,"in":[]}`<br />`action{"did":"viomi-vacuum-reset-map","siid":4,"aiid":7,"in":[]}`<br />`action{"did":"viomi-vacuum-set-calibration","siid":4,"aiid":10,"in":[]}`<br />`action{"did":"viomi-vacuum-reset-consumable","siid":4,"aiid":11,"in":[35.0]}`<br />`action{"did":"viomi-vacuum-set-room-clean","siid":4,"aiid":13,"in":[36.0, 37.0, 38.0]}`<br />`action{"did":"order-del","siid":5,"aiid":2,"in":[1.0]}`<br />`action{"did":"order-get","siid":5,"aiid":3,"in":[]}`<br />`action{"did":"point-zone-start-point-clean","siid":6,"aiid":1,"in":[]}`<br />`action{"did":"point-zone-pause-point-clean","siid":6,"aiid":2,"in":[]}`<br />`action{"did":"point-zone-start-zone-clean","siid":6,"aiid":5,"in":[]}`<br />`action{"did":"point-zone-pause-zone-clean","siid":6,"aiid":6,"in":[]}`<br />`action{"did":"map-upload-by-maptype","siid":7,"aiid":1,"in":[]}`<br />`action{"did":"map-upload-by-mapid","siid":7,"aiid":2,"in":[]}`<br />`action{"did":"map-set-cur-map","siid":7,"aiid":3,"in":[2.0, 15.0]}`<br />`action{"did":"map-del-map","siid":7,"aiid":5,"in":[2.0]}`<br />`action{"did":"map-rename-map","siid":7,"aiid":7,"in":[2.0, 4.0]}`<br />`action{"did":"map-arrange-room","siid":7,"aiid":8,"in":[2.0, 5.0, 6.0, 14.0]}`<br />`action{"did":"map-split-room","siid":7,"aiid":9,"in":[2.0, 5.0, 7.0, 8.0, 14.0]}`<br />`action{"did":"map-rename-room","siid":7,"aiid":10,"in":[2.0, 7.0, 9.0, 14.0]}`<br />`action{"did":"map-get-map-list","siid":7,"aiid":11,"in":[]}`<br />`action{"did":"map-get-cleaning-path","siid":7,"aiid":12,"in":[12.0]}`<br />`action{"did":"map-set-new-map","siid":7,"aiid":13,"in":[]}`<br />`action{"did":"map-deal-new-map","siid":7,"aiid":14,"in":[16.0]}`<br />`action{"did":"voice-find-device","siid":8,"aiid":2,"in":[]}`<br />`action{"did":"voice-download-voice","siid":8,"aiid":3,"in":[3.0, 7.0, 8.0]}`<br />`action{"did":"voice-get-downloadstatus","siid":8,"aiid":4,"in":[]}`<br />Please test and feedback if they are working to they can be linked to a channel.<br />Experimental support. Please report back if all channels are functional. Preferably share the debug log of property refresh and command responses |
|
||||
| Viomi S9 | miio:basic | [viomi.vacuum.v18](#viomi-vacuum-v18) | Yes | Identified manual actions for execution<br />`action{"did":"vacuum-start-sweep","siid":2,"aiid":1,"in":[]}`<br />`action{"did":"vacuum-stop-sweeping","siid":2,"aiid":2,"in":[]}`<br />`action{"did":"vacuum-pause","siid":2,"aiid":3,"in":[]}`<br />`action{"did":"vacuum-start-charge","siid":2,"aiid":4,"in":[]}`<br />`action{"did":"vacuum-stop-massage","siid":2,"aiid":5,"in":[]}`<br />`action{"did":"vacuum-start-mop","siid":2,"aiid":6,"in":[]}`<br />`action{"did":"vacuum-start-only-sweep","siid":2,"aiid":7,"in":[]}`<br />`action{"did":"vacuum-start-sweep-mop","siid":2,"aiid":8,"in":[]}`<br />`action{"did":"viomi-vacuum-reset-map","siid":4,"aiid":7,"in":[]}`<br />`action{"did":"viomi-vacuum-set-calibration","siid":4,"aiid":10,"in":[]}`<br />`action{"did":"viomi-vacuum-reset-consumable","siid":4,"aiid":11,"in":[35.0]}`<br />`action{"did":"viomi-vacuum-set-room-clean","siid":4,"aiid":13,"in":[36.0, 37.0, 38.0]}`<br />`action{"did":"order-del","siid":5,"aiid":2,"in":[1.0]}`<br />`action{"did":"order-get","siid":5,"aiid":3,"in":[]}`<br />`action{"did":"point-zone-start-point-clean","siid":6,"aiid":1,"in":[]}`<br />`action{"did":"point-zone-pause-point-clean","siid":6,"aiid":2,"in":[]}`<br />`action{"did":"point-zone-start-zone-clean","siid":6,"aiid":5,"in":[]}`<br />`action{"did":"point-zone-pause-zone-clean","siid":6,"aiid":6,"in":[]}`<br />`action{"did":"map-upload-by-maptype","siid":7,"aiid":1,"in":[]}`<br />`action{"did":"map-upload-by-mapid","siid":7,"aiid":2,"in":[]}`<br />`action{"did":"map-set-cur-map","siid":7,"aiid":3,"in":[2.0, 15.0]}`<br />`action{"did":"map-del-map","siid":7,"aiid":5,"in":[2.0]}`<br />`action{"did":"map-rename-map","siid":7,"aiid":7,"in":[2.0, 4.0]}`<br />`action{"did":"map-arrange-room","siid":7,"aiid":8,"in":[2.0, 5.0, 6.0, 14.0]}`<br />`action{"did":"map-split-room","siid":7,"aiid":9,"in":[2.0, 5.0, 7.0, 8.0, 14.0]}`<br />`action{"did":"map-rename-room","siid":7,"aiid":10,"in":[2.0, 7.0, 9.0, 14.0]}`<br />`action{"did":"map-get-map-list","siid":7,"aiid":11,"in":[]}`<br />`action{"did":"map-get-cleaning-path","siid":7,"aiid":12,"in":[12.0]}`<br />`action{"did":"map-set-new-map","siid":7,"aiid":13,"in":[]}`<br />`action{"did":"map-deal-new-map","siid":7,"aiid":14,"in":[16.0]}`<br />`action{"did":"voice-find-device","siid":8,"aiid":2,"in":[]}`<br />`action{"did":"voice-download-voice","siid":8,"aiid":3,"in":[3.0, 7.0, 8.0]}`<br />`action{"did":"voice-get-downloadstatus","siid":8,"aiid":4,"in":[]}`<br />Please test and feedback if they are working so they can be linked to a channel.<br />Experimental support. Please report back if all channels are functional. Preferably share the debug log of property refresh and command responses |
|
||||
| VIOMI Internet Electric Water Heater 1A (60L) | miio:basic | [viomi.waterheater.e1](#viomi-waterheater-e1) | Yes | Experimental support. Please report back if all channels are functional. Preferably share the debug log of property refresh and command responses |
|
||||
| Mi Inverter Air Conditioner (1.5HP) | miio:basic | [xiaomi.aircondition.ma1](#xiaomi-aircondition-ma1) | Yes | Experimental support. Please report back if all channels are functional. Preferably share the debug log of property refresh and command responses |
|
||||
| Mi Inverter Air Conditioner (1.5HP, China Energy Label Level 1) | miio:basic | [xiaomi.aircondition.ma2](#xiaomi-aircondition-ma2) | Yes | Experimental support. Please report back if all channels are functional. Preferably share the debug log of property refresh and command responses |
|
||||
|
@ -481,14 +481,15 @@ Currently the miio binding supports more than 300 different models.
