Updated external content (Jenkins build 418)
parent
1ed0e0378a
commit
836102a2bd
File diff suppressed because one or more lines are too long
|
@ -0,0 +1,57 @@
|
|||
---
|
||||
id: jsscripting
|
||||
label: JavaScript Scripting
|
||||
title: JavaScript Scripting - Automation
|
||||
type: automation
|
||||
description: "This add-on provides support for JavaScript (ECMAScript 2021+) that can be used as a scripting language within automation rules."
|
||||
since: 3x
|
||||
install: manual
|
||||
---
|
||||
|
||||
<!-- Attention authors: Do not edit directly. Please add your changes to the appropriate source repository -->
|
||||
|
||||
{% include base.html %}
|
||||
|
||||
# JavaScript Scripting
|
||||
|
||||
This add-on provides support for JavaScript (ECMAScript 2021+) that can be used as a scripting language within automation rules.
|
||||
|
||||
## Creating JavaScript Scripts
|
||||
|
||||
When this add-on is installed, JavaScript script actions will be run by this add-on and allow ECMAScript 2021+ features.
|
||||
|
||||
Alternatively, you can create scripts in the `automation/jsr223` configuration directory.
|
||||
If you create an empty file called `test.js`, you will see a log line with information similar to:
|
||||
|
||||
```text
|
||||
... [INFO ] [.a.m.s.r.i.l.ScriptFileWatcher:150 ] - Loading script 'test.js'
|
||||
```
|
||||
|
||||
To enable debug logging, use the [console logging]({{base}}/administration/logging.html) commands to enable debug logging for the automation functionality:
|
||||
|
||||
```text
|
||||
log:set DEBUG org.openhab.core.automation
|
||||
```
|
||||
|
||||
For more information on the available APIs in scripts see the [JSR223 Scripting]({{base}}/configuration/jsr223.html) documentation.
|
||||
|
||||
## Script Examples
|
||||
|
||||
JavaScript scripts provide access to almost all the functionality in an openHAB runtime environment.
|
||||
As a simple example, the following script logs "Hello, World!".
|
||||
Note that `console.log` will usually not work since the output has no terminal to display the text.
|
||||
The openHAB server uses the [SLF4J](https://www.slf4j.org/) library for logging.
|
||||
|
||||
```js
|
||||
const LoggerFactory = Java.type('org.slf4j.LoggerFactory');
|
||||
|
||||
LoggerFactory.getLogger("org.openhab.core.automation.examples").info("Hello world!");
|
||||
```
|
||||
|
||||
Depending on the openHAB logging configuration, you may need to prefix logger names with `org.openhab.core.automation` for them to show up in the log file (or you modify the logging configuration).
|
||||
|
||||
The script uses the [LoggerFactory](https://www.slf4j.org/apidocs/org/slf4j/Logger.html) to obtain a named logger and then logs a message like:
|
||||
|
||||
```text
|
||||
... [INFO ] [.openhab.core.automation.examples:-2 ] - Hello world!
|
||||
```
|
|
@ -107,13 +107,13 @@ The following channels are supported:
|
|||
|
||||
| Channel Type ID | Item Type | Description |
|
||||
|---------------------|-----------|-------------------------------------------------------------------------------------------|
|
||||
| changedByUser | String | This channel reports the user that last changed the state of the alarm. |
|
||||
| changedVia | String | This channel reports the method used to change the status. |
|
||||
| timestamp | DateTime | This channel reports the last time the alarm status was changed. |
|
||||
| installationName | String | This channel reports the installation name. |
|
||||
| installationId | Number | This channel reports the installation ID. |
|
||||
| changedByUser | String | This channel reports the user that last changed the state of the alarm. |
|
||||
| changedVia | String | This channel reports the method used to change the status. |
|
||||
| timestamp | DateTime | This channel reports the last time the alarm status was changed. |
|
||||
| installationName | String | This channel reports the installation name. |
|
||||
| installationId | Number | This channel reports the installation ID. |
|
||||
| alarmStatus | String | This channel is used to arm/disarm the alarm. Available alarm status are "DISARMED", "ARMED_HOME" and "ARMED_AWAY".|
|
||||
| alarmTriggerChannel | trigger | This is a trigger channel that receives events.|
|
||||
| alarmTriggerChannel | trigger | This is a trigger channel that receives events. |
|
||||
|
||||
### Verisure Yaleman SmartLock
|
||||
|
||||
|
@ -128,11 +128,11 @@ The following channels are supported:
|
|||
|
||||
| Channel Type ID | Item Type | Description |
|
||||
|-------------------------|-----------|----------------------------------------------------------------------------------------------------------|
|
||||
| changedByUser | String | This channel reports the user that last changed the state of the alarm. |
|
||||
| changedByUser | String | This channel reports the user that last changed the state of the alarm. |
|
||||
| timestamp | DateTime | This channel reports the last time the alarm status was changed. |
|
||||
| changedVia | String | This channel reports the method used to change the status. |
|
||||
| motorJam | Switch | This channel reports if the SmartLock motor has jammed. |
|
||||
| location | String | This channel reports the location of the device. |
|
||||
| changedVia | String | This channel reports the method used to change the status. |
|
||||
| motorJam | Switch | This channel reports if the SmartLock motor has jammed. |
|
||||
| location | String | This channel reports the location of the device. |
|
||||
| installationName | String | This channel reports the installation name. |
|
||||
| installationId | Number | This channel reports the installation ID. |
|
||||
| smartLockStatus | Switch | This channel is used to lock/unlock. |
|
||||
|
@ -146,7 +146,7 @@ The following channels are supported:
|
|||
#### Configuration Options
|
||||
|
||||
* `deviceId` - Device Id
|
||||
* Sensor Id. Example 5A4C35FT (Note: Verisure ID, found in the Verisure App or My Pages or on the sensor itself)
|
||||
* Sensor Id. Example 5A4C35FT (Note: Verisure ID, found in the Verisure App or My Pages or on the sensor itself)
|
||||
|
||||
#### Channels
|
||||
|
||||
|
@ -166,7 +166,7 @@ The following channels are supported:
|
|||
#### Configuration Options
|
||||
|
||||
* `deviceId` - Device Id
|
||||
* Sensor Id. Example 5A4C35FT (Note: Verisure ID, found in the Verisure App or on the sensor itself)
|
||||
* Sensor Id. Example 5A4C35FT (Note: Verisure ID, found in the Verisure App or on the sensor itself)
|
||||
|
||||
#### Channels
|
||||
|
||||
|
@ -175,34 +175,36 @@ The following channels are supported:
|
|||
| Channel Type ID | Item Type | Description |
|
||||
|-----------------------------|-----------------------|-----------------------------------------------------------------------------|
|
||||
| temperature | Number:Temperature | This channel reports the current temperature. |
|
||||
| humidity | Number | This channel reports the current humidity in percentage. |
|
||||
| humidity | Number | This channel reports the current humidity in percentage. |
|
||||
| humidityEnabled | Switch | This channel reports if the Climate is device capable of reporting humidity.|
|
||||
| timestamp | DateTime | This channel reports the last time this sensor was updated. |
|
||||
| timestamp | DateTime | This channel reports the last time this sensor was updated. |
|
||||
| location | String | This channel reports the location of the device. |
|
||||
| installationName | String | This channel reports the installation name. |
|
||||
| installationId | Number | This channel reports the installation ID. |
|
||||
| smokeDetectorTriggerChannel | trigger | This is a trigger channel that receives events.|
|
||||
| lowBattery | Switch | This channel reports if the battery level is low. |
|
||||
| smokeDetectorTriggerChannel | trigger | This is a trigger channel that receives events. |
|
||||
|
||||
### Verisure Water Detector
|
||||
|
||||
#### Configuration Options
|
||||
|
||||
* `deviceId` - Device Id
|
||||
* Sensor Id. Example 5A4C35FT (Note: Verisure ID, found in the Verisure App or My Pages or on the sensor itself)
|
||||
* Sensor Id. Example 5A4C35FT (Note: Verisure ID, found in the Verisure App or My Pages or on the sensor itself)
|
||||
|
||||
#### Channels
|
||||
|
||||
The following channels are supported:
|
||||
|
||||
|
||||
| Channel Type ID | Item Type | Description |
|
||||
|-----------------------------|-----------------------|--------------------------------------------------------------|
|
||||
| temperature | Number:Temperature | This channel reports the current temperature. |
|
||||
|
||||
| Channel Type ID | Item Type | Description |
|
||||
|-----------------------------|-----------------------|--------------------------------------------------------------|
|
||||
| temperature | Number:Temperature | This channel reports the current temperature. |
|
||||
| timestamp | DateTime | This channel reports the last time this sensor was updated. |
|
||||
| location | String | This channel reports the location of the device. |
|
||||
| installationName | String | This channel reports the installation name. |
|
||||
| installationId | Number | This channel reports the installation ID. |
|
||||
| waterDetectorTriggerChannel | trigger | This is a trigger channel that receives events. |
|
||||
| location | String | This channel reports the location of the device. |
|
||||
| installationName | String | This channel reports the installation name. |
|
||||
| installationId | Number | This channel reports the installation ID. |
|
||||
| waterDetectorTriggerChannel | trigger | This is a trigger channel that receives events. |
|
||||
|
||||
|
||||
### Verisure Siren
|
||||
|
@ -210,47 +212,48 @@ The following channels are supported:
|
|||
#### Configuration Options
|
||||
|
||||
* `deviceId` - Device Id
|
||||
* Sensor Id. Example 5A4C35FT (Note: Verisure ID, found in the Verisure App or My Pages or on the sensor itself)
|
||||
* Sensor Id. Example 5A4C35FT (Note: Verisure ID, found in the Verisure App or My Pages or on the sensor itself)
|
||||
|
||||
#### Channels
|
||||
|
||||
The following channels are supported:
|
||||
|
||||
| Channel Type ID | Item Type | Description |
|
||||
|---------------------|-----------------------|------------------------------------------------------------|
|
||||
| temperature | Number:Temperature | This channel reports the current temperature. |
|
||||
|
||||
| Channel Type ID | Item Type | Description |
|
||||
|---------------------|-----------------------|------------------------------------------------------------|
|
||||
| temperature | Number:Temperature | This channel reports the current temperature. |
|
||||
| timestamp | DateTime | This channel reports the last time this sensor was updated.|
|
||||
| location | String | This channel reports the location. |
|
||||
| installationName | String | This channel reports the installation name. |
|
||||
| installationId | Number | This channel reports the installation ID. |
|
||||
| sirenTriggerChannel | trigger | This is a trigger channel that receives events. |
|
||||
| location | String | This channel reports the location. |
|
||||
| installationName | String | This channel reports the installation name. |
|
||||
| installationId | Number | This channel reports the installation ID. |
|
||||
| lowBattery | Switch | This channel reports if the battery level is low. |
|
||||
| sirenTriggerChannel | trigger | This is a trigger channel that receives events. |
|
||||
|
||||
### Verisure Night Control
|
||||
|
||||
#### Configuration Options
|
||||
|
||||
* `deviceId` - Device Id
|
||||
* Sensor Id. Example 5A4C35FT (Note: Verisure ID, found in the Verisure App or My Pages or on the sensor itself)
|
||||
* Sensor Id. Example 5A4C35FT (Note: Verisure ID, found in the Verisure App or My Pages or on the sensor itself)
|
||||
|
||||
#### Channels
|
||||
|
||||
The following channels are supported:
|
||||
|
||||
| Channel Type ID | Item Type | Description |
|
||||
|----------------------------|-----------------------|------------------------------------------------------------|
|
||||
| temperature | Number:Temperature | This channel reports the current temperature. |
|
||||
The following channels are supported:
|
||||
| Channel Type ID | Item Type | Description |
|
||||
|----------------------------|-----------------------|------------------------------------------------------------|
|
||||
| temperature | Number:Temperature | This channel reports the current temperature. |
|
||||
| timestamp | DateTime | This channel reports the last time this sensor was updated.|
|
||||
| location | String | This channel reports the location. |
|
||||
| installationName | String | This channel reports the installation name. |
|
||||
| installationId | Number | This channel reports the installation ID. |
|
||||
| nightControlTriggerChannel | trigger | This is a trigger channel that receives events. |
|
||||
| location | String | This channel reports the location. |
|
||||
| installationName | String | This channel reports the installation name. |
|
||||
| installationId | Number | This channel reports the installation ID. |
|
||||
| lowBattery | Switch | This channel reports if the battery level is low. |
|
||||
| nightControlTriggerChannel | trigger | This is a trigger channel that receives events. |
|
||||
|
||||
### Verisure DoorWindow Sensor
|
||||
|
||||
#### Configuration Options
|
||||
|
||||
* `deviceId` - Device Id
|
||||
* Sensor Id. Example 5A4C35FT (Note: Verisure ID, found in the Verisure App or My Pages or on the sensor itself)
|
||||
* Sensor Id. Example 5A4C35FT (Note: Verisure ID, found in the Verisure App or My Pages or on the sensor itself)
|
||||
|
||||
#### Channels
|
||||
|
||||
|
@ -263,7 +266,8 @@ The following channels are supported:
|
|||
| location | String | This channel reports the location of the device. |
|
||||
| installationName | String | This channel reports the installation name. |
|
||||
| installationId | Number | This channel reports the installation ID. |
|
||||
| doorWindowTriggerChannel | trigger | This is a trigger channel that receives events. |
|
||||
| lowBattery | Switch | This channel reports if the battery level is low. |
|
||||
| doorWindowTriggerChannel | trigger | This is a trigger channel that receives events. |
|
||||
|
||||
|
||||
### Verisure User Presence
|
||||
|
@ -271,7 +275,7 @@ The following channels are supported:
|
|||
#### Configuration Options
|
||||
|
||||
* `deviceId` - Device Id
|
||||
* Since User presence lacks a Verisure ID, it is constructed from the user's email address, where the '@' sign is removed, and the site id. The following naming convention is used for User presence on site id 123456789 for a user with email address test@gmail.com: 'uptestgmailcom123456789'. Installation ID can be found using DEBUG log settings.
|
||||
* Since User presence lacks a Verisure ID, it is constructed from the user's email address, where the '@' sign is removed, and the site id. The following naming convention is used for User presence on site id 123456789 for a user with email address test@gmail.com: 'uptestgmailcom123456789'. Installation ID can be found using DEBUG log settings.
|
||||
|
||||
#### Channels
|
||||
|
||||
|
@ -292,7 +296,7 @@ The following channels are supported:
|
|||
#### Configuration Options
|
||||
|
||||
* `deviceId` - Device Id
|
||||
* Since Broadband connection lacks a Verisure ID, the following naming convention is used for Broadband connection on site id 123456789: 'bc123456789'. Installation ID can be found using DEBUG log settings.
|
||||
* Since Broadband connection lacks a Verisure ID, the following naming convention is used for Broadband connection on site id 123456789: 'bc123456789'. Installation ID can be found using DEBUG log settings.