|
|||
| Mi Water Purifier v4 | miio:basic | [yunmi.waterpurifier.v4](#yunmi-waterpurifier-v4) | Yes | Experimental support. Please report back if all channels are functional. Preferably share the debug log of property refresh and command responses |
|
||||
| Smartmi Ventilation System | miio:basic | [zhimi.airfresh.va2](#zhimi-airfresh-va2) | Yes | |
|
||||
| Smartmi Fresh Air System (Heating) | miio:basic | [zhimi.airfresh.va4](#zhimi-airfresh-va4) | Yes | |
|
||||
| Mi Fresh Air Ventilator C1-80 | miio:basic | [zhimi.airfresh.ua1](#zhimi-airfresh-ua1) | Yes | Identified manual actions for execution<br />`action{"did":"filter-reset-filter-life","siid":4,"aiid":1,"in":[1.0]}`<br />Please test and feedback if they are working so they can be linked to a channel. |
|
||||
| Mi PM2.5 Air Quality Monitor | miio:basic | [zhimi.airmonitor.v1](#zhimi-airmonitor-v1) | Yes | |
|
||||
| Mi Air Purifier 2 (mini) | miio:basic | [zhimi.airpurifier.m1](#zhimi-airpurifier-m1) | Yes | |
|
||||
| Mi Air Purifier 2 | miio:basic | [zhimi.airpurifier.m2](#zhimi-airpurifier-m2) | Yes | |
|
||||
| Mi Air Purifier 2S | miio:basic | [zhimi.airpurifier.ma1](#zhimi-airpurifier-ma1) | Yes | |
|
||||
| Mi Air Purifier 2S | miio:basic | [zhimi.airpurifier.ma2](#zhimi-airpurifier-ma2) | Yes | |
|
||||
| Mi Air Purifier 3 | miio:basic | [zhimi.airpurifier.ma4](#zhimi-airpurifier-ma4) | Yes | Identified manual actions for execution<br />`action{"did":"filter-reset-filter-life","siid":4,"aiid":1,"in":[]}`<br />`action{"did":"button-toggle","siid":8,"aiid":1,"in":[]}`<br />`action{"did":"button-toggle-mode","siid":8,"aiid":2,"in":[]}`<br />Please test and feedback if they are working to they can be linked to a channel.<br />Experimental support. Please report back if all channels are functional. Preferably share the debug log of property refresh and command responses |
|
||||
| Mi Air Purifier 3 | miio:basic | [zhimi.airpurifier.ma4](#zhimi-airpurifier-ma4) | Yes | Identified manual actions for execution<br />`action{"did":"filter-reset-filter-life","siid":4,"aiid":1,"in":[]}`<br />`action{"did":"button-toggle","siid":8,"aiid":1,"in":[]}`<br />`action{"did":"button-toggle-mode","siid":8,"aiid":2,"in":[]}`<br />Please test and feedback if they are working so they can be linked to a channel.<br />Experimental support. Please report back if all channels are functional. Preferably share the debug log of property refresh and command responses |
|
||||
| Mi Air Purifier 2S | miio:basic | [zhimi.airpurifier.mb1](#zhimi-airpurifier-mb1) | Yes | |
|
||||
| Mi Air Purifier 3/3H | miio:basic | [zhimi.airpurifier.mb3](#zhimi-airpurifier-mb3) | Yes | Identified manual actions for execution<br />`action{"did":"filter-reset-filter-life","siid":4,"aiid":1,"in":[]}`<br />`action{"did":"button-toggle","siid":8,"aiid":1,"in":[]}`<br />`action{"did":"button-toggle-mode","siid":8,"aiid":2,"in":[]}`<br />Please test and feedback if they are working to they can be linked to a channel.<br />Experimental support. Please report back if all channels are functional. Preferably share the debug log of property refresh and command responses |
|
||||
| Mi Air Purifier 3/3H | miio:basic | [zhimi.airpurifier.mb3](#zhimi-airpurifier-mb3) | Yes | Identified manual actions for execution<br />`action{"did":"filter-reset-filter-life","siid":4,"aiid":1,"in":[]}`<br />`action{"did":"button-toggle","siid":8,"aiid":1,"in":[]}`<br />`action{"did":"button-toggle-mode","siid":8,"aiid":2,"in":[]}`<br />Please test and feedback if they are working so they can be linked to a channel.<br />Experimental support. Please report back if all channels are functional. Preferably share the debug log of property refresh and command responses |
|
||||
| Mi Air Purifier 3C | miio:basic | [zhimi.airpurifier.mb4](#zhimi-airpurifier-mb4) | Yes | Specified action for filter reset <br />`action{"did":"filter-reset-filter-life","siid":4,"aiid":1,"in":[3.0]}`<br />However, this has not been successfully tested yet. |
|
||||
| Mi Air Purifier 2S | miio:basic | [zhimi.airpurifier.mc1](#zhimi-airpurifier-mc1) | Yes | |
|
||||
| Mi Air Purifier 2H | miio:basic | [zhimi.airpurifier.mc2](#zhimi-airpurifier-mc2) | Yes | |
|
||||
|
@ -513,7 +514,7 @@ Currently the miio binding supports more than 300 different models.
|
|||
| Smartmi Standing Fan 2S | miio:basic | [zhimi.fan.za4](#zhimi-fan-za4) | Yes | |
|
||||
| Smartmi Standing Fan 3 | miio:basic | [zhimi.fan.za5](#zhimi-fan-za5) | Yes | Experimental support. Please report back if all channels are functional. Preferably share the debug log of property refresh and command responses |
|
||||
| Mi Smart Space Heater S | miio:basic | [zhimi.heater.ma2](#zhimi-heater-ma2) | Yes | Experimental support. Please report back if all channels are functional. Preferably share the debug log of property refresh and command responses |
|
||||
| Mi Smart Baseboard Heater E | miio:basic | [zhimi.heater.ma3](#zhimi-heater-ma3) | Yes | Identified manual actions for execution<br />`action{"did":"private-service-toggle-switch","siid":8,"aiid":1,"in":[]}`<br />Please test and feedback if they are working to they can be linked to a channel.<br />Experimental support. Please report back if all channels are functional. Preferably share the debug log of property refresh and command responses |
|
||||
| Mi Smart Baseboard Heater E | miio:basic | [zhimi.heater.ma3](#zhimi-heater-ma3) | Yes | Identified manual actions for execution<br />`action{"did":"private-service-toggle-switch","siid":8,"aiid":1,"in":[]}`<br />Please test and feedback if they are working so they can be linked to a channel.<br />Experimental support. Please report back if all channels are functional. Preferably share the debug log of property refresh and command responses |
|
||||
| Mi Smart Space Heater S | miio:basic | [zhimi.heater.mc2](#zhimi-heater-mc2) | Yes | Experimental support. Please report back if all channels are functional. Preferably share the debug log of property refresh and command responses |
|
||||
| Smartmi Smart Fan | miio:basic | [zhimi.heater.na1](#zhimi-heater-na1) | Yes | Experimental support. Please report back if all channels are functional. Preferably share the debug log of property refresh and command responses |
|
||||
| Smartmi Smart Fan Heater | miio:basic | [zhimi.heater.nb1](#zhimi-heater-nb1) | Yes | Experimental support. Please report back if all channels are functional. Preferably share the debug log of property refresh and command responses |
|
||||
|
@ -708,6 +709,7 @@ Note, not all the values need to be in the json file, e.g. a subset of the param
|
|||
| countdown | Number:Time | Imilab Timer - Countdown | |
|
||||
| task-switch | Switch | Imilab Timer - Task Switch | |
|
||||
| countdown-info | Switch | Imilab Timer - Countdown Info | |
|
||||
| bt-gw | String | BT Gateway | Value mapping `["disable"="Disable","enable"="Enable"]` |
|
||||
|
||||
### Mi Smart Plug WiFi (<a name="chuangmi-plug-hmi205">chuangmi.plug.hmi205</a>) Channels
|
||||
|
||||
|
@ -4355,6 +4357,24 @@ Note, not all the values need to be in the json file, e.g. a subset of the param
|
|||
| led_level | Number | Led - Brightness | Value mapping `["0"="High","1"="Low","2"="Idle"]` |
|
||||
| temperature | Number:Temperature | Temperature | |
|
||||
|
||||
### Mi Fresh Air Ventilator C1-80 (<a name="zhimi-airfresh-ua1">zhimi.airfresh.ua1</a>) Channels
|
||||
|
||||
| Channel | Type | Description | Comment |
|
||||
|----------------------|----------------------|------------------------------------------|------------|
|
||||
| actions | String | Actions | Value mapping `["filter-reset-filter-life"="Filter Reset Filter Life"]` |
|
||||
| on | Switch | Air Fresh - Switch Status | |
|
||||
| fault | Number | Device Fault | Value mapping `["0"="No Faults"]` |
|
||||
| fan_level | Number | Air Fresh - Fan Level | Value mapping `["1"="Level1","2"="Level2","3"="Level3"]` |
|
||||
| heater | Switch | Heater | |
|
||||