|
||||
|
||||
#### Channels
|
||||
|
||||
|
@ -310,33 +314,32 @@ The following channels are supported:
|
|||
#### Configuration Options
|
||||
|
||||
* `deviceId` - Device Id
|
||||
* Sensor Id. Example 5A4C35FT (Note: Verisure ID, found in the Verisure App or My Pages or on the sensor itself)
|
||||
* Sensor Id. Example 5A4C35FT (Note: Verisure ID, found in the Verisure App or My Pages or on the sensor itself)
|
||||
|
||||
#### Channels
|
||||
|
||||
The following channels are supported:
|
||||
|
||||
| Channel Type ID | Item Type | Description |
|
||||
|-----------------------------|--------------------|-------------------------------------------------------------------------------------|
|
||||
| countLatestDetection | Number | This channel reports the number of mice counts the latest detection during last 24. |
|
||||
| countLast24Hours | Number | This channel reports the total number of mice counts the last 24h. |
|
||||
|
||||
| Channel Type ID | Item Type | Description |
|
||||
|-----------------------------|--------------------|-------------------------------------------------------------------------------------|
|
||||
| countLatestDetection | Number | This channel reports the number of mice counts the latest detection during last 24. |
|
||||
| countLast24Hours | Number | This channel reports the total number of mice counts the last 24h. |
|
||||
| durationLatestDetection | Number:Time | This channel reports the detection duration in min of latest detection. |
|
||||
| durationLast24Hours | Number:Time | This channel reports the total detection duration in min for the last 24 hours. |
|
||||
| timestamp | DateTime | This channel reports time for the last mouse detection. |
|
||||
| temperature | Number:Temperature | This channel reports the current temperature. |
|
||||
| temperatureTimestamp | DateTime | This channel reports the time for the last temperature reading. |
|
||||
| durationLast24Hours | Number:Time | This channel reports the total detection duration in min for the last 24 hours. |
|
||||
| timestamp | DateTime | This channel reports time for the last mouse detection. |
|
||||
| temperature | Number:Temperature | This channel reports the current temperature. |
|
||||
| temperatureTimestamp | DateTime | This channel reports the time for the last temperature reading. |
|
||||
| location | String | This channel reports the location of the device. |
|
||||
| installationName | String | This channel reports the installation name. |
|
||||
| installationId | Number | This channel reports the installation ID. |
|
||||
| miceDetectionTriggerChannel | trigger | This is a trigger channel that receives events. |
|
||||
| miceDetectionTriggerChannel | trigger | This is a trigger channel that receives events. |
|
||||
|
||||
### Verisure Event Log
|
||||
|
||||
#### Configuration Options
|
||||
|
||||
* `deviceId` - Device Id
|
||||
* Since Event Log lacks a Verisure ID, the following naming convention is used for Event Log on site id 123456789: 'el123456789'. Installation ID can be found using DEBUG log settings.
|
||||
|
||||
* Since Event Log lacks a Verisure ID, the following naming convention is used for Event Log on site id 123456789: 'el123456789'. Installation ID can be found using DEBUG log settings.
|
||||
|
||||
#### Channels
|
||||
|
||||
|
@ -358,7 +361,7 @@ The following channels are supported:
|
|||
#### Configuration Options
|
||||
|
||||
* `deviceId` - Device Id
|
||||
* Sensor Id. Example 3B4C35FT (Note: Verisure ID, found in the Verisure App or My Pages or on the Gateway itself)
|
||||
* Sensor Id. Example 3B4C35FT (Note: Verisure ID, found in the Verisure App or My Pages or on the Gateway itself)
|
||||
|
||||
#### Channels
|
||||
|
||||
|
@ -382,7 +385,7 @@ The following channels are supported:
|
|||
To be able to get trigger events you need an active Event Log thing, you can either get it via auto-detection or create your own in a things-file.
|
||||
The following trigger events are defined per thing type:
|
||||
|
||||
| Event | Thing Type | Description |
|
||||
| Event Type | Thing Type | Description |
|
||||
|-------------------|---------------|------------------------------------------------------------|
|
||||
| LOCK | SmartLock | SmartLock has been locked. |
|
||||
| UNLOCK | SmartLock | SmartLock has been locked. |
|
||||
|
|
|
@ -27,6 +27,10 @@ Tap the paddle of your new HomeSeer switch to begin the inclusion process. This
|
|||
|
||||
Tap the paddle of your new HomeSeer switch to begin the exclusion process.
|
||||
|
||||
### General Usage Information
|
||||
|
||||
|
||||
|
||||
## Channels
|
||||
|
||||
The following table summarises the channels available for the WD-100 -:
|
||||
|
|
|
@ -0,0 +1,351 @@
|
|||
---
|
||||
layout: documentation
|
||||
title: FGWCEU-201 - ZWave
|
||||
---
|
||||
|
||||
{% include base.html %}
|
||||
|
||||
# FGWCEU-201 FIBARO Walli Double Wall Mounted Remote Controller
|
||||
This describes the Z-Wave device *FGWCEU-201*, manufactured by *[Fibargroup](http://www.fibaro.com/)* with the thing type UID of ```fibaro_fgwceu-201_00_000```.
|
||||
|
||||
The device is in the category of *Battery*, defining Batteries, Energy Storages.
|
||||
|
||||
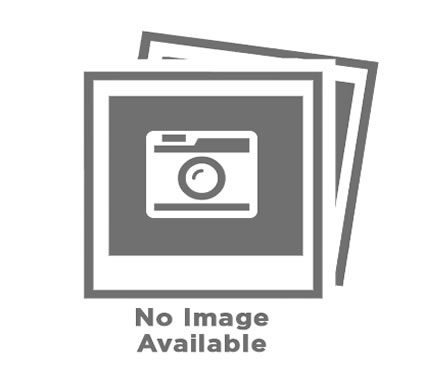
|
||||
|
||||
|
||||
The FGWCEU-201 supports routing. This allows the device to communicate using other routing enabled devices as intermediate routers. This device is unable to participate in the routing of data from other devices.
|
||||
|
||||
The FGWCEU-201 does not permanently listen for messages sent from the controller - it will periodically wake up automatically to check if the controller has messages to send, but will sleep most of the time to conserve battery life. Refer to the *Wakeup Information* section below for further information.
|
||||
|
||||
## Overview
|
||||
|
||||
FIBARO Walli Controller is a smart wall-mounted Z-Wave™ remote controller that can activate scenes or control other Z-Wave devices via associations.
|
||||
|
||||
Main features:
|
||||
• Can be used for controlling multiple types of devices, e.g. switches, dimmers, roller shutters.
|
||||
• Pre-set configurations allow to easily adjust operation for specific type of controlled devices.
|
||||
• Battery or VDC powered.
|
||||
• Equipped with a built-in temperature sensor.
|
||||
|
||||
|
||||
• Supports Z-Wave network Security Modes: S0 with AES-128 encryption and S2 Authenticated with PRNG-based encryption.
|
||||
• Works as a Z-Wave signal repeater when VDC powered (all non-battery operated devices within the network will act as repeaters to increase reliability of the network).
|
||||
• May be used with all devices certified with the Z-Wave Plus™ certificate and should be compatible with such devices produced by other manufacturers
|
||||
|
||||
### Inclusion Information
|
||||
|
||||
To add the device to the Z-Wave network manually:
|
||||
|
||||
1. Set the main controller in (Security/non-Security Mode) add mode (see the controller’s manual).
|
||||
2. Quickly, three times click one of the buttons.
|
||||
3. If you are adding in Security S2 Authenticated, input the underlined part of the DSK (label on the box).
|
||||
4. LED will start blinking yellow, wait for the adding process to end.
|
||||
5. Adding result will be confirmed by the Z-Wave controller’s message and the LED frame:
|
||||
• Green – successful (non-secure, S0, S2 non-authenticated),
|
||||
• Magenta – successful (Security S2 Authenticated),
|
||||
• Red – not successful.
|
||||
|
||||
### Exclusion Information
|
||||
|
||||
To remove the device from the Z-Wave network:
|
||||
|
||||
1. Set the main controller into remove mode (see the controller’s manual).
|
||||
2. Quickly, three times click, then press and hold one of the buttons for 12 seconds.
|
||||
3. Release the button when the device glows green.
|
||||
4. Quickly click the button to confirm.
|
||||
5. LED will start blinking yellow, wait for the removing process to end.
|
||||
6. Successful removing will be confirmed by the Z-Wave controller’s message and red LED colour
|
||||
|
||||
### Wakeup Information
|
||||
|
||||
The FGWCEU-201 does not permanently listen for messages sent from the controller - it will periodically wake up automatically to check if the controller has messages to send, but will sleep most of the time to conserve battery life. The wakeup period can be configured in the user interface - it is advisable not to make this too short as it will impact battery life - a reasonable compromise is 1 hour.
|
||||
|
||||
The wakeup period does not impact the devices ability to report events or sensor data. The device can be manually woken with a button press on the device as described below - note that triggering a device to send an event is not the same as a wakeup notification, and this will not allow the controller to communicate with the device.
|
||||
|
||||
|
||||
You can wake up the device manually using first menu position (white):
|
||||
|
||||
1. Quickly, three times click, then press and hold one of the buttons.
|
||||
|
||||
2. After 3 seconds LED ring signals adding status:
|
||||
• Blinking green – entering the menu (added as non-secure, S0, S2 non-authenticated)
|
||||
|
||||
|
||||
• Blinking magenta – entering the menu (added as Security S2 Authenticated)
|
||||
• Blinking red – entering the menu (not added to a Z-Wave network)
|
||||
|
||||
3. The LED ring will turn off for 3 seconds, then start signalling menu positions.
|
||||
|
||||
4. Release the button when device signals desired position with colour:
|
||||
• WHITE – wake up the device
|
||||
• GREEN – start learning mode (add/remove)
|
||||
• MAGENTA – test Z-Wave range
|
||||
• CYAN – show battery level
|
||||
» Green – 50-100%
|
||||
» Yellow – 16-49%
|
||||
» Red – 1-15%
|
||||
• YELLOW – reset to factory default
|
||||
|
||||
5. Quickly click the button to confirm.
|
||||
|
||||
### General Usage Information
|
||||
|
||||
Resetting to factory defaults
|
||||
|
||||
Reset procedure allows to restore the device back to its factory settings, which means all information about the Z-Wave controller and user configuration will be deleted.
|
||||
|
||||
Resetting the device is not the recommended way of removing the device from the Z-Wave network. Use reset procedure only if the primary controller is missing or inoperable.
|
||||
|
||||
1. Quickly, three times click, then press and hold one of the buttons for 21 seconds.
|
||||
2. Release the button when the device glows yellow.
|
||||
3. Quickly click the button to confirm.
|
||||
4. After a few seconds the device will be restarted, which is signalled with red LED colour.
|
||||
|
||||
## Channels
|
||||
|
||||
The following table summarises the channels available for the FGWCEU-201 -:
|
||||
|
||||
| Channel Name | Channel ID | Channel Type | Category | Item Type |
|
||||
|--------------|------------|--------------|----------|-----------|
|
||||
| Sensor (temperature) | sensor_temperature | sensor_temperature | Temperature | Number:Temperature |
|
||||
| Scene Number | scene_number | scene_number | | Number |
|
||||
| Battery Level | battery-level | system.battery_level | Battery | Number |
|
||||
|
||||
### Sensor (temperature)
|
||||
Indicates the current temperature.
|
||||
|
||||
The ```sensor_temperature``` channel is of type ```sensor_temperature``` and supports the ```Number:Temperature``` item and is in the ```Temperature``` category.
|
||||
|
||||
### Scene Number
|
||||
Triggers when a scene button is pressed.
|
||||
|
||||
The ```scene_number``` channel is of type ```scene_number``` and supports the ```Number``` item.
|
||||
This channel provides the scene, and the event as a decimal value in the form ```<scene>.<event>```. The scene number is set by the device, and the event is as follows -:
|
||||
|
||||
| Event ID | Event Description |
|
||||
|----------|--------------------|
|
||||
| 0 | Single key press |
|
||||
| 1 | Key released |
|
||||
| 2 | Key held down |
|
||||
| 3 | Double keypress |
|
||||
| 4 | Tripple keypress |
|
||||
| 5 | 4 x keypress |
|
||||
| 6 | 5 x keypress |
|
||||
|
||||
### Battery Level
|
||||
Represents the battery level as a percentage (0-100%). Bindings for things supporting battery level in a different format (e.g. 4 levels) should convert to a percentage to provide a consistent battery level reading.
|
||||
|
||||
The ```system.battery-level``` channel is of type ```system.battery-level``` and supports the ```Number``` item and is in the ```Battery``` category.
|
||||
This channel provides the battery level as a percentage and also reflects the low battery warning state. If the battery state is in low battery warning state, this will read 0%.
|
||||
|
||||
|
||||
## Device Configuration
|
||||
|
||||
The following table provides a summary of the 6 configuration parameters available in the FGWCEU-201.
|
||||
Detailed information on each parameter can be found in the sections below.
|
||||
|
||||
| Param | Name | Description |
|
||||
|-------|-------|-------------|
|
||||
| 13 | LED frame – brightness | This parameter allows to adjust the LED frame brightness |
|
||||
| 20 | Operation mode | This parameter defines operation of the device. Choose the mode depending on the type of the device you want to control remotely |
|
||||
| 150 | LED ring – first button | This parameter defines the colour of first button indicator (upper part of the LED ring) for indications using Indicator CC. |
|
||||
| 151 | LED ring – second button | This parameter defines the colour of second button indicator (lower part of the LED ring) for indications using Indicator CC. |
|
||||
| 152 | 1st button - double click value | This parameter defines value of Basic Set or Multilevel Set frame (depending on selected mode) sent to associated devices after double click. This parameter is not relevant for Scene Controller Mode |
|
||||
| 153 | 2nd button - double click value | This parameter defines value of Basic Set or Multilevel Set frame (depending on selected mode) sent to associated devices after double click. This parameter is used only in Double Button Mode. |
|
||||
| | Wakeup Interval | Sets the interval at which the device will accept commands from the controller |
|
||||
| | Wakeup Node | Sets the node ID of the device to receive the wakeup notifications |
|
||||
|
||||
### Parameter 13: LED frame – brightness
|
||||
|
||||
This parameter allows to adjust the LED frame brightness
|
||||
0 – LED disabled
|
||||
1-100 (1-100% brightness)
|
||||
Values in the range 0 to 100 may be set.
|
||||
|
||||
The manufacturer defined default value is ```100```.
|
||||
|
||||
This parameter has the configuration ID ```config_13_1``` and is of type ```INTEGER```.
|
||||
|
||||
|
||||
### Parameter 20: Operation mode
|
||||
|
||||
This parameter defines operation of the device. Choose the mode depending on the type of the device you want to control remotely
|
||||
0 – scene controller mode
|
||||
1 – double button mode
|
||||
2 – single button mode
|
||||
3 – switch controller mode
|
||||
4 – dimmer / roller shutter controller mode
|
||||
5 – roller shutter controller mode (step-by-step)
|
||||
6 – venetian blinds controller mode (step-by-step)
|
||||
Values in the range 0 to 6 may be set.
|
||||
|
||||
The manufacturer defined default value is ```0```.
|
||||
|
||||
This parameter has the configuration ID ```config_20_1``` and is of type ```INTEGER```.
|
||||
|
||||
|
||||
### Parameter 150: LED ring – first button
|
||||
|
||||
This parameter defines the colour of first button indicator (upper part of the LED ring) for indications using Indicator CC.
|
||||
0 – LED disabled
|
||||
1 – White
|
||||
2 – Red
|
||||
3 – Green
|
||||
4 – Blue
|
||||
5 – Yellow
|
||||
6 – Cyan
|
||||
7 – Magenta
|
||||
8 – Blinking red-white-blue
|
||||
Values in the range 0 to 8 may be set.
|
||||
|
||||
The manufacturer defined default value is ```1```.
|
||||
|
||||
This parameter has the configuration ID ```config_150_2``` and is of type ```INTEGER```.
|
||||
|
||||
|
||||
### Parameter 151: LED ring – second button
|
||||
|
||||
This parameter defines the colour of second button indicator (lower part of the LED ring) for indications using Indicator CC.
|
||||
0 – LED disabled
|
||||
1 – White
|
||||
2 – Red
|
||||
3 – Green
|
||||
4 – Blue
|
||||
5 – Yellow
|
||||
6 – Cyan
|
||||
7 – Magenta
|
||||
8 – Blinking red-white-blue
|
||||
Values in the range 0 to 8 may be set.
|
||||
|
||||
The manufacturer defined default value is ```1```.
|
||||
|
||||
This parameter has the configuration ID ```config_151_2``` and is of type ```INTEGER```.
|
||||
|
||||
|
||||
### Parameter 152: 1st button - double click value
|
||||
|
||||
This parameter defines value of Basic Set or Multilevel Set frame (depending on selected mode) sent to associated devices after double click. This parameter is not relevant for Scene Controller Mode
|
||||
Available values 0-99 or 255
|
||||
Values in the range 0 to 255 may be set.
|
||||
|
||||
The manufacturer defined default value is ```99```.
|
||||
|
||||
This parameter has the configuration ID ```config_152_2``` and is of type ```INTEGER```.
|
||||
|
||||
|
||||
### Parameter 153: 2nd button - double click value
|
||||
|
||||
This parameter defines value of Basic Set or Multilevel Set frame (depending on selected mode) sent to associated devices after double click. This parameter is used only in Double Button Mode.
|
||||
Available values 0-99 or 255
|
||||
Values in the range 0 to 255 may be set.
|
||||
|
||||
The manufacturer defined default value is ```99```.
|
||||
|
||||
This parameter has the configuration ID ```config_153_2``` and is of type ```INTEGER```.
|
||||
|
||||
### Wakeup Interval
|
||||
|
||||
The wakeup interval sets the period at which the device will listen for messages from the controller. This is required for battery devices that sleep most of the time in order to conserve battery life. The device will wake up at this interval and send a message to the controller to tell it that it can accept messages - after a few seconds, it will go back to sleep if there is no further communications.
|
||||
|
||||
This setting is defined in *seconds*. It is advisable not to set this interval too short or it could impact battery life. A period of 1 hour (3600 seconds) is suitable in most instances.
|
||||
|
||||
Note that this setting does not affect the devices ability to send sensor data, or notification events.
|
||||
|
||||
This parameter has the configuration ID ```wakeup_interval``` and is of type ```INTEGER```.
|
||||
|
||||
### Wakeup Node
|
||||
|
||||
When sleeping devices wake up, they send a notification to a listening device. Normally, this device is the network controller, and normally the controller will set this automatically to its own address.
|
||||
In the event that the network contains multiple controllers, it may be necessary to configure this to a node that is not the main controller. This is an advanced setting and should not be changed without a full understanding of the impact.
|
||||
|
||||
This parameter has the configuration ID ```wakeup_node``` and is of type ```INTEGER```.
|
||||
|
||||
|
||||
## Association Groups
|
||||
|
||||
Association groups allow the device to send unsolicited reports to the controller, or other devices in the network. Using association groups can allow you to eliminate polling, providing instant feedback of a device state change without unnecessary network traffic.
|
||||
|
||||
The FGWCEU-201 supports 7 association groups.
|
||||
|
||||
### Group 1: Lifeline
|
||||
|
||||
The Lifeline association group reports device status to a hub and is not designed to control other devices directly. When using the Lineline group with a hub, in most cases, only the lifeline group will need to be configured and normally the hub will perform this automatically during the device initialisation.
|
||||
Reports the device status and allows for assigning single device only (main controller by default)
|
||||
|
||||
Association group 1 supports 1 node.
|
||||
|
||||
### Group 2: On/Off (1)
|
||||
|
||||
Used to turn the associated devices on/off.
|
||||
|
||||
Association group 2 supports 5 nodes.
|
||||
|
||||
### Group 3: Dimmer (1)
|
||||
|
||||
Used to change level of associated devices
|
||||
|
||||
Association group 3 supports 5 nodes.
|
||||
|
||||
### Group 4: On/Off (2)
|
||||
|
||||
Used to turn the associated devices on/off
|
||||
|
||||
Association group 4 supports 5 nodes.
|
||||
|
||||
### Group 5: Dimmer (2)
|
||||
|
||||
Used to change level of associated devices
|
||||
|
||||
Association group 5 supports 5 nodes.
|
||||
|
||||
### Group 6: Multidevice
|
||||
|
||||
Used to control different type of devices
|
||||
|
||||
Association group 6 supports 5 nodes.
|
||||
|
||||
### Group 7: Slats
|
||||
|
||||
Used to move slats of Venetian blinds.
|
||||
|
||||
Association group 7 supports 5 nodes.
|
||||
|
||||
## Technical Information
|
||||
|
||||
### Endpoints
|
||||
|
||||
#### Endpoint 0
|
||||
|
||||
| Command Class | Comment |
|
||||
|---------------|---------|
|
||||
| COMMAND_CLASS_NO_OPERATION_V1| |
|
||||
| COMMAND_CLASS_APPLICATION_STATUS_V1| |
|
||||
| COMMAND_CLASS_SENSOR_MULTILEVEL_V10| |
|
||||
| COMMAND_CLASS_TRANSPORT_SERVICE_V1| |
|
||||
| COMMAND_CLASS_ASSOCIATION_GRP_INFO_V1| |
|
||||
| COMMAND_CLASS_DEVICE_RESET_LOCALLY_V1| |
|
||||
| COMMAND_CLASS_CENTRAL_SCENE_V3| |
|
||||
| COMMAND_CLASS_ZWAVEPLUS_INFO_V1| |
|
||||
| COMMAND_CLASS_SUPERVISION_V1| |
|
||||
| COMMAND_CLASS_CONFIGURATION_V1| |
|
||||
| COMMAND_CLASS_MANUFACTURER_SPECIFIC_V1| |
|
||||
| COMMAND_CLASS_POWERLEVEL_V1| |
|
||||
| COMMAND_CLASS_PROTECTION_V1| |
|
||||
| COMMAND_CLASS_FIRMWARE_UPDATE_MD_V1| |
|
||||
| COMMAND_CLASS_BATTERY_V1| |
|
||||
| COMMAND_CLASS_WAKE_UP_V2| |
|
||||
| COMMAND_CLASS_ASSOCIATION_V2| |
|
||||
| COMMAND_CLASS_VERSION_V2| |
|
||||
| COMMAND_CLASS_INDICATOR_V3| |
|
||||
| COMMAND_CLASS_MULTI_CHANNEL_ASSOCIATION_V3| |
|
||||
| COMMAND_CLASS_SECURITY_V1| |
|
||||
| COMMAND_CLASS_SECURITY_2_V1| |
|
||||
|
||||
### Documentation Links
|
||||
|
||||
* [Fibaro Walli Controller manual EN](https://opensmarthouse.org/zwavedatabase/1383/reference/FGWCEU-201-T-EN-1.1.pdf)
|
||||
|
||||
---
|
||||
|
||||
Did you spot an error in the above definition or want to improve the content?
|
||||
You can [contribute to the database here](https://opensmarthouse.org/zwavedatabase/1383).
|
|
@ -103,7 +103,7 @@ Detailed information on each parameter can be found in the sections below.
|
|||
|
||||
| Param | Name | Description |
|
||||
|-------|-------|-------------|
|
||||
| 1 | Twin Assist | Helps you to lock and unlock your door lock. |
|
||||
| 1 | Twist Assist | Helps you to lock and unlock your door lock. |
|
||||
| 2 | Hold and Release | After a unlock operation the lock holds the lock, so the door can be opened |
|
||||
| 3 | Block to block | The lock will run the motor until it hits resistance. |
|
||||
| 4 | BLE Temporary Allowed | The BLE is enabled for a number of seconds. |
|
||||
|
@ -111,7 +111,7 @@ Detailed information on each parameter can be found in the sections below.
|
|||
| 6 | Autolock | Lock after an unlock.Value is delay in sec before lock is locked again. |
|
||||
| | Lock Timeout | Sets the time after which the door will auto lock |
|
||||
|
||||
### Parameter 1: Twin Assist
|
||||
### Parameter 1: Twist Assist
|
||||
|
||||
Helps you to lock and unlock your door lock.
|
||||
0 -> disabled
|
||||
|
@ -135,7 +135,7 @@ After a unlock operation the lock holds the lock, so the door can be opened
|
|||
0 -> disabled
|
||||
|
||||
1 to 2147483647 -> enabled no. of seconds
|
||||
The following option values may be configured, in addition to values in the range 0 to 2147483647 -:
|
||||
The following option values may be configured -:
|
||||
|
||||
| Value | Description |
|
||||
|--------|-------------|
|
||||
|
|
|
@ -0,0 +1,252 @@
|
|||
---
|
||||
layout: documentation
|
||||
title: zen34v1.01 - ZWave
|
||||
---
|
||||
|
||||
{% include base.html %}
|
||||
|
||||
# zen34v1.01 Remote Switch
|
||||
This describes the Z-Wave device *zen34v1.01*, manufactured by *[Zooz](http://www.getzooz.com/)* with the thing type UID of ```zooz_zen34v101_00_000```.
|
||||
|
||||
The device is in the category of *Remote Control*, defining Any portable or hand-held device that controls the status of something, e.g. remote control, keyfob etc..
|
||||
|
||||
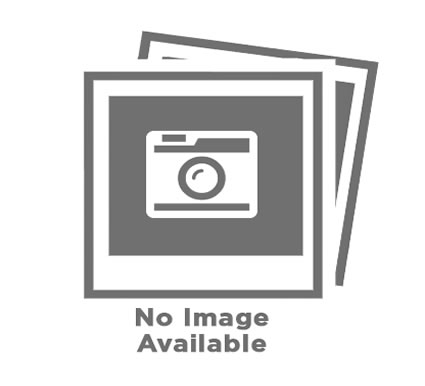
|
||||
|
||||
|
||||
The zen34v1.01 supports routing. This allows the device to communicate using other routing enabled devices as intermediate routers. This device is unable to participate in the routing of data from other devices.
|
||||
|
||||
The zen34v1.01 does not permanently listen for messages sent from the controller - it will periodically wake up automatically to check if the controller has messages to send, but will sleep most of the time to conserve battery life. Refer to the *Wakeup Information* section below for further information.
|
||||
|
||||
## Overview
|
||||
|
||||
* Control other Z-Wave devices and enable scenes with a click of a button
|
||||
|
||||
* Classic paddle switch look and size + magnetic base
|
||||
|
||||
* Install in your electrical switch box or mount anywhere on a wall with just adhesive tape
|
||||
|
||||
* The latest 700 chip with S2 and SmartStart support
|
||||
|
||||
* Powered by batteries, no wires needed
|
||||
|
||||
### Inclusion Information
|
||||
|
||||
Put the openHAB Z-Wave binding into inclusion mode and click the upper paddle 6 times quickly. The LED indicator will blink blue during the process and light up green once added successfully. It will light up red if failed.
|
||||
|
||||
### Exclusion Information
|
||||
|
||||
Put the openHAB Z-Wave binding into exclusion mode and click the lower paddle 6 times quickly.
|
||||
|
||||
### Wakeup Information
|
||||
|
||||
The zen34v1.01 does not permanently listen for messages sent from the controller - it will periodically wake up automatically to check if the controller has messages to send, but will sleep most of the time to conserve battery life. The wakeup period can be configured in the user interface - it is advisable not to make this too short as it will impact battery life - a reasonable compromise is 1 hour.
|
||||
|
||||
The wakeup period does not impact the devices ability to report events or sensor data. The device can be manually woken with a button press on the device as described below - note that triggering a device to send an event is not the same as a wakeup notification, and this will not allow the controller to communicate with the device.
|
||||
|
||||
|
||||
|
||||
|
||||
### General Usage Information
|
||||
|
||||
The LED indication will light up any time a paddle is pressed. It will blink once for each tap. You can adjust the LED indicator color and operation mode in the configuration parameters.
|
||||
|
||||
Upper Paddle
|
||||
|
||||
TAP x 1 for Scene 1, pressed (or ON basic for associations)
|
||||
|
||||
TAP x 2, 3, 4, or 5 for Scene 1 multi-tap
|
||||
|
||||
HOLD for Scene 1, held (or multilevel start level change, add brightness)
|
||||
|
||||
RELEASE for Scene 1, released (or multilevel stop level change)
|
||||
|
||||
Lower Paddle
|
||||
|
||||
TAP x 1 for Scene 2, pressed (or OFF basic for associations)
|
||||
|
||||
TAP x 2, 3, 4, or 5 for Scene 2 multi-tap
|
||||
|
||||
HOLD for Scene 2, held (or multilevel start level change, dim the light)
|
||||
|
||||
RELEASE for Scene 2, released (or multilevel stop level change)
|
||||
|
||||
## Channels
|
||||
|
||||
The following table summarises the channels available for the zen34v1.01 -:
|
||||
|
||||
| Channel Name | Channel ID | Channel Type | Category | Item Type |
|
||||
|--------------|------------|--------------|----------|-----------|
|
||||
| Scene Number | scene_number | scene_number | | Number |
|
||||
| Battery Level | battery-level | system.battery_level | Battery | Number |
|
||||
|
||||
### Scene Number
|
||||
Triggers when a scene button is pressed.
|
||||
|
||||
The ```scene_number``` channel is of type ```scene_number``` and supports the ```Number``` item.
|
||||
This channel provides the scene, and the event as a decimal value in the form ```<scene>.<event>```. The scene number is set by the device, and the event is as follows -:
|
||||
|
||||
| Event ID | Event Description |
|
||||
|----------|--------------------|
|
||||
| 0 | Single key press |
|
||||
| 1 | Key released |
|
||||
| 2 | Key held down |
|
||||
| 3 | Double keypress |
|
||||
| 4 | Tripple keypress |
|
||||
| 5 | 4 x keypress |
|
||||
| 6 | 5 x keypress |
|
||||
|
||||
### Battery Level
|
||||
Represents the battery level as a percentage (0-100%). Bindings for things supporting battery level in a different format (e.g. 4 levels) should convert to a percentage to provide a consistent battery level reading.
|
||||
|
||||
The ```system.battery-level``` channel is of type ```system.battery-level``` and supports the ```Number``` item and is in the ```Battery``` category.
|
||||
This channel provides the battery level as a percentage and also reflects the low battery warning state. If the battery state is in low battery warning state, this will read 0%.
|
||||
|
||||
|
||||
## Device Configuration
|
||||
|
||||
The following table provides a summary of the 3 configuration parameters available in the zen34v1.01.
|
||||
Detailed information on each parameter can be found in the sections below.
|
||||
|
||||
| Param | Name | Description |
|
||||
|-------|-------|-------------|
|
||||
| 1 | LED indication mode | |
|
||||
| 2 | Upper paddle indicator color | |
|
||||
| 3 | Lower paddle indicator color | |
|
||||
| | Wakeup Interval | Sets the interval at which the device will accept commands from the controller |
|
||||
| | Wakeup Node | Sets the node ID of the device to receive the wakeup notifications |
|
||||
|
||||
### Parameter 1: LED indication mode
|
||||
|
||||
|
||||
|
||||
The following option values may be configured, in addition to values in the range 0 to 2 -:
|
||||
|
||||
| Value | Description |
|
||||
|--------|-------------|
|
||||
| 0 | LED always OFF |
|
||||
| 1 | LED ON when button pressed |
|
||||
| 2 | LED always ON (color from #2) |
|
||||
| 3 | LED always ON (color from #3) |
|
||||
|
||||
The manufacturer defined default value is ```1``` (LED ON when button pressed).
|
||||
|
||||
This parameter has the configuration ID ```config_1_1``` and is of type ```INTEGER```.
|
||||
|
||||
|
||||
### Parameter 2: Upper paddle indicator color
|
||||
|
||||
|
||||
|
||||
The following option values may be configured, in addition to values in the range 0 to 6 -:
|
||||
|
||||
| Value | Description |
|
||||
|--------|-------------|
|
||||
| 0 | White |
|
||||
| 1 | Blue |
|
||||
| 2 | Green |
|
||||
| 3 | Red |
|
||||
| 4 | Magenta |
|
||||
| 5 | Yellow |
|
||||
| 6 | Cyan |
|
||||
|
||||
The manufacturer defined default value is ```1``` (Blue).
|
||||
|
||||
This parameter has the configuration ID ```config_2_1``` and is of type ```INTEGER```.
|
||||
|
||||
|
||||
### Parameter 3: Lower paddle indicator color
|
||||
|
||||
|
||||
|
||||
The following option values may be configured, in addition to values in the range 0 to 6 -:
|
||||
|
||||
| Value | Description |
|
||||
|--------|-------------|
|
||||
| 0 | White |
|
||||
| 1 | Blue |
|
||||
| 2 | Green |
|
||||
| 3 | Red |
|
||||
| 4 | Magenta |
|
||||
| 5 | Yellow |
|
||||
| 6 | Cyan |
|
||||
|
||||
The manufacturer defined default value is ```1``` (Blue).
|
||||
|
||||
This parameter has the configuration ID ```config_3_1``` and is of type ```INTEGER```.