| filter_used_time | Number:Time | Filter - Filter Used Time | |
|
||||
| filter_life_level | Number:Dimensionless | Filter - Filter Life Level | |
|
||||
| physical_controls_locked | Switch | Physical Control Locked - Physical Control Locked | |
|
||||
| alarm | Switch | Alarm - Alarm | |
|
||||
| brightness | Dimmer | Indicator Light - Brightness | |
|
||||
| motor_a_speed_rpm | Number | Custom Serveice - Motor A Speed Rpm | |
|
||||
| motor_b_speed_rpm | Number | Custom Serveice - Motor B Speed Rpm | |
|
||||
| temperature | Number:Temperature | Custom Serveice - Temperature | |
|
||||
|
||||
### Mi PM2.5 Air Quality Monitor (<a name="zhimi-airmonitor-v1">zhimi.airmonitor.v1</a>) Channels
|
||||
|
||||
| Channel | Type | Description | Comment |
|
||||
|
@ -5484,6 +5504,7 @@ Number:Time off_duration "Imilab Timer - Off Duration" (G_plug) {channel="miio:b
|
|||
Number:Time countdown "Imilab Timer - Countdown" (G_plug) {channel="miio:basic:plug:countdown"}
|
||||
Switch task_switch "Imilab Timer - Task Switch" (G_plug) {channel="miio:basic:plug:task-switch"}
|
||||
Switch countdown_info "Imilab Timer - Countdown Info" (G_plug) {channel="miio:basic:plug:countdown-info"}
|
||||
String bt_gw "BT Gateway" (G_plug) {channel="miio:basic:plug:bt-gw"}
|
||||
```
|
||||
|
||||
### Mi Smart Plug WiFi (chuangmi.plug.hmi205) item file lines
|
||||
|
@ -9810,6 +9831,27 @@ Number led_level "Led - Brightness" (G_airfresh) {channel="miio:basic:airfresh:l
|
|||
Number:Temperature temperature "Temperature" (G_airfresh) {channel="miio:basic:airfresh:temperature"}
|
||||
```
|
||||
|
||||
### Mi Fresh Air Ventilator C1-80 (zhimi.airfresh.ua1) item file lines
|
||||
|
||||
note: Autogenerated example. Replace the id (airfresh) in the channel with your own. Replace `basic` with `generic` in the thing UID depending on how your thing was discovered.
|
||||
|
||||
```
|
||||
Group G_airfresh "Mi Fresh Air Ventilator C1-80" <status>
|
||||
String actions "Actions" (G_airfresh) {channel="miio:basic:airfresh:actions"}
|
||||
Switch on "Air Fresh - Switch Status" (G_airfresh) {channel="miio:basic:airfresh:on"}
|
||||
Number fault "Device Fault" (G_airfresh) {channel="miio:basic:airfresh:fault"}
|
||||
Number fan_level "Air Fresh - Fan Level" (G_airfresh) {channel="miio:basic:airfresh:fan_level"}
|
||||
Switch heater "Heater" (G_airfresh) {channel="miio:basic:airfresh:heater"}
|
||||
Number:Time filter_used_time "Filter - Filter Used Time" (G_airfresh) {channel="miio:basic:airfresh:filter_used_time"}
|
||||
Number:Dimensionless filter_life_level "Filter - Filter Life Level" (G_airfresh) {channel="miio:basic:airfresh:filter_life_level"}
|
||||
Switch physical_controls_locked "Physical Control Locked - Physical Control Locked" (G_airfresh) {channel="miio:basic:airfresh:physical_controls_locked"}
|
||||
Switch alarm "Alarm - Alarm" (G_airfresh) {channel="miio:basic:airfresh:alarm"}
|
||||
Dimmer brightness "Indicator Light - Brightness" (G_airfresh) {channel="miio:basic:airfresh:brightness"}
|
||||
Number motor_a_speed_rpm "Custom Serveice - Motor A Speed Rpm" (G_airfresh) {channel="miio:basic:airfresh:motor_a_speed_rpm"}
|
||||
Number motor_b_speed_rpm "Custom Serveice - Motor B Speed Rpm" (G_airfresh) {channel="miio:basic:airfresh:motor_b_speed_rpm"}
|
||||
Number:Temperature temperature "Custom Serveice - Temperature" (G_airfresh) {channel="miio:basic:airfresh:temperature"}
|
||||
```
|
||||
|
||||
### Mi PM2.5 Air Quality Monitor (zhimi.airmonitor.v1) item file lines
|
||||
|
||||
note: Autogenerated example. Replace the id (airmonitor) in the channel with your own. Replace `basic` with `generic` in the thing UID depending on how your thing was discovered.