|
||||
|
||||
### Wakeup Interval
|
||||
|
||||
The wakeup interval sets the period at which the device will listen for messages from the controller. This is required for battery devices that sleep most of the time in order to conserve battery life. The device will wake up at this interval and send a message to the controller to tell it that it can accept messages - after a few seconds, it will go back to sleep if there is no further communications.
|
||||
|
||||
This setting is defined in *seconds*. It is advisable not to set this interval too short or it could impact battery life. A period of 1 hour (3600 seconds) is suitable in most instances.
|
||||
|
||||
Note that this setting does not affect the devices ability to send sensor data, or notification events.
|
||||
|
||||
This parameter has the configuration ID ```wakeup_interval``` and is of type ```INTEGER```.
|
||||
|
||||
### Wakeup Node
|
||||
|
||||
When sleeping devices wake up, they send a notification to a listening device. Normally, this device is the network controller, and normally the controller will set this automatically to its own address.
|
||||
In the event that the network contains multiple controllers, it may be necessary to configure this to a node that is not the main controller. This is an advanced setting and should not be changed without a full understanding of the impact.
|
||||
|
||||
This parameter has the configuration ID ```wakeup_node``` and is of type ```INTEGER```.
|
||||
|
||||
|
||||
## Association Groups
|
||||
|
||||
Association groups allow the device to send unsolicited reports to the controller, or other devices in the network. Using association groups can allow you to eliminate polling, providing instant feedback of a device state change without unnecessary network traffic.
|
||||
|
||||
The zen34v1.01 supports 3 association groups.
|
||||
|
||||
### Group 1: Lifeline
|
||||
|
||||
The Lifeline association group reports device status to a hub and is not designed to control other devices directly. When using the Lineline group with a hub, in most cases, only the lifeline group will need to be configured and normally the hub will perform this automatically during the device initialisation.
|
||||
|
||||
Association group 1 supports 1 node.
|
||||
|
||||
### Group 2: On/Off
|
||||
|
||||
1-tap for on.off control
|
||||
|
||||
Association group 2 supports 5 nodes.
|
||||
|
||||
### Group 3: dimming
|
||||
|
||||
Paddle held/released for dimming
|
||||
|
||||
Association group 3 supports 5 nodes.
|
||||
|
||||
## Technical Information
|
||||
|
||||
### Endpoints
|
||||
|
||||
#### Endpoint 0
|
||||
|
||||
| Command Class | Comment |
|
||||
|---------------|---------|
|
||||
| COMMAND_CLASS_NO_OPERATION_V1| |
|
||||
| COMMAND_CLASS_TRANSPORT_SERVICE_V1| |
|
||||
| COMMAND_CLASS_ASSOCIATION_GRP_INFO_V1| |
|
||||
| COMMAND_CLASS_DEVICE_RESET_LOCALLY_V1| |
|
||||
| COMMAND_CLASS_CENTRAL_SCENE_V3| |
|
||||
| COMMAND_CLASS_ZWAVEPLUS_INFO_V1| |
|
||||
| COMMAND_CLASS_SUPERVISION_V1| |
|
||||
| COMMAND_CLASS_CONFIGURATION_V1| |
|
||||
| COMMAND_CLASS_MANUFACTURER_SPECIFIC_V1| |
|
||||
| COMMAND_CLASS_POWERLEVEL_V1| |
|
||||
| COMMAND_CLASS_FIRMWARE_UPDATE_MD_V1| |
|
||||
| COMMAND_CLASS_BATTERY_V1| |
|
||||
| COMMAND_CLASS_WAKE_UP_V2| |
|
||||
| COMMAND_CLASS_ASSOCIATION_V2| |
|
||||
| COMMAND_CLASS_VERSION_V2| |
|
||||
| COMMAND_CLASS_MULTI_CHANNEL_ASSOCIATION_V3| |
|
||||
| COMMAND_CLASS_SECURITY_2_V1| |
|
||||
|
||||
### Documentation Links
|
||||
|
||||
* [Manual](https://opensmarthouse.org/zwavedatabase/1382/reference/zooz-700-series-remote-switch-zen34-manual.pdf)
|
||||
|
||||
---
|
||||
|
||||
Did you spot an error in the above definition or want to improve the content?
|
||||
You can [contribute to the database here](https://opensmarthouse.org/zwavedatabase/1382).
|
|
@ -160,6 +160,7 @@
|
|||
<channel id="installationName" typeId="installationName"/>
|
||||
<channel id="installationId" typeId="installationId"/>
|
||||
<channel id="smokeDetectorTriggerChannel" typeId="triggerChannel"/>
|
||||
<channel id="lowBattery" typeId="system.low-battery"/>
|
||||
</channels>
|
||||
|
||||
<properties>
|
||||
|
@ -224,6 +225,7 @@
|
|||
<channel id="installationName" typeId="installationName"/>
|
||||
<channel id="installationId" typeId="installationId"/>
|
||||
<channel id="sirenTriggerChannel" typeId="triggerChannel"/>
|
||||
<channel id="lowBattery" typeId="system.low-battery"/>
|
||||
</channels>
|
||||
|
||||
<properties>
|
||||
|
@ -256,6 +258,7 @@
|
|||
<channel id="installationName" typeId="installationName"/>
|
||||
<channel id="installationId" typeId="installationId"/>
|
||||
<channel id="nightControlTriggerChannel" typeId="triggerChannel"/>
|
||||
<channel id="lowBattery" typeId="system.low-battery"/>
|
||||
</channels>
|
||||
|
||||
<properties>
|
||||
|
@ -288,6 +291,7 @@
|
|||
<channel id="installationName" typeId="installationName"/>
|
||||
<channel id="installationId" typeId="installationId"/>
|
||||
<channel id="doorWindowTriggerChannel" typeId="triggerChannel"/>
|
||||
<channel id="lowBattery" typeId="system.low-battery"/>
|
||||
</channels>
|
||||
|
||||
<properties>
|
||||
|
|
|
@ -8,7 +8,7 @@
|
|||
<thing-type id="dragontech_wd100_00_000" listed="false">
|
||||
<label>WD-100 Wall Dimmer Switch</label>
|
||||
<description><![CDATA[
|
||||
Wall Dimmer Switch<br /> <h1>Overview</h1><p>HS-WD100+ is a Z-Wave wall dimmer that’s designed for wireless on-off-dim control of connected dimmable lighting loads. </p> <br /> <h2>Inclusion Information</h2><p>Tap the paddle of your new HomeSeer switch to begin the inclusion process. This will take a few moments to complete.</p> <br /> <h2>Exclusion Information</h2><p>Tap the paddle of your new HomeSeer switch to begin the exclusion process. </p>
|
||||
Wall Dimmer Switch<br /> <h1>Overview</h1><p>HS-WD100+ is a Z-Wave wall dimmer that’s designed for wireless on-off-dim control of connected dimmable lighting loads. </p> <br /> <h2>Inclusion Information</h2><p>Tap the paddle of your new HomeSeer switch to begin the inclusion process. This will take a few moments to complete.</p> <br /> <h2>Exclusion Information</h2><p>Tap the paddle of your new HomeSeer switch to begin the exclusion process. </p> <br /> <h2>Wakeup Information</h2><p><br /></p>
|
||||
]]></description>
|
||||
<category>WallSwitch</category>
|
||||
|
||||
|
@ -34,7 +34,7 @@ Wall Dimmer Switch<br /> <h1>Overview</h1><p>HS-WD100+ is a Z-Wave wall dimmer t
|
|||
<property name="vendor">Dragon Tech Industrial, Ltd.</property>
|
||||
<property name="modelId">WD-100</property>
|
||||
<property name="manufacturerId">0184</property>
|
||||
<property name="manufacturerRef">4447:3034,4744:3032</property>
|
||||
<property name="manufacturerRef">4447:3034</property>
|
||||
<property name="dbReference">243</property>
|
||||
<property name="defaultAssociations">1</property>
|
||||
</properties>
|
||||
|
|
|
@ -1,899 +0,0 @@
|
|||
<?xml version="1.0" encoding="UTF-8"?>
|
||||
<thing:thing-descriptions bindingId="zwave"
|
||||
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
|
||||
xmlns:thing="https://openhab.org/schemas/thing-description/v1.0.0"
|
||||
xsi:schemaLocation="https://openhab.org/schemas/thing-description/v1.0.0
|
||||
https://openhab.org/schemas/thing-description/v1.0.0">
|
||||
|
||||
<thing-type id="fibaro_fgrgbw442_00_000" listed="false">
|
||||
<label>FGRGBW-442 RGBW CONTROLLER 2</label>
|
||||
<description><![CDATA[
|
||||
RGBW CONTROLLER 2<br /> <h1>Overview</h1><p>FIBARO RGBW Controller 2 uses PWM output signal, allowing it to control LED, RGB, RGBW strips, halogen lights and other resistive loads. It can also measure active power and energy consumed by the load. Controlled devices may be powered by 12 or 24V DC.</p> <p>Inputs support momentary/toggle switches and 0-10V analog sensors, like temperature sensors, humidity sensors, light sensors etc</p> <br /> <h2>Inclusion Information</h2><p>Quickly, three times click the service button.</p> <br /> <h2>Exclusion Information</h2><p>Quickly, three times click the service button.</p> <br /> <h2>Wakeup Information</h2><p><br /></p>
|
||||
]]></description>
|
||||
<category>LightBulb</category>
|
||||
|
||||
<!-- CHANNEL DEFINITIONS -->
|
||||
<channels>
|
||||
<channel id="switch_dimmer" typeId="switch_dimmer">
|
||||
<label>Dimmer</label>
|
||||
<properties>
|
||||
<property name="binding:*:PercentType">COMMAND_CLASS_SWITCH_MULTILEVEL</property>
|
||||
<property name="binding:Command:OnOffType">COMMAND_CLASS_SWITCH_MULTILEVEL</property>
|
||||
</properties>
|
||||
</channel>
|
||||
<channel id="sensor_voltage" typeId="sensor_voltage">
|
||||
<label>Sensor (voltage)</label>
|
||||
<properties>
|
||||
<property name="binding:*:DecimalType">COMMAND_CLASS_SENSOR_MULTILEVEL;type=VOLTAGE</property>
|
||||
</properties>
|
||||
</channel>
|
||||
<channel id="meter_watts" typeId="meter_watts">
|
||||
<label>Electric meter (watts)</label>
|
||||
<properties>
|
||||
<property name="binding:*:DecimalType">COMMAND_CLASS_METER;type=E_W</property>
|
||||
</properties>
|
||||
</channel>
|
||||
<channel id="meter_kwh" typeId="meter_kwh">
|
||||
<label>Electric meter (kWh)</label>
|
||||
<properties>
|
||||
<property name="binding:*:DecimalType">COMMAND_CLASS_METER;type=E_KWh</property>
|
||||
</properties>
|
||||
</channel>
|
||||
<channel id="color_color" typeId="color_color">
|
||||
<label>Color Control</label>
|
||||
<properties>
|
||||
<property name="binding:*:HSBType">COMMAND_CLASS_SWITCH_COLOR</property>
|
||||
<property name="binding:*:PercentType">COMMAND_CLASS_SWITCH_MULTILEVEL</property>
|
||||
<property name="binding:Command:OnOffType">COMMAND_CLASS_SWITCH_MULTILEVEL</property>
|
||||
,COMMAND_CLASS_BASIC </properties>
|
||||
</channel>
|
||||
<channel id="color_temperature" typeId="color_temperature">
|
||||
<label>Color Temperature</label>
|
||||
<properties>
|
||||
<property name="binding:*:PercentType">COMMAND_CLASS_SWITCH_COLOR;colorMode=DIFF_WHITE</property>
|
||||
<property name="binding:Command:OnOffType">COMMAND_CLASS_SWITCH_MULTILEVEL</property>
|
||||
,COMMAND_CLASS_BASIC </properties>
|
||||
</channel>
|
||||
<channel id="scene_number" typeId="scene_number">
|
||||
<label>Scene Number</label>
|
||||
<properties>
|
||||
<property name="binding:*:DecimalType">COMMAND_CLASS_CENTRAL_SCENE</property>
|
||||
</properties>
|
||||
</channel>
|
||||
<channel id="config_decimal_param157" typeId="fibaro_fgrgbw442_00_000_config_decimal_param157">
|
||||
<label>Start programmed sequence</label>
|
||||
<properties>
|
||||
<property name="binding:*:DecimalType">COMMAND_CLASS_CONFIGURATION;parameter=157</property>
|
||||
</properties>
|
||||
</channel>
|
||||
<channel id="alarm_power" typeId="alarm_power">
|
||||
<label>Alarm (power)</label>
|
||||
<properties>
|
||||
<property name="binding:*:OnOffType">COMMAND_CLASS_ALARM;type=POWER_MANAGEMENT</property>
|
||||
</properties>
|
||||
</channel>
|
||||
<channel id="alarm_system" typeId="alarm_system">
|
||||
<label>Alarm (system)</label>
|
||||
<properties>
|
||||
<property name="binding:*:OnOffType">COMMAND_CLASS_ALARM;type=SYSTEM</property>
|
||||
</properties>
|
||||
</channel>
|
||||
<channel id="switch_dimmer1" typeId="switch_dimmer">
|
||||
<label>Dimmer 1</label>
|
||||
<properties>
|
||||
<property name="binding:*:PercentType">COMMAND_CLASS_SWITCH_MULTILEVEL:1,COMMAND_CLASS_BASIC:1</property>
|
||||
<property name="binding:Command:OnOffType">COMMAND_CLASS_SWITCH_MULTILEVEL:1</property>
|
||||
</properties>
|
||||
</channel>
|
||||
<channel id="meter_watts1" typeId="meter_watts">
|
||||
<label>Electric meter (watts) 1</label>
|
||||
<properties>
|
||||
<property name="binding:*:DecimalType">COMMAND_CLASS_METER:1;type=E_W</property>
|
||||
</properties>
|
||||
</channel>
|
||||
<channel id="meter_kwh1" typeId="meter_kwh">
|
||||
<label>Electric meter (kWh) 1</label>
|
||||
<properties>
|
||||
<property name="binding:*:DecimalType">COMMAND_CLASS_METER:1;type=E_KWh</property>
|
||||
</properties>
|
||||
</channel>
|
||||
<channel id="color_color1" typeId="color_color">
|
||||
<label>Color Control 1</label>
|
||||
<properties>
|
||||
<property name="binding:*:HSBType">COMMAND_CLASS_SWITCH_COLOR:1;colorMode=RGB</property>
|
||||
<property name="binding:*:PercentType">COMMAND_CLASS_SWITCH_MULTILEVEL:1</property>
|
||||
<property name="binding:Command:OnOffType">COMMAND_CLASS_SWITCH_MULTILEVEL:1</property>
|
||||
</properties>
|
||||
</channel>
|
||||
<channel id="color_temperature1" typeId="color_temperature">
|
||||
<label>Color Temperature 1</label>
|
||||
<properties>
|
||||
<property name="binding:*:PercentType">COMMAND_CLASS_SWITCH_COLOR:1;colorMode=DIFF_WHITE</property>
|
||||
<property name="binding:Command:OnOffType">COMMAND_CLASS_SWITCH_MULTILEVEL:1</property>
|
||||
</properties>
|
||||
</channel>
|
||||
<channel id="alarm_power1" typeId="alarm_power">
|
||||
<label>Alarm (power) 1</label>
|
||||
<properties>
|
||||
<property name="binding:*:OnOffType">COMMAND_CLASS_ALARM:1;type=POWER_MANAGEMENT</property>
|
||||
</properties>
|
||||
</channel>
|
||||
<channel id="alarm_system1" typeId="alarm_system">
|
||||
<label>Alarm (system) 1</label>
|
||||
<properties>
|
||||
<property name="binding:*:OnOffType">COMMAND_CLASS_ALARM:1;type=SYSTEM</property>
|
||||
</properties>
|
||||
</channel>
|
||||
<channel id="switch_dimmer2" typeId="switch_dimmer">
|
||||
<label>Dimmer 2</label>
|
||||
<properties>
|
||||
<property name="binding:*:PercentType">COMMAND_CLASS_SWITCH_MULTILEVEL:2,COMMAND_CLASS_BASIC:2</property>
|
||||
<property name="binding:Command:OnOffType">COMMAND_CLASS_SWITCH_MULTILEVEL:2</property>
|
||||
</properties>
|
||||
</channel>
|
||||
<channel id="switch_dimmer3" typeId="switch_dimmer">
|
||||
<label>Dimmer 3</label>
|
||||
<properties>
|
||||
<property name="binding:*:PercentType">COMMAND_CLASS_SWITCH_MULTILEVEL:3,COMMAND_CLASS_BASIC:3</property>
|
||||
<property name="binding:Command:OnOffType">COMMAND_CLASS_SWITCH_MULTILEVEL:3</property>
|
||||
</properties>
|
||||
</channel>
|
||||
<channel id="switch_dimmer4" typeId="switch_dimmer">
|
||||
<label>Dimmer 4</label>
|
||||
<properties>
|
||||
<property name="binding:*:PercentType">COMMAND_CLASS_SWITCH_MULTILEVEL:4,COMMAND_CLASS_BASIC:4</property>
|
||||
<property name="binding:Command:OnOffType">COMMAND_CLASS_SWITCH_MULTILEVEL:4</property>
|
||||
</properties>
|
||||
</channel>
|
||||
<channel id="switch_dimmer5" typeId="switch_dimmer">
|
||||
<label>Dimmer 5</label>
|
||||
<properties>
|
||||
<property name="binding:*:PercentType">COMMAND_CLASS_SWITCH_MULTILEVEL:5,COMMAND_CLASS_BASIC:5</property>
|
||||
<property name="binding:Command:OnOffType">COMMAND_CLASS_SWITCH_MULTILEVEL:5</property>
|
||||
</properties>
|
||||
</channel>
|
||||
<channel id="sensor_voltage6" typeId="sensor_voltage">
|
||||
<label>Sensor (voltage) 6</label>
|
||||
<properties>
|
||||
<property name="binding:*:DecimalType">COMMAND_CLASS_SENSOR_MULTILEVEL:6,COMMAND_CLASS_BASIC:6;type=VOLTAGE</property>
|
||||
</properties>
|
||||
</channel>
|
||||
<channel id="sensor_voltage7" typeId="sensor_voltage">
|
||||
<label>Sensor (voltage) 7</label>
|
||||
<properties>
|
||||
<property name="binding:*:DecimalType">COMMAND_CLASS_SENSOR_MULTILEVEL:7,COMMAND_CLASS_BASIC:7;type=VOLTAGE</property>
|
||||
</properties>
|
||||
</channel>
|
||||
<channel id="sensor_voltage8" typeId="sensor_voltage">
|
||||
<label>Sensor (voltage) 8</label>
|
||||
<properties>
|
||||
<property name="binding:*:DecimalType">COMMAND_CLASS_SENSOR_MULTILEVEL:8,COMMAND_CLASS_BASIC:8;type=VOLTAGE</property>
|
||||
</properties>
|
||||
</channel>
|
||||
<channel id="sensor_voltage9" typeId="sensor_voltage">
|
||||
<label>Sensor (voltage) 9</label>
|
||||
<properties>
|
||||
<property name="binding:*:DecimalType">COMMAND_CLASS_SENSOR_MULTILEVEL:9,COMMAND_CLASS_BASIC:9;type=VOLTAGE</property>
|
||||
</properties>
|
||||
</channel>
|
||||
</channels>
|
||||
|
||||
<!-- DEVICE PROPERTY DEFINITIONS -->
|
||||
<properties>
|
||||
<property name="vendor">Fibargroup</property>
|
||||
<property name="modelId">FGRGBW-442</property>
|
||||
<property name="manufacturerId">010F</property>
|
||||
<property name="manufacturerRef">0902:1000,0902:2000,0902:3000,0902:4000</property>
|
||||
<property name="dbReference">1127</property>
|
||||
<property name="defaultAssociations">1</property>
|
||||
</properties>
|
||||
|
||||
<!-- CONFIGURATION DESCRIPTIONS -->
|
||||
<config-description>
|
||||
|
||||
<!-- GROUP DEFINITIONS -->
|
||||
<parameter-group name="configuration">
|
||||
<context>setup</context>
|
||||
<label>Configuration Parameters</label>
|
||||
</parameter-group>
|
||||
|
||||
<parameter-group name="association">
|
||||
<context>link</context>
|
||||
<label>Association Groups</label>
|
||||
</parameter-group>
|
||||
|
||||
<!-- PARAMETER DEFINITIONS -->
|
||||
<parameter name="config_1_1" type="integer" groupName="configuration">
|
||||
<label>1: Remember device status before the power failure</label>
|
||||
<description><![CDATA[
|
||||
<br /> <h1>Overview</h1><p>This parameter determines how the device will react in the event of power supply failure (e.g. power outage or taking out from the electrical outlet).</p><p>After the power supply is back on, the device can be restored to previous state or remain switched off. The sequence is not remem- bered after the power returns. After power failure, the last color set before the sequence will be restored.</p>
|
||||
]]></description>
|
||||
<default>0</default>
|
||||
<options>
|
||||
<option value="0">device remains switched off</option>
|
||||
<option value="1">restore the state</option>
|
||||
</options>
|
||||
<limitToOptions>false</limitToOptions>
|
||||
</parameter>
|
||||
|
||||
<parameter name="config_20_1" type="integer" groupName="configuration">
|
||||
<label>20: Input 1 - operating mode</label>
|
||||
<description><![CDATA[
|
||||
Input 1 - operating mode<br /> <h1>Overview</h1><p>This parameter allows to choose mode of 1st input (IN1). Change it depending on connected device.<br /></p>
|
||||
]]></description>
|
||||
<default>2</default>
|
||||
<options>
|
||||
<option value="0">Analog input without internal pull-up</option>
|
||||
<option value="1">Analog input with internal pull-up</option>
|
||||
<option value="2">Momentary switch (Central Scene)</option>
|
||||
<option value="3">Toggle switch: switch state on every input change</option>
|
||||
<option value="4">Toggle switch: closed – ON, opened – OFF</option>
|
||||
</options>
|
||||
<limitToOptions>false</limitToOptions>
|
||||
</parameter>
|
||||
|
||||
<parameter name="config_21_1" type="integer" groupName="configuration">
|
||||
<label>21: Input 2 - operating mode</label>
|
||||
<description><![CDATA[
|
||||
Input 2 - operating mode<br /> <h1>Overview</h1><p>This parameter allows to choose mode of 2nd input (IN2). Change it depending on connected device.<br /></p>
|
||||
]]></description>
|
||||
<default>2</default>
|
||||
<options>
|
||||
<option value="0">Analog input without internal pull-up</option>
|
||||
<option value="1">Analog input with internal pull-up</option>
|
||||
<option value="2">Momentary switch (Central Scene)</option>
|
||||
<option value="3">Toggle switch: switch state on every input change</option>
|
||||
<option value="4">Toggle switch: closed – ON, opened – OFF</option>
|
||||
</options>
|
||||
<limitToOptions>false</limitToOptions>
|
||||
</parameter>
|
||||
|
||||
<parameter name="config_22_1" type="integer" groupName="configuration">
|
||||
<label>22: Input 3 - operating mode</label>
|
||||
<description><![CDATA[
|
||||
Input 3 - operating mode<br /> <h1>Overview</h1><p>This parameter allows to choose mode of 3rd input (IN3). Change it depending on connected device.<br /></p>
|
||||
]]></description>
|
||||
<default>2</default>
|
||||
<options>
|
||||
<option value="0">Analog input without internal pull-up</option>
|
||||
<option value="1">Analog input with internal pull-up</option>
|
||||
<option value="2">Momentary switch (Central Scene)</option>
|
||||
<option value="3">Toggle switch: switch state on every input change</option>
|
||||
<option value="4">Toggle switch: closed – ON, opened – OFF</option>
|
||||
</options>
|
||||
<limitToOptions>false</limitToOptions>
|
||||
</parameter>
|
||||
|
||||
<parameter name="config_23_1" type="integer" groupName="configuration">
|
||||
<label>23: Input 4 - operating mode</label>
|
||||
<description><![CDATA[
|
||||
Input 4 - operating mode<br /> <h1>Overview</h1><p>This parameter allows to choose mode of 4th input (IN4). Change it depending on connected device.<br /></p>
|
||||
]]></description>
|
||||
<default>2</default>
|
||||
<options>
|
||||
<option value="0">Analog input without internal pull-up</option>
|
||||
<option value="1">Analog input with internal pull-up</option>
|
||||
<option value="2">Momentary switch (Central Scene)</option>
|
||||
<option value="3">Toggle switch: switch state on every input change</option>
|
||||
<option value="4">Toggle switch:closed – ON, opened – OF</option>
|
||||
</options>
|
||||
<limitToOptions>false</limitToOptions>
|
||||
</parameter>
|
||||
|
||||
<parameter name="config_30_4_000000FF" type="integer" groupName="configuration"
|
||||
min="0" max="255">
|
||||
<label>30: Alarm configuration - 1st slot - 4B [LSB]</label>
|
||||
<description><![CDATA[
|
||||
Alarm configuration - 1st slot (Action)<br /> <h1>Overview</h1><p>4B [LSB] – action:</p> 0x00 – no reaction,<br />0x0X – turn off selected channel,<br />0x1X – turn on selected channel,<br />0x2X – blink selected channel,<br />0x3Y – activate alarm sequenceX – channels summarized: 1/2/3/4 channel are equal to values 1/2/4/8.<br />Y – sequence number: 1-10 (parameter 157).
|
||||
]]></description>
|
||||
<default>0</default>
|
||||
<limitToOptions>false</limitToOptions>
|
||||
</parameter>
|
||||
|
||||
<parameter name="config_30_4_0000FF00" type="integer" groupName="configuration"
|
||||
min="0" max="255">
|
||||
<label>30: Alarm configuration - 1st slot - 3B</label>
|
||||
<description><![CDATA[
|
||||
Alarm configuration - 1st slot (Event/State Parameters)<br /> <h1>Overview</h1><p>3B – Event/State Parameters</p><p>0x00</p>
|
||||
]]></description>
|
||||
<default>0</default>
|
||||
<limitToOptions>false</limitToOptions>
|
||||
</parameter>
|
||||
|
||||
<parameter name="config_30_4_00FF0000" type="integer" groupName="configuration"
|
||||
min="0" max="255">
|
||||
<label>30: Alarm configuration - 1st slot - 2B</label>
|
||||
<description><![CDATA[
|
||||
Alarm configuration - 1st slot (Notification Status)<br /> <h1>Overview</h1><p>2B – Notification Status</p><p>0x00 - disabled</p>
|
||||
]]></description>
|
||||
<default>0</default>
|
||||
<limitToOptions>false</limitToOptions>
|
||||
</parameter>
|
||||
|
||||
<parameter name="config_30_4_7F000000" type="integer" groupName="configuration"
|
||||
min="0" max="255">
|
||||
<label>30: Alarm configuration - 1st slot - 1B [MSB]</label>
|
||||
<description><![CDATA[
|
||||
Alarm configuration - 1st slot (Notification Type)<br /> <h1>Overview</h1><p>1B [MSB] – Notification Type</p><p>0x00 - disabled</p>
|
||||
]]></description>
|
||||
<default>0</default>
|
||||
<limitToOptions>false</limitToOptions>
|
||||
</parameter>
|
||||
|
||||
<parameter name="config_31_4_000000FF" type="integer" groupName="configuration"
|
||||
min="0" max="255">
|
||||
<label>31: Alarm configuration - 2nd slot - 4B [LSB]</label>
|
||||
<description><![CDATA[
|
||||
Alarm configuration - 2nd slot - Water (Action )<br /> <h1>Overview</h1><p>4B [LSB] – action:</p>0x00 – no reaction,<br />0x0X – turn off selected channel,<br />0x1X – turn on selected channel,<br />0x2X – blink selected channel,<br />0x3Y – activate alarm sequenceX – channels summarized: 1/2/3/4 channel are equal to values 1/2/4/8.<br />Y – sequence number: 1-10 (parameter 157).
|
||||
]]></description>
|
||||
<default>0</default>
|
||||
<limitToOptions>false</limitToOptions>
|
||||
</parameter>
|
||||
|
||||
<parameter name="config_31_4_0000FF00" type="integer" groupName="configuration"
|
||||
min="0" max="255">
|
||||
<label>31: Alarm configuration - 2nd slot - 3B</label>
|
||||
<description><![CDATA[
|
||||
Alarm configuration - 2nd slot - Water (Event/State Parameters)<br /> <h1>Overview</h1><p>3B – Event/State Parameters</p><p>0x00</p>
|
||||
]]></description>
|
||||
<default>0</default>
|
||||
<limitToOptions>false</limitToOptions>
|
||||
</parameter>
|
||||
|
||||
<parameter name="config_31_4_00FF0000" type="integer" groupName="configuration"
|
||||
min="0" max="255">
|
||||
<label>31: Alarm configuration - 2nd slot - 2B</label>
|
||||
<description><![CDATA[
|
||||
Alarm configuration - 2nd slot - Water (Notification Status)<br /> <h1>Overview</h1><p>2B – Notification Status</p><p>0xFF - any notification</p>
|
||||
]]></description>
|
||||
<default>255</default>
|
||||
<limitToOptions>false</limitToOptions>
|
||||
</parameter>
|
||||
|
||||
<parameter name="config_31_4_7F000000" type="integer" groupName="configuration"
|
||||
min="0" max="255">
|
||||
<label>31: Alarm configuration - 2nd slot - 1B [MSB]</label>
|
||||
<description><![CDATA[
|
||||
Alarm configuration - 2nd slot - Water (Notification Type)<br /> <h1>Overview</h1><p>1B [MSB] – Notification Type</p><p>0x05 - Water</p>
|
||||
]]></description>
|
||||
<default>5</default>
|
||||
<limitToOptions>false</limitToOptions>
|
||||
</parameter>
|
||||
|
||||
<parameter name="config_32_4_000000FF" type="integer" groupName="configuration"
|
||||
min="0" max="255">
|
||||
<label>32: Alarm configuration - 3rd slot - 4B [LSB]</label>
|
||||
<description><![CDATA[
|
||||
Alarm configuration - 3rd slot - Smoke (Action )<br /> <h1>Overview</h1><p>4B [LSB] – action:</p>0x00 – no reaction,<br />0x0X – turn off selected channel,<br />0x1X – turn on selected channel,<br />0x2X – blink selected channel,<br />0x3Y – activate alarm sequenceX – channels summarized: 1/2/3/4 channel are equal to values 1/2/4/8.<br />Y – sequence number: 1-10 (parameter 157).
|
||||
]]></description>
|
||||
<default>0</default>
|
||||
<limitToOptions>false</limitToOptions>
|
||||
</parameter>
|
||||
|
||||
<parameter name="config_32_4_0000FF00" type="integer" groupName="configuration"
|
||||
min="0" max="255">
|
||||
<label>32: Alarm configuration - 3rd slot - 3B</label>
|
||||
<description><![CDATA[
|
||||
Alarm configuration - 3rd slot - Smoke (Event/State Parameters)<br /> <h1>Overview</h1><p>3B – Event/State Parameters</p><p>0x00</p>
|
||||
]]></description>
|
||||
<default>0</default>
|
||||
<limitToOptions>false</limitToOptions>
|
||||
</parameter>
|
||||
|
||||
<parameter name="config_32_4_00FF0000" type="integer" groupName="configuration"
|
||||
min="0" max="255">
|
||||
<label>32: Alarm configuration - 3rd slot - 2B</label>
|
||||
<description><![CDATA[
|
||||
Alarm configuration - 3rd slot - Smoke (Notification Status)<br /> <h1>Overview</h1><p>2B – Notification Status</p><p>0xFF - any notification</p>
|
||||
]]></description>
|
||||
<default>255</default>
|
||||
<limitToOptions>false</limitToOptions>
|
||||
</parameter>
|
||||
|
||||
<parameter name="config_32_4_7F000000" type="integer" groupName="configuration"
|
||||
min="0" max="255">
|
||||
<label>32: Alarm configuration - 3rd slot - 1B [MSB]</label>
|
||||
<description><![CDATA[
|
||||
Alarm configuration - 3rd slot - Smoke (Notification Type)<br /> <h1>Overview</h1><p>1B [MSB] – Notification Type</p><p>0x01 - Smoke Alarm</p>
|
||||
]]></description>
|
||||
<default>1</default>
|
||||
<limitToOptions>false</limitToOptions>
|
||||
</parameter>
|
||||
|
||||
<parameter name="config_33_4_000000FF" type="integer" groupName="configuration"
|
||||
min="0" max="255">
|
||||
<label>33: Alarm configuration - 4th slot - 4B [LSB]</label>
|
||||
<description><![CDATA[
|
||||
Alarm configuration - 4th slot - CO (Action )<br /> <h1>Overview</h1><p>4B [LSB] – action:</p>0x00 – no reaction,<br />0x0X – turn off selected channel,<br />0x1X – turn on selected channel,<br />0x2X – blink selected channel,<br />0x3Y – activate alarm sequenceX – channels summarized: 1/2/3/4 channel are equal to values 1/2/4/8.<br />Y – sequence number: 1-10 (parameter 157).