|
||||
|
|
|
@ -0,0 +1,201 @@
|
|||
<?xml version="1.0" encoding="UTF-8"?>
|
||||
<thing:thing-descriptions bindingId="anel"
|
||||
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
|
||||
xmlns:thing="https://openhab.org/schemas/thing-description/v1.0.0"
|
||||
xsi:schemaLocation="https://openhab.org/schemas/thing-description/v1.0.0 https://openhab.org/schemas/thing-description-1.0.0.xsd">
|
||||
|
||||
<thing-type id="home">
|
||||
<label>HOME</label>
|
||||
<description>Anel device with 3 controllable outlets without IO ports.</description>
|
||||
|
||||
<!-- Example channel ID: anel:home:mydevice:prop#temperature -->
|
||||
<channel-groups>
|
||||
<channel-group id="prop" typeId="propertiesGroup"/>
|
||||
|
||||
<channel-group id="r1" typeId="relayGroup"/>
|
||||
<channel-group id="r2" typeId="relayGroup"/>
|
||||
<channel-group id="r3" typeId="relayGroup"/>
|
||||
</channel-groups>
|
||||
|
||||
<properties>
|
||||
<property name="vendor">ANEL Elektronik AG</property>
|
||||
<property name="modelId">NET-PwrCtrl HOME</property>
|
||||
</properties>
|
||||
<representation-property>macAddress</representation-property>
|
||||
|
||||
<config-description-ref uri="thing-type:anel:config"/>
|
||||
</thing-type>
|
||||
|
||||
<thing-type id="simple-firmware">
|
||||
<label>PRO / POWER</label>
|
||||
<description>Anel device with 8 controllable outlets without IO ports.</description>
|
||||
|
||||
<channel-groups>
|
||||
<channel-group id="prop" typeId="propertiesGroup"/>
|
||||
|
||||
<!-- Example channel ID: anel:simple-firmware:mydevice:r1#state -->
|
||||
<channel-group id="r1" typeId="relayGroup"/>
|
||||
<channel-group id="r2" typeId="relayGroup"/>
|
||||
<channel-group id="r3" typeId="relayGroup"/>
|
||||
<channel-group id="r4" typeId="relayGroup"/>
|
||||
<channel-group id="r5" typeId="relayGroup"/>
|
||||
<channel-group id="r6" typeId="relayGroup"/>
|
||||
<channel-group id="r7" typeId="relayGroup"/>
|
||||
<channel-group id="r8" typeId="relayGroup"/>
|
||||
</channel-groups>
|
||||
|
||||
<properties>
|
||||
<property name="vendor">ANEL Elektronik AG</property>
|
||||
<property name="modelId">NET-PwrCtrl PRO / POWER</property>
|
||||
</properties>
|
||||
<representation-property>macAddress</representation-property>
|
||||
|
||||
<config-description-ref uri="thing-type:anel:config"/>
|
||||
</thing-type>
|
||||
|
||||
<thing-type id="advanced-firmware">
|
||||
<label>ADV / IO / HUT</label>
|
||||
<description>Anel device with 8 controllable outlets / relays and possibly 8 IO ports.</description>
|
||||
|
||||
<channel-groups>
|
||||
<channel-group id="prop" typeId="propertiesGroup"/>
|
||||
|
||||
<channel-group id="r1" typeId="relayGroup"/>
|
||||
<channel-group id="r2" typeId="relayGroup"/>
|
||||
<channel-group id="r3" typeId="relayGroup"/>
|
||||
<channel-group id="r4" typeId="relayGroup"/>
|
||||
<channel-group id="r5" typeId="relayGroup"/>
|
||||
<channel-group id="r6" typeId="relayGroup"/>
|
||||
<channel-group id="r7" typeId="relayGroup"/>
|
||||
<channel-group id="r8" typeId="relayGroup"/>
|
||||
|
||||
<channel-group id="io1" typeId="ioGroup"/>
|
||||
<channel-group id="io2" typeId="ioGroup"/>