|
||||
]]></description>
|
||||
<default>0</default>
|
||||
<limitToOptions>false</limitToOptions>
|
||||
</parameter>
|
||||
|
||||
<parameter name="config_33_4_0000FF00" type="integer" groupName="configuration"
|
||||
min="0" max="255">
|
||||
<label>33: Alarm configuration - 4th slot - 3B</label>
|
||||
<description><![CDATA[
|
||||
Alarm configuration - 4th slot - CO (Event/State Parameters)<br /> <h1>Overview</h1><p>3B – Event/State Parameters</p><p>0x00</p>
|
||||
]]></description>
|
||||
<default>0</default>
|
||||
<limitToOptions>false</limitToOptions>
|
||||
</parameter>
|
||||
|
||||
<parameter name="config_33_4_00FF0000" type="integer" groupName="configuration"
|
||||
min="0" max="255">
|
||||
<label>33: Alarm configuration - 4th slot - 2B</label>
|
||||
<description><![CDATA[
|
||||
Alarm configuration - 4th slot - CO (Notification Status)<br /> <h1>Overview</h1><p>2B – Notification Status</p><p>0xFF - any notification</p>
|
||||
]]></description>
|
||||
<default>255</default>
|
||||
<limitToOptions>false</limitToOptions>
|
||||
</parameter>
|
||||
|
||||
<parameter name="config_33_4_7F000000" type="integer" groupName="configuration"
|
||||
min="0" max="255">
|
||||
<label>33: Alarm configuration - 4th slot - 1B [MSB]</label>
|
||||
<description><![CDATA[
|
||||
Alarm configuration - 4th slot - CO (Notification Type)<br /> <h1>Overview</h1><p>1B [MSB] – Notification Type</p><p>0x02 - CO Alarm</p>
|
||||
]]></description>
|
||||
<default>2</default>
|
||||
<limitToOptions>false</limitToOptions>
|
||||
</parameter>
|
||||
|
||||
<parameter name="config_34_4_000000FF" type="integer" groupName="configuration"
|
||||
min="0" max="255">
|
||||
<label>34: Alarm configuration - 5th slot - 4B [LSB]</label>
|
||||
<description><![CDATA[
|
||||
Alarm configuration - 5th slot - Heat (Action )<br /> <h1>Overview</h1><p>4B [LSB] – action:</p>0x00 – no reaction,<br />0x0X – turn off selected channel,<br />0x1X – turn on selected channel,<br />0x2X – blink selected channel,<br />0x3Y – activate alarm sequenceX – channels summarized: 1/2/3/4 channel are equal to values 1/2/4/8.<br />Y – sequence number: 1-10 (parameter 157).
|
||||
]]></description>
|
||||
<default>0</default>
|
||||
<limitToOptions>false</limitToOptions>
|
||||
</parameter>
|
||||
|
||||
<parameter name="config_34_4_0000FF00" type="integer" groupName="configuration"
|
||||
min="0" max="255">
|
||||
<label>34: Alarm configuration - 5th slot - 3B</label>
|
||||
<description><![CDATA[
|
||||
Alarm configuration - 5th slot - Heat (Event/State Parameters)<br /> <h1>Overview</h1><p>3B – Event/State Parameters</p><p>0x00</p>
|
||||
]]></description>
|
||||
<default>0</default>
|
||||
<limitToOptions>false</limitToOptions>
|
||||
</parameter>
|
||||
|
||||
<parameter name="config_34_4_00FF0000" type="integer" groupName="configuration"
|
||||
min="0" max="255">
|
||||
<label>34: Alarm configuration - 5th slot - 2B</label>
|
||||
<description><![CDATA[
|
||||
Alarm configuration - 5th slot - Heat (Notification Status)<br /> <h1>Overview</h1><p>2B – Notification Status</p><p>0xFF - any notification</p>
|
||||
]]></description>
|
||||
<default>255</default>
|
||||
<limitToOptions>false</limitToOptions>
|
||||
</parameter>
|
||||
|
||||
<parameter name="config_34_4_7F000000" type="integer" groupName="configuration"
|
||||
min="0" max="255">
|
||||
<label>34: Alarm configuration - 5th slot - 1B [MSB]</label>
|
||||
<description><![CDATA[
|
||||
Alarm configuration - 5th slot - Heat (Notification Type)<br /> <h1>Overview</h1><p>1B [MSB] – Notification Type</p><p>0x04 - Heat Alarm</p>
|
||||
]]></description>
|
||||
<default>4</default>
|
||||
<limitToOptions>false</limitToOptions>
|
||||
</parameter>
|
||||
|
||||
<parameter name="config_35_2_0000FFFF" type="integer" groupName="configuration"
|
||||
min="0" max="32400">
|
||||
<label>35: Duration of alarm signalization</label>
|
||||
<description><![CDATA[
|
||||
Duration of alarm signalization<br /> <h1>Overview</h1><p>This parameter determines the duration of alarm signaling (flashing mode and/or alarm sequence). Available Values:</p><p>0 – infinite signalization,<br />1-32400 (1s-9h, 1s step)</p>
|
||||
]]></description>
|
||||
<default>600</default>
|
||||
<limitToOptions>false</limitToOptions>
|
||||
</parameter>
|
||||
|
||||
<parameter name="config_40_1" type="integer" groupName="configuration"
|
||||
min="0" max="15">
|
||||
<label>40: Input 1 - sent scenes</label>
|
||||
<description><![CDATA[
|
||||
Input 1 - sent scenes<br /> <h1>Overview</h1><p>This parameter defines which actions result in sending scene ID and attribute assigned to them. Parameter is relevant only if parameter 20 is set to 2, 3 or 4.</p><p>Actions can be summed up, e.g. 1+2+4+8=15 and entered as a value for the parameter. Available values</p>1 - Key pressed 1 time<br />2 - Key pressed 2 times<br />3 - Key pressed 3 times<br />4 - Key hold down and key released
|
||||
]]></description>
|
||||
<default>15</default>
|
||||
<limitToOptions>false</limitToOptions>
|
||||
</parameter>
|
||||
|
||||
<parameter name="config_41_1" type="integer" groupName="configuration"
|
||||
min="0" max="15">
|
||||
<label>41: Input 2 - sent scenes</label>
|
||||
<description><![CDATA[
|
||||
Input 2 - sent scenes<br /> <h1>Overview</h1><p>This parameter defines which actions result in sending scene ID and attribute assigned to them. Parameter is relevant only if parameter 21 is set to 2, 3 or 4.</p><p>Actions can be summed up, e.g. 1+2+4+8=15 and entered as a value for the parameter. Available values</p>1 - Key pressed 1 time<br />2 - Key pressed 2 times<br />3 - Key pressed 3 times<br />4 - Key hold down and key released
|
||||
]]></description>
|
||||
<default>15</default>
|
||||
<limitToOptions>false</limitToOptions>
|
||||
</parameter>
|
||||
|
||||
<parameter name="config_42_1" type="integer" groupName="configuration"
|
||||
min="0" max="15">
|
||||
<label>42: Input 3 - sent scenes</label>
|
||||
<description><![CDATA[
|
||||
Input 3 - sent scenes<br /> <h1>Overview</h1><p>This parameter defines which actions result in sending scene ID and attribute assigned to them. Parameter is relevant only if parameter 22 is set to 2, 3 or 4.</p><p>Actions can be summed up, e.g. 1+2+4+8=15 and entered as a value for the parameter. Available values</p>1 - Key pressed 1 time<br />2 - Key pressed 2 times<br />3 - Key pressed 3 times<br />4 - Key hold down and key released
|
||||
]]></description>
|
||||
<default>15</default>
|
||||
<limitToOptions>false</limitToOptions>
|
||||
</parameter>
|
||||
|
||||
<parameter name="config_43_1" type="integer" groupName="configuration"
|
||||
min="0" max="15">
|
||||
<label>43: Input 4 - sent scenes</label>
|
||||
<description><![CDATA[
|
||||
Input 4 - sent scenes<br /> <h1>Overview</h1><p>This parameter defines which actions result in sending scene ID and attribute assigned to them. Parameter is relevant only if parameter 23 is set to 2, 3 or 4.</p><p>Actions can be summed up, e.g. 1+2+4+8=15 and entered as a value for the parameter. Available values</p>1 - Key pressed 1 time<br />2 - Key pressed 2 times<br />3 - Key pressed 3 times<br />4 - Key hold down and key released
|
||||
]]></description>
|
||||
<default>15</default>
|
||||
<limitToOptions>false</limitToOptions>
|
||||
</parameter>
|
||||
|
||||
<parameter name="config_62_2" type="integer" groupName="configuration"
|
||||
min="0" max="32400">
|
||||
<label>62: Power reports - periodic</label>
|
||||
<description><![CDATA[
|
||||
Power reports - periodic<br /> <h1>Overview</h1><p>This parameter determines in what time intervals the periodic power reports are sent to the main controller. Periodic reports do not depend of power change (parameter 61).</p> <ul><li>0 - periodic reports are disabled</li> <li>30-32400 (30-32400s) - report interval</li> <li>Default - 3600 (1h)</li> </ul>
|
||||
]]></description>
|
||||
<default>3600</default>
|
||||
<limitToOptions>false</limitToOptions>
|
||||
</parameter>
|
||||
|
||||
<parameter name="config_63_2" type="integer" groupName="configuration"
|
||||
min="0" max="100">
|
||||
<label>63: Analog inputs output change on input change</label>
|
||||
<description><![CDATA[
|
||||
Analog inputs output change on input change<br /> <h1>Overview</h1><h1>Analog inputs reports and output change on input change</h1> <p>This parameter defines minimal change (from the last reported) of analog input voltage that results in sending new report and change in the output value. Parameter is relevant only for analog inpu ts (parameter 20, 21, 22 or 23 set to 0 or 1).</p> <ul><li>0 - reporting on change disabled</li> <li>1-100 (0.1-10V, 0.1V step)</li> <li>Default - 5 (0.5V)</li> </ul>
|
||||
]]></description>
|
||||
<default>5</default>
|
||||
<limitToOptions>false</limitToOptions>
|
||||
</parameter>
|
||||
|
||||
<parameter name="config_64_2" type="integer" groupName="configuration"
|
||||
min="0" max="32400">
|
||||
<label>64: Analog inputs reports - periodic</label>
|
||||
<description><![CDATA[
|
||||
Analog inputs reports - periodic<br /> <h1>Overview</h1><p>This parameter defines reporting period of analog inputs value.</p> <p>Periodical reports are independent from changes in value (parameter 63).</p> <p>Parameter is relevant only for analog inputs (parameter 20, 21, 22 or 23 set to 0 or 1)</p> <ul><li>0 – periodical reports disabled</li> <li>30-32400 (30-32400s, 1s step)</li> </ul>
|
||||
]]></description>
|
||||
<default>0</default>
|
||||
<limitToOptions>false</limitToOptions>
|
||||
</parameter>
|
||||
|
||||
<parameter name="config_65_2" type="integer" groupName="configuration"
|
||||
min="0" max="500">
|
||||
<label>65: Energy reports - on change</label>
|
||||
<description><![CDATA[
|
||||
Energy reports - on change<br /> <h1>Overview</h1><p>This parameter determines the minimum change in consumed energy that will result in sending new energy report to the main controller. Energy reports are sent no often than every 30 seconds.</p> <ul><li>0 - reports are disabled</li> <li>1-500 (0.01 - 5 kWh) - change in energy</li> </ul>
|
||||
]]></description>
|
||||
<default>10</default>
|
||||
<limitToOptions>false</limitToOptions>
|
||||
</parameter>
|
||||
|
||||
<parameter name="config_66_2" type="integer" groupName="configuration"
|
||||
min="0" max="32400">
|
||||
<label>66: Energy reports - periodic</label>
|
||||
<description><![CDATA[
|
||||
Energy reports - periodic<br /> <h1>Overview</h1><p>This parameter determines in what time intervals the periodic energy reports are sent to the main controller. Periodic reports do not depend of energy change (parameter 65)</p> <ul><li>0 - periodic reports are disabled</li> <li>30-32400 (30-32400s) - report interval</li> </ul>
|
||||
]]></description>
|
||||
<default>3600</default>
|
||||
<limitToOptions>false</limitToOptions>
|
||||
</parameter>
|
||||
|
||||
<parameter name="config_150_1" type="integer" groupName="configuration">
|
||||
<label>150: Inputs - LED colour control mode</label>
|
||||
<description><![CDATA[
|
||||
Inputs - LED colour control mode<br /> <h1>Overview</h1><p>This parameter determines how connected switches control LED strips.<br /></p>0 – RGBW mode (every input controls output with the same number, IN1-OUT1, IN2-OUT2, IN3-OUT3, IN4-OUT4)<br />1 – HSB and White mode (inputs works in HSB color model, IN1-H (Hue), IN2-S (Saturation), IN3-B (Brightness), IN4-White (OUT4)
|
||||
]]></description>
|
||||
<default>0</default>
|
||||
<options>
|
||||
<option value="0">RGBW mode</option>
|
||||
<option value="1">HSB and White mode</option>
|
||||
</options>
|
||||
<limitToOptions>false</limitToOptions>
|
||||
</parameter>
|
||||
|
||||
<parameter name="config_151_2" type="integer" groupName="configuration"
|
||||
min="0" max="254">
|
||||
<label>151: Local control - transition time</label>
|
||||
<description><![CDATA[
|
||||
Local control - transition time<br /> <h1>Overview</h1><p>This parameter determines time of smooth transition between 0% and 100% when controlling with connected switches.</p> <ul><li>0 – instantly</li> <li>1-127 (1s-127s, 1s step)</li> <li>128-254 (1min-127min, 1min step)</li><li>3 (3s) Default<br /></li> </ul>
|
||||
]]></description>
|
||||
<default>3</default>
|
||||
<limitToOptions>false</limitToOptions>
|
||||
</parameter>
|
||||
|
||||
<parameter name="config_152_2" type="integer" groupName="configuration"
|
||||
min="0" max="254">
|
||||
<label>152: Remote control - transition time</label>
|
||||
<description><![CDATA[
|
||||
Remote control - transition time<br /> <h1>Overview</h1><p>This parameter determines time needed to change the state between current and target values when controlling via Z-Wave network.</p> <ul><li>0 – instantly</li> <li>1-127 (1s-127s, 1s step)</li> <li>128-254 (1min-127min, 1min step)</li><li>3 (3s) Default</li> </ul>
|
||||
]]></description>
|
||||
<default>3</default>
|
||||
<limitToOptions>false</limitToOptions>
|
||||
</parameter>
|
||||
|
||||
<parameter name="config_154_4" type="integer" groupName="configuration"
|
||||
min="0" max="4294967295">
|
||||
<label>154: ON frame value for single click</label>
|
||||
<description><![CDATA[
|
||||
ON frame value for single click<br /> <h1>Overview</h1><p>For every byte: 0-99, 255<br /></p>
|
||||
]]></description>
|
||||
<default>4294967295</default>
|
||||
<advanced>true</advanced>
|
||||
<limitToOptions>false</limitToOptions>
|
||||
</parameter>
|
||||
|
||||
<parameter name="config_154_4_000000FF" type="integer" groupName="configuration"
|
||||
min="0" max="255">
|
||||
<label>154: ON frame value for single click IN1</label>
|
||||
<description><![CDATA[
|
||||
ON frame value for single click IN1<br /> <h1>Overview</h1><p>For every byte: 0-99, 255<br /></p>
|
||||
]]></description>
|
||||
<default>255</default>
|
||||
<limitToOptions>false</limitToOptions>
|
||||
</parameter>
|
||||
|
||||
<parameter name="config_154_4_0000FF00" type="integer" groupName="configuration"
|
||||
min="0" max="255">
|
||||
<label>154: ON frame value for single click IN2</label>
|