|
||||
<channel-group id="io3" typeId="ioGroup"/>
|
||||
<channel-group id="io4" typeId="ioGroup"/>
|
||||
<channel-group id="io5" typeId="ioGroup"/>
|
||||
<channel-group id="io6" typeId="ioGroup"/>
|
||||
<channel-group id="io7" typeId="ioGroup"/>
|
||||
<channel-group id="io8" typeId="ioGroup"/>
|
||||
|
||||
<!-- Example channel ID: anel:advanced-firmware:mydevice:sensor#humidity -->
|
||||
<channel-group id="sensor" typeId="sensorGroup"/>
|
||||
</channel-groups>
|
||||
|
||||
<properties>
|
||||
<property name="vendor">ANEL Elektronik AG</property>
|
||||
<property name="modelId">NET-PwrCtrl ADV / IO / HUT</property>
|
||||
</properties>
|
||||
<representation-property>macAddress</representation-property>
|
||||
|
||||
<config-description-ref uri="thing-type:anel:config"/>
|
||||
</thing-type>
|
||||
|
||||
<channel-group-type id="propertiesGroup">
|
||||
<label>Device Properties</label>
|
||||
<description>Device properties</description>
|
||||
<channels>
|
||||
<channel id="name" typeId="name-channel"/>
|
||||
<channel id="temperature" typeId="temperature-channel"/>
|
||||
</channels>
|
||||
</channel-group-type>
|
||||
<channel-group-type id="relayGroup">
|
||||
<label>Relay / Socket</label>
|
||||
<description>A relay / socket</description>
|
||||
<channels>
|
||||
<channel id="name" typeId="relayName-channel"/>
|
||||
<channel id="locked" typeId="relayLocked-channel"/>
|
||||
<channel id="state" typeId="relayState-channel"/>
|
||||
</channels>
|
||||
</channel-group-type>
|
||||
<channel-group-type id="ioGroup">
|
||||
<label>I/O Port</label>
|
||||
<description>An Input / Output Port</description>
|
||||
<channels>
|
||||
<channel id="name" typeId="ioName-channel"/>
|
||||
<channel id="mode" typeId="ioMode-channel"/>
|
||||
<channel id="state" typeId="ioState-channel"/>
|
||||
<channel id="event" typeId="system.rawbutton"/>
|
||||
</channels>
|
||||
</channel-group-type>
|
||||
<channel-group-type id="sensorGroup">
|
||||
<label>Sensor</label>
|
||||
<description>Optional sensor values</description>
|
||||
<channels>
|
||||
<channel id="temperature" typeId="sensorTemperature-channel"/>
|
||||
<channel id="humidity" typeId="sensorHumidity-channel"/>
|
||||
<channel id="brightness" typeId="sensorBrightness-channel"/>
|
||||
</channels>
|
||||
</channel-group-type>
|
||||
|
||||
<channel-type id="name-channel">
|
||||
<item-type>String</item-type>
|
||||
<label>Device Name</label>
|
||||
<description>The name of the Anel device</description>
|
||||
<state readOnly="true"/>
|
||||
</channel-type>
|
||||
<channel-type id="temperature-channel">
|
||||
<item-type>Number:Temperature</item-type>
|
||||
<label>Anel Device Temperature</label>
|
||||
<description>The value of the built-in temperature sensor of the Anel device</description>
|
||||
<state readOnly="true"/>
|
||||
</channel-type>
|
||||
|
||||
<channel-type id="relayName-channel">
|
||||
<item-type>String</item-type>
|
||||
<label>Relay Name</label>
|
||||
<description>The name of the relay / socket</description>
|
||||
<state readOnly="true"/>
|
||||
</channel-type>
|
||||
<channel-type id="relayLocked-channel" advanced="true">