||||
<description><![CDATA[
|
||||
ON frame value for single click IN2<br /> <h1>Overview</h1><p>For every byte: 0-99, 255<br /></p>
|
||||
]]></description>
|
||||
<default>255</default>
|
||||
<limitToOptions>false</limitToOptions>
|
||||
</parameter>
|
||||
|
||||
<parameter name="config_154_4_00FF0000" type="integer" groupName="configuration"
|
||||
min="0" max="255">
|
||||
<label>154: ON frame value for single click IN3</label>
|
||||
<description><![CDATA[
|
||||
ON frame value for single click IN3<br /> <h1>Overview</h1><p>For every byte: 0-99, 255<br /></p>
|
||||
]]></description>
|
||||
<default>255</default>
|
||||
<limitToOptions>false</limitToOptions>
|
||||
</parameter>
|
||||
|
||||
<parameter name="config_154_4_7F000000" type="integer" groupName="configuration"
|
||||
min="0" max="255">
|
||||
<label>154: ON frame value for single click IN4</label>
|
||||
<description><![CDATA[
|
||||
ON frame value for single click IN4<br /> <h1>Overview</h1><p>For every byte: 0-99, 255<br /></p>
|
||||
]]></description>
|
||||
<default>255</default>
|
||||
<limitToOptions>false</limitToOptions>
|
||||
</parameter>
|
||||
|
||||
<parameter name="config_155_4" type="integer" groupName="configuration"
|
||||
min="0" max="4294967295">
|
||||
<label>155: OFF frame value for single click</label>
|
||||
<description><![CDATA[
|
||||
OFF frame value for single click<br /> <h1>Overview</h1><p>For every byte: 0-99, 255<br /></p>
|
||||
]]></description>
|
||||
<default>0</default>
|
||||
<advanced>true</advanced>
|
||||
<limitToOptions>false</limitToOptions>
|
||||
</parameter>
|
||||
|
||||
<parameter name="config_155_4_000000FF" type="integer" groupName="configuration"
|
||||
min="0" max="255">
|
||||
<label>155: OFF frame value for single click IN1</label>
|
||||
<description><![CDATA[
|
||||
OFF frame value for single click IN1<br /> <h1>Overview</h1><p>For every byte: 0-99, 255<br /></p>
|
||||
]]></description>
|
||||
<default>0</default>
|
||||
<limitToOptions>false</limitToOptions>
|
||||
</parameter>
|
||||
|
||||
<parameter name="config_155_4_0000FF00" type="integer" groupName="configuration"
|
||||
min="0" max="255">
|
||||
<label>155: OFF frame value for single click IN2</label>
|
||||
<description><![CDATA[
|
||||
OFF frame value for single click IN2<br /> <h1>Overview</h1><p>For every byte: 0-99, 255<br /></p>
|
||||
]]></description>
|
||||
<default>0</default>
|
||||
<limitToOptions>false</limitToOptions>
|
||||
</parameter>
|
||||
|
||||
<parameter name="config_155_4_00FF0000" type="integer" groupName="configuration"
|
||||
min="0" max="255">
|
||||
<label>155: OFF frame value for single click IN3</label>
|
||||
<description><![CDATA[
|
||||
OFF frame value for single click IN3<br /> <h1>Overview</h1><p>For every byte: 0-99, 255<br /></p>
|
||||
]]></description>
|
||||
<default>0</default>
|
||||
<limitToOptions>false</limitToOptions>
|
||||
</parameter>
|
||||
|
||||
<parameter name="config_155_4_7F000000" type="integer" groupName="configuration"
|
||||
min="0" max="255">
|
||||
<label>155: OFF frame value for single click IN4</label>
|
||||
<description><![CDATA[
|
||||
OFF frame value for single click IN4<br /> <h1>Overview</h1><p>For every byte: 0-99, 255<br /></p>
|
||||
]]></description>
|
||||
<default>0</default>
|
||||
<limitToOptions>false</limitToOptions>
|
||||
</parameter>
|
||||
|
||||
<parameter name="config_156_4" type="integer" groupName="configuration"
|
||||
min="0" max="4294967295">
|
||||
<label>156: ON frame value for double click</label>
|
||||
<description><![CDATA[
|
||||
ON frame value for double click<br /> <h1>Overview</h1><p>For every byte: 0-99, 255<br /></p>
|
||||
]]></description>
|
||||
<default>1667457891</default>
|
||||
<advanced>true</advanced>
|
||||
<limitToOptions>false</limitToOptions>
|
||||
</parameter>
|
||||
|
||||
<parameter name="config_156_4_000000FF" type="integer" groupName="configuration"
|
||||
min="0" max="255">
|
||||
<label>156: ON frame value for double click IN1</label>
|
||||
<description><![CDATA[
|
||||
ON frame value for double click IN1<br /> <h1>Overview</h1><p>For every byte: 0-99, 255<br /></p>
|
||||
]]></description>
|
||||
<default>99</default>
|
||||
<limitToOptions>false</limitToOptions>
|
||||
</parameter>
|
||||
|
||||
<parameter name="config_156_4_0000FF00" type="integer" groupName="configuration"
|
||||
min="0" max="255">
|
||||
<label>156: ON frame value for double click IN2</label>
|
||||
<description><![CDATA[
|
||||
ON frame value for double click IN2<br /> <h1>Overview</h1><p>For every byte: 0-99, 255<br /></p>
|
||||
]]></description>
|
||||
<default>99</default>
|
||||
<limitToOptions>false</limitToOptions>
|
||||
</parameter>
|
||||
|
||||
<parameter name="config_156_4_00FF0000" type="integer" groupName="configuration"
|
||||
min="0" max="255">
|
||||
<label>156: ON frame value for double click IN3</label>
|
||||
<description><![CDATA[
|
||||
ON frame value for double click IN3<br /> <h1>Overview</h1><p>For every byte: 0-99, 255<br /></p>
|
||||
]]></description>
|
||||
<default>99</default>
|
||||
<limitToOptions>false</limitToOptions>
|
||||
</parameter>
|
||||
|
||||
<parameter name="config_156_4_7F000000" type="integer" groupName="configuration"
|
||||
min="0" max="255">
|
||||
<label>156: ON frame value for double click IN4</label>
|
||||
<description><![CDATA[
|
||||
ON frame value for double click IN4<br /> <h1>Overview</h1><p>For every byte: 0-99, 255<br /></p>
|
||||
]]></description>
|
||||
<default>99</default>
|
||||
<limitToOptions>false</limitToOptions>
|
||||
</parameter>
|
||||
|
||||
<parameter name="config_157_1" type="integer" groupName="configuration">
|
||||
<label>157: Start programmed sequence</label>
|
||||
<description><![CDATA[
|
||||
Start programmed sequence<br /> <h1>Overview</h1><p>Setting this parameter will start programmed sequence with selected number. User can define own sequences via controller. While the sequence is active, the menu is unavailable.</p>
|
||||
]]></description>
|
||||
<default>0</default>
|
||||
<options>
|
||||
<option value="0">sequence inactive</option>
|
||||
<option value="1">user-defined sequence 1</option>
|
||||
<option value="2">user-defined sequence 2</option>
|
||||
<option value="3">user-defined sequence 3</option>
|
||||
<option value="4">user-defined sequence 4</option>
|
||||
<option value="5">user-defined sequence 5</option>
|
||||
<option value="6">Fireplace sequence</option>
|
||||
<option value="7">Storm sequence</option>
|
||||
<option value="8">Rainbow sequence</option>
|
||||
<option value="9">Aurora sequence</option>
|
||||
<option value="10">Police (red-white-blue) sequence</option>
|
||||
</options>
|
||||
<limitToOptions>false</limitToOptions>
|
||||
</parameter>
|
||||
|
||||
<!-- ASSOCIATION DEFINITIONS -->
|
||||
<parameter name="group_1" type="text" groupName="association">
|
||||
<label>1: Lifeline</label>
|
||||
</parameter>
|
||||
|
||||
<parameter name="group_2" type="text" groupName="association" multiple="true">
|
||||
<label>2: RGBW Sync</label>
|
||||
<description><![CDATA[
|
||||
<br /> <h1>Overview</h1><p>allows to synchronize state of other FIBARO RGBW Controller devices (FGRGBW-442 and FGRGBWM- 441)</p> <p>- do not use with other devices.</p>
|
||||
]]></description>
|
||||
<multipleLimit>5</multipleLimit>
|
||||
</parameter>
|
||||
|
||||
<parameter name="group_3" type="text" groupName="association" multiple="true">
|
||||
<label>3: On/Off (IN1)</label>
|
||||
<description><![CDATA[
|
||||
<br /> <h1>Overview</h1><p>used to turn the associated devices on/off reflecting IN1 operation.</p>
|
||||
]]></description>
|
||||
<multipleLimit>5</multipleLimit>
|
||||
</parameter>
|
||||
|
||||
<parameter name="group_4" type="text" groupName="association" multiple="true">
|
||||
<label>4: Dimmer (IN1)</label>
|
||||
<description><![CDATA[
|
||||
<br /> <h1>Overview</h1><p>used to change level of associated devices reflecting IN1 operation.</p>
|
||||
]]></description>
|
||||
<multipleLimit>5</multipleLimit>
|
||||
</parameter>
|
||||
|
||||
<parameter name="group_5" type="text" groupName="association" multiple="true">
|
||||
<label>5: On/Off (IN2)</label>
|
||||
<description><![CDATA[
|
||||
<br /> <h1>Overview</h1><p>used to turn the associated devices on/off reflecting IN2 operation.</p>
|
||||
]]></description>
|
||||
<multipleLimit>5</multipleLimit>
|
||||
</parameter>
|
||||
|
||||
<parameter name="group_6" type="text" groupName="association" multiple="true">
|
||||
<label>6: Dimmer (IN2)</label>
|
||||
<description><![CDATA[
|
||||
<br /> <h1>Overview</h1><p>used to change level of associated devices reflecting IN2 operation.</p>
|
||||
]]></description>
|
||||
<multipleLimit>5</multipleLimit>
|
||||
</parameter>
|
||||
|
||||
<parameter name="group_7" type="text" groupName="association" multiple="true">
|
||||
<label>7: On/Off (IN3)</label>
|
||||
<description><![CDATA[
|
||||
<br /> <h1>Overview</h1><p>used to turn the associated devices on/off reflecting IN3 operation.</p>
|
||||
]]></description>
|
||||
<multipleLimit>5</multipleLimit>
|
||||
</parameter>
|
||||
|
||||
<parameter name="group_8" type="text" groupName="association" multiple="true">
|
||||
<label>8: Dimmer (IN3)</label>
|
||||
<description><![CDATA[
|
||||
<br /> <h1>Overview</h1><p>used to change level of associated devices reflecting IN3 operation.</p>
|
||||
]]></description>
|
||||
<multipleLimit>5</multipleLimit>
|
||||
</parameter>
|
||||
|
||||
<parameter name="group_9" type="text" groupName="association" multiple="true">
|
||||
<label>9: On/Off (IN4)</label>
|
||||
<description><![CDATA[
|
||||
<br /> <h1>Overview</h1><p>used to turn the associated devices on/off reflecting IN4 operation.</p>
|
||||
]]></description>
|
||||
<multipleLimit>5</multipleLimit>
|
||||
</parameter>
|
||||
|
||||
<parameter name="group_10" type="text" groupName="association" multiple="true">
|
||||
<label>10: Dimmer (IN4)</label>
|
||||
<description><![CDATA[
|
||||
<br /> <h1>Overview</h1><p>used to change level of associated devices reflecting IN4 operation.</p>
|
||||
]]></description>
|
||||
<multipleLimit>5</multipleLimit>
|
||||
</parameter>
|
||||
|
||||
<!-- STATIC DEFINITIONS -->
|
||||
<parameter name="node_id" type="integer" min="1" max="232" readOnly="true" required="true">
|
||||
<label>Node ID</label>
|
||||
<advanced>true</advanced>
|
||||
</parameter>
|
||||
|
||||
</config-description>
|
||||
|
||||
</thing-type>
|
||||
|
||||
<channel-type id="fibaro_fgrgbw442_00_000_config_decimal_param157">
|
||||
<item-type>Number</item-type>
|
||||
<label>Start programmed sequence</label>
|
||||
<description>Start programmed sequence</description>
|
||||
<state pattern="%s">
|
||||
<options>
|
||||
<option value="0">sequence inactive</option>
|
||||
<option value="1">user-defined sequence 1</option>
|
||||
<option value="2">user-defined sequence 2</option>
|
||||
<option value="3">user-defined sequence 3</option>
|
||||
<option value="4">user-defined sequence 4</option>
|
||||
<option value="5">user-defined sequence 5</option>
|
||||
<option value="6">Fireplace sequence</option>
|
||||
<option value="7">Storm sequence</option>
|
||||
<option value="8">Rainbow sequence</option>
|
||||
<option value="9">Aurora sequence</option>
|
||||
<option value="10">Police (red-white-blue) sequence</option>
|
||||
</options>
|
||||
</state>
|
||||
</channel-type>
|
||||
|
||||
</thing:thing-descriptions>
|
|
@ -0,0 +1,173 @@
|
|||
<?xml version="1.0" encoding="UTF-8"?>
|
||||
<thing:thing-descriptions bindingId="zwave"
|
||||
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
|
||||
xmlns:thing="https://openhab.org/schemas/thing-description/v1.0.0"
|
||||
xsi:schemaLocation="https://openhab.org/schemas/thing-description/v1.0.0
|
||||
https://openhab.org/schemas/thing-description/v1.0.0">
|
||||
|
||||
<thing-type id="fibaro_fgwceu-201_00_000" listed="false">
|
||||
<label>FGWCEU-201 FIBARO Walli Double Wall Mounted Remote Controller</label>
|
||||
<description><![CDATA[
|
||||
FIBARO Walli Double Wall Mounted Remote Controller<br /> <h1>Overview</h1><p>FIBARO Walli Controller is a smart wall-mounted Z-Wave™ remote controller that can activate scenes or control other Z-Wave devices via associations.</p><p>Main features:<br />• Can be used for controlling multiple types of devices, e.g. switches, dimmers, roller shutters.<br />• Pre-set configurations allow to easily adjust operation for specific type of controlled devices.<br />• Battery or VDC powered.<br />• Equipped with a built-in temperature sensor.<br /></p><p>• Supports Z-Wave network Security Modes: S0 with AES-128 encryption and S2 Authenticated with PRNG-based encryption.<br />• Works as a Z-Wave signal repeater when VDC powered (all non-battery operated devices within the network will act as repeaters to increase reliability of the network).<br />• May be used with all devices certified with the Z-Wave Plus™ certificate and should be compatible with such devices produced by other manufacturers</p> <br /> <h2>Inclusion Information</h2><p>To add the device to the Z-Wave network manually:</p><p>1. Set the main controller in (Security/non-Security Mode) add mode (see the controller’s manual).<br />2. Quickly, three times click one of the buttons.<br />3. If you are adding in Security S2 Authenticated, input the underlined part of the DSK (label on the box).<br />4. LED will start blinking yellow, wait for the adding process to end.<br />5. Adding result will be confirmed by the Z-Wave controller’s message and the LED frame:<br />• Green – successful (non-secure, S0, S2 non-authenticated),<br />• Magenta – successful (Security S2 Authenticated),<br />• Red – not successful.</p> <br /> <h2>Exclusion Information</h2><p>To remove the device from the Z-Wave network:</p><p>1. Set the main controller into remove mode (see the controller’s manual).<br />2. Quickly, three times click, then press and hold one of the buttons for 12 seconds.<br />3. Release the button when the device glows green.<br />4. Quickly click the button to confirm.<br />5. LED will start blinking yellow, wait for the removing process to end.<br />6. Successful removing will be confirmed by the Z-Wave controller’s message and red LED colour</p> <br /> <h2>Wakeup Information</h2><p>You can wake up the device manually using first menu position (white):</p><p>1. Quickly, three times click, then press and hold one of the buttons.</p><p>2. After 3 seconds LED ring signals adding status:<br /></p>• Blinking green – entering the menu (added as non-secure, S0, S2 non-authenticated)<br />• Blinking magenta – entering the menu (added as Security S2 Authenticated)<br />• Blinking red – entering the menu (not added to a Z-Wave network)<p>3. The LED ring will turn off for 3 seconds, then start signalling menu positions.</p><p>4. Release the button when device signals desired position with colour:<br />• WHITE – wake up the device<br />• GREEN – start learning mode (add/remove)<br />• MAGENTA – test Z-Wave range<br />• CYAN – show battery level<br />» Green – 50-100%<br />» Yellow – 16-49%<br />» Red – 1-15%<br />• YELLOW – reset to factory default</p><p>5. Quickly click the button to confirm.<br /></p>