|
||||
<item-type>Switch</item-type>
|
||||
<label>Relay Locked</label>
|
||||
<description>Whether or not the relay is locked</description>
|
||||
<state readOnly="true"/>
|
||||
</channel-type>
|
||||
<channel-type id="relayState-channel">
|
||||
<item-type>Switch</item-type>
|
||||
<label>Relay State</label>
|
||||
<description>The state of the relay / socket (read-only if locked!)</description>
|
||||
<autoUpdatePolicy>veto</autoUpdatePolicy><!-- updates are only sent in non-locked mode -->
|
||||
</channel-type>
|
||||
|
||||
<channel-type id="ioName-channel">
|
||||
<item-type>String</item-type>
|
||||
<label>IO Name</label>
|
||||
<description>The name of the I/O port</description>
|
||||
<state readOnly="true"/>
|
||||
</channel-type>
|
||||
<channel-type id="ioMode-channel" advanced="true">
|
||||
<item-type>Switch</item-type>
|
||||
<label>IO is Input</label>
|
||||
<description>Whether the port is configured as input (true) or output (false)</description>
|
||||
<state readOnly="true"/>
|
||||
</channel-type>
|
||||
<channel-type id="ioState-channel">
|
||||
<item-type>Switch</item-type>
|
||||
<label>IO State</label>
|
||||
<description>The state of the I/O port (read-only for input ports)</description>
|
||||
<autoUpdatePolicy>veto</autoUpdatePolicy><!-- updates are only sent in output mode -->
|
||||
</channel-type>
|
||||
|
||||
<channel-type id="sensorTemperature-channel">
|
||||
<item-type>Number:Temperature</item-type>
|
||||
<label>Sensor Temperature</label>
|
||||
<description>The temperature value of the optional sensor</description>
|
||||
<state readOnly="true"/>
|
||||
</channel-type>
|
||||
<channel-type id="sensorHumidity-channel">
|
||||
<item-type>Number</item-type>
|
||||
<label>Sensor Humidity</label>
|
||||
<description>The humidity value of the optional sensor</description>
|
||||
<state readOnly="true"/>
|
||||
</channel-type>
|
||||
<channel-type id="sensorBrightness-channel">
|
||||
<item-type>Number</item-type>
|
||||
<label>Sensor Brightness</label>
|
||||
<description>The brightness value of the optional sensor</description>
|
||||
<state readOnly="true"/>
|
||||
</channel-type>
|
||||
|
||||
</thing:thing-descriptions>
|
|
@ -29,7 +29,7 @@
|
|||
<channel id="switch" typeId="switch"/>
|
||||
<channel id="target" typeId="targetTemperature">
|
||||
<label>Temperature</label>
|
||||
<description>Temperature of the selected program</description>
|
||||
<description>Temperature of the selected program (10 °C = cold)</description>
|
||||
</channel>
|
||||
<channel id="spinningspeed" typeId="spinningspeed"/>
|
||||
<channel id="powerConsumption" typeId="powerConsumption"/>
|
||||
|
|
|
@ -38,6 +38,10 @@
|
|||
<label>Password</label>
|
||||
<description>Password for the registered Miele@home user.</description>
|
||||
</parameter>
|
||||
<parameter name="language" type="text" required="false">
|
||||
<label>Language</label>
|
||||
<description>Language for state, program and phase texts. Leave blank for system language.</description>
|
||||
</parameter>
|
||||
</config-description>
|
||||
</bridge-type>
|
||||
|
||||
|
|
Loading…
Reference in New Issue