|
||||
]]></description>
|
||||
<category>Battery</category>
|
||||
|
||||
<!-- CHANNEL DEFINITIONS -->
|
||||
<channels>
|
||||
<channel id="sensor_temperature" typeId="sensor_temperature">
|
||||
<label>Sensor (temperature)</label>
|
||||
<properties>
|
||||
<property name="binding:*:QuantityType">COMMAND_CLASS_SENSOR_MULTILEVEL;type=TEMPERATURE</property>
|
||||
</properties>
|
||||
</channel>
|
||||
<channel id="scene_number" typeId="scene_number">
|
||||
<label>Scene Number</label>
|
||||
<properties>
|
||||
<property name="binding:*:DecimalType">COMMAND_CLASS_CENTRAL_SCENE</property>
|
||||
</properties>
|
||||
</channel>
|
||||
<channel id="battery-level" typeId="system.battery-level">
|
||||
<properties>
|
||||
<property name="binding:*:PercentType">COMMAND_CLASS_BATTERY</property>
|
||||
</properties>
|
||||
</channel>
|
||||
</channels>
|
||||
|
||||
<!-- DEVICE PROPERTY DEFINITIONS -->
|
||||
<properties>
|
||||
<property name="vendor">Fibargroup</property>
|
||||
<property name="modelId">FGWCEU-201</property>
|
||||
<property name="manufacturerId">010F</property>
|
||||
<property name="manufacturerRef">2301:1000</property>
|
||||
<property name="dbReference">1383</property>
|
||||
<property name="defaultAssociations">1</property>
|
||||
</properties>
|
||||
|
||||
<!-- CONFIGURATION DESCRIPTIONS -->
|
||||
<config-description>
|
||||
|
||||
<!-- GROUP DEFINITIONS -->
|
||||
<parameter-group name="configuration">
|
||||
<context>setup</context>
|
||||
<label>Configuration Parameters</label>
|
||||
</parameter-group>
|
||||
|
||||
<parameter-group name="association">
|
||||
<context>link</context>
|
||||
<label>Association Groups</label>
|
||||
</parameter-group>
|
||||
|
||||
<!-- PARAMETER DEFINITIONS -->
|
||||
<parameter name="config_13_1" type="integer" groupName="configuration"
|
||||
min="0" max="100">
|
||||
<label>13: LED frame – brightness</label>
|
||||
<description><![CDATA[
|
||||
This parameter allows to adjust the LED frame brightness<br /> <h1>Overview</h1><p>0 – LED disabled<br />1-100 (1-100% brightness)</p>
|
||||
]]></description>
|
||||
<default>100</default>
|
||||
<limitToOptions>false</limitToOptions>
|
||||
</parameter>
|
||||
|
||||
<parameter name="config_20_1" type="integer" groupName="configuration"
|
||||
min="0" max="6">
|
||||
<label>20: Operation mode</label>
|
||||
<description><![CDATA[
|
||||
This parameter defines operation of the device. Choose the mode depending on the type of the device you want to control remotely<br /> <h1>Overview</h1><p>0 – scene controller mode<br />1 – double button mode<br />2 – single button mode<br />3 – switch controller mode<br />4 – dimmer / roller shutter controller mode<br />5 – roller shutter controller mode (step-by-step)<br />6 – venetian blinds controller mode (step-by-step)</p>
|
||||
]]></description>
|
||||
<default>0</default>
|
||||
<limitToOptions>false</limitToOptions>
|
||||
</parameter>
|
||||
|
||||
<parameter name="config_150_2" type="integer" groupName="configuration"
|
||||
min="0" max="8">
|
||||
<label>150: LED ring – first button</label>
|
||||
<description><![CDATA[
|
||||
This parameter defines the colour of first button indicator (upper part of the LED ring) for indications using Indicator CC.<br /> <h1>Overview</h1><p>0 – LED disabled<br />1 – White<br />2 – Red<br />3 – Green<br />4 – Blue<br />5 – Yellow<br />6 – Cyan<br />7 – Magenta<br />8 – Blinking red-white-blue</p>
|
||||
]]></description>
|
||||
<default>1</default>
|
||||
<limitToOptions>false</limitToOptions>
|
||||
</parameter>
|
||||
|
||||
<parameter name="config_151_2" type="integer" groupName="configuration"
|
||||
min="0" max="8">
|
||||
<label>151: LED ring – second button</label>
|
||||
<description><![CDATA[
|
||||
This parameter defines the colour of second button indicator (lower part of the LED ring) for indications using Indicator CC.<br /> <h1>Overview</h1><p>0 – LED disabled<br />1 – White<br />2 – Red<br />3 – Green<br />4 – Blue<br />5 – Yellow<br />6 – Cyan<br />7 – Magenta<br />8 – Blinking red-white-blue</p>
|
||||
]]></description>
|
||||
<default>1</default>
|
||||
<limitToOptions>false</limitToOptions>
|
||||
</parameter>
|
||||
|
||||
<parameter name="config_152_2" type="integer" groupName="configuration"
|
||||
min="0" max="255">
|
||||
<label>152: 1st button - double click value</label>
|
||||
<description><![CDATA[
|
||||
This parameter defines value of Basic Set or Multilevel Set frame (depending on selected mode) sent to associated devices after double click. This parameter is not relevant for Scene Controller Mode<br /> <h1>Overview</h1><p>Available values 0-99 or 255</p>
|
||||
]]></description>
|
||||
<default>99</default>
|
||||
<limitToOptions>false</limitToOptions>
|
||||
</parameter>
|
||||
|
||||
<parameter name="config_153_2" type="integer" groupName="configuration"
|
||||
min="0" max="255">
|
||||
<label>153: 2nd button - double click value</label>
|
||||
<description><![CDATA[
|
||||
This parameter defines value of Basic Set or Multilevel Set frame (depending on selected mode) sent to associated devices after double click. This parameter is used only in Double Button Mode.<br /> <h1>Overview</h1><p>Available values 0-99 or 255</p>
|
||||
]]></description>
|
||||
<default>99</default>
|
||||
<limitToOptions>false</limitToOptions>
|
||||
</parameter>
|
||||
|
||||
<!-- ASSOCIATION DEFINITIONS -->
|
||||
<parameter name="group_1" type="text" groupName="association">
|
||||
<label>1: Lifeline</label>
|
||||
<description>Reports the device status and allows for assigning single device only (main controller by default)</description>
|
||||
</parameter>
|
||||
|
||||
<parameter name="group_2" type="text" groupName="association" multiple="true">
|
||||
<label>2: On/Off (1)</label>
|
||||
<description>Used to turn the associated devices on/off.</description>
|
||||
<multipleLimit>5</multipleLimit>
|
||||
</parameter>
|
||||
|
||||
<parameter name="group_3" type="text" groupName="association" multiple="true">
|
||||
<label>3: Dimmer (1)</label>
|
||||
<description>Used to change level of associated devices</description>
|
||||
<multipleLimit>5</multipleLimit>
|
||||
</parameter>
|
||||
|
||||
<parameter name="group_4" type="text" groupName="association" multiple="true">
|
||||
<label>4: On/Off (2)</label>
|
||||
<description>Used to turn the associated devices on/off</description>
|
||||
<multipleLimit>5</multipleLimit>
|
||||
</parameter>
|
||||
|
||||
<parameter name="group_5" type="text" groupName="association" multiple="true">
|
||||
<label>5: Dimmer (2)</label>
|
||||
<description>Used to change level of associated devices</description>
|
||||
<multipleLimit>5</multipleLimit>
|
||||
</parameter>
|
||||
|
||||
<parameter name="group_6" type="text" groupName="association" multiple="true">
|
||||
<label>6: Multidevice</label>
|
||||
<description>Used to control different type of devices</description>
|
||||
<multipleLimit>5</multipleLimit>
|
||||
</parameter>
|
||||
|
||||
<parameter name="group_7" type="text" groupName="association" multiple="true">
|
||||
<label>7: Slats</label>
|
||||
<description>Used to move slats of Venetian blinds.</description>
|
||||
<multipleLimit>5</multipleLimit>
|
||||
</parameter>
|
||||
|
||||
<!-- STATIC DEFINITIONS -->
|
||||
<parameter name="node_id" type="integer" min="1" max="232" readOnly="true" required="true">
|
||||
<label>Node ID</label>
|
||||
<advanced>true</advanced>
|
||||
</parameter>
|
||||
|
||||
</config-description>
|
||||
|
||||
</thing-type>
|
||||
|
||||
</thing:thing-descriptions>
|
|
@ -1,119 +0,0 @@
|
|||
<?xml version="1.0" encoding="UTF-8"?>
|
||||
<thing:thing-descriptions bindingId="zwave"
|
||||
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
|
||||
xmlns:thing="https://openhab.org/schemas/thing-description/v1.0.0"
|
||||
xsi:schemaLocation="https://openhab.org/schemas/thing-description/v1.0.0
|
||||
https://openhab.org/schemas/thing-description/v1.0.0">
|
||||
|
||||
<thing-type id="mcohome_c221_00_000" listed="false">
|
||||
<label>MH-C221 MCO HOME Micro Shutter</label>
|
||||
<description><![CDATA[
|
||||
MCO HOME Micro Shutter<br /> <h1>Overview</h1><p>MCO HOME Micro Shutter for windows roller shutter</p> <p>The device allows for controlling motors of roller blinds, awnings, venetian blinds, gates and others, which are single phase AC powered. This Roller Shutter allows for precise positioning of a roller blind or venetian blind lamellas. Precise positioning is available for the motors equipped with mechanic and electronic end switches.</p> <p>The module may be controlled wirelessly, through the Z-Wave network main controller, or through the switch keys connected to it. It’s also possible to combine few devices into groups of devices, which then can be controlled simultaneously. In addition, this Roller Shutter is equipped with Power Metering.</p> <br /> <h2>Inclusion Information</h2><ol><li>Connect the power supply.</li> <li>Set the main controller into the learn mode (see main controllers operating manual).</li> <li>Triple click the B-button or a push button connected to the S1 terminal.</li> <li>MCO Home Roller Shutter will be detected and included into the Z-Wave network</li> </ol> <br /> <h2>Exclusion Information</h2><ol><li>Make sure the module is connected to the power supply.</li> <li>Set the main controller into the learn mode (see main controllers operating manual).</li> <li>Triple click the B-button or a push button connected to the S1 terminal.</li> </ol> <br /> <h2>Wakeup Information</h2><p>Press B button</p>
|
||||
]]></description>
|
||||
<category>Blinds</category>
|
||||
|
||||
<!-- CHANNEL DEFINITIONS -->
|
||||
<channels>
|
||||
<channel id="switch_binary" typeId="switch_binary">
|
||||
<label>switch_binary</label>
|
||||
<properties>
|
||||
<property name="binding:*:OnOffType">COMMAND_CLASS_SWITCH_BINARY</property>
|
||||
</properties>
|
||||
</channel>
|
||||
<channel id="blinds_control" typeId="blinds_control">
|
||||
<label>blinds control</label>
|
||||
<properties>
|
||||
<property name="binding:*:PercentType">COMMAND_CLASS_SWITCH_MULTILEVEL</property>
|
||||
<property name="binding:Command:StopMoveType">COMMAND_CLASS_SWITCH_MULTILEVEL</property>
|
||||
<property name="binding:Command:UpDownType">COMMAND_CLASS_SWITCH_MULTILEVEL</property>
|
||||
</properties>
|
||||
</channel>
|
||||
<channel id="meter_watts" typeId="meter_watts">
|
||||
<label>Electric Meter (Watts) 1</label>
|
||||
<properties>
|
||||
<property name="binding:*:DecimalType">COMMAND_CLASS_METER;meterScale=E_W</property>
|
||||
</properties>
|
||||
</channel>
|
||||
<channel id="meter_voltage" typeId="meter_voltage">
|
||||
<label>Electric Meter (Volts)</label>
|
||||
<properties>
|
||||
<property name="binding:*:DecimalType">COMMAND_CLASS_METER</property>
|
||||
</properties>
|
||||
</channel>
|
||||
<channel id="meter_current" typeId="meter_current">
|
||||
<label>Electric Meter (amp)</label>
|
||||
<properties>
|
||||
<property name="binding:*:DecimalType">COMMAND_CLASS_METER</property>
|
||||
</properties>
|
||||
</channel>
|
||||
<channel id="meter_kwh" typeId="meter_kwh">
|
||||
<label>Electric Meter (Kwh)</label>
|
||||
<properties>
|
||||
<property name="binding:*:DecimalType">COMMAND_CLASS_METER</property>
|
||||
</properties>
|
||||
</channel>
|
||||
<channel id="alarm_general" typeId="alarm_general">
|
||||
<label>Alarm</label>
|
||||
<properties>
|
||||
<property name="binding:*:OnOffType">COMMAND_CLASS_ALARM</property>
|
||||
</properties>
|
||||
</channel>
|
||||
</channels>
|
||||
|
||||
<!-- DEVICE PROPERTY DEFINITIONS -->
|
||||
<properties>
|
||||
<property name="vendor">McoHome Technology Co., Ltd</property>
|
||||
<property name="modelId">MH-C221</property>
|
||||
<property name="manufacturerId">015F</property>
|
||||
<property name="manufacturerRef">0301:1001,0301:3001,0302:1000,C221:5102</property>
|
||||
<property name="dbReference">1038</property>
|
||||
<property name="defaultAssociations">1</property>
|
||||
</properties>
|
||||
|
||||
<!-- CONFIGURATION DESCRIPTIONS -->
|
||||
<config-description>
|
||||
|
||||
<!-- GROUP DEFINITIONS -->
|
||||
<parameter-group name="configuration">
|
||||
<context>setup</context>
|
||||
<label>Configuration Parameters</label>
|
||||
</parameter-group>
|
||||
|
||||
<parameter-group name="association">
|
||||
<context>link</context>
|
||||
<label>Association Groups</label>
|
||||
</parameter-group>
|
||||
|
||||
<!-- PARAMETER DEFINITIONS -->
|
||||
<parameter name="config_1_2" type="integer" groupName="configuration"
|
||||
min="1" max="32767">
|
||||
<label>1: Watt meter report period</label>
|
||||
<description>Watt meter report period</description>
|
||||
<default>720</default>
|
||||
<limitToOptions>false</limitToOptions>
|
||||
</parameter>
|
||||
|
||||
<!-- ASSOCIATION DEFINITIONS -->
|
||||
<parameter name="group_1" type="text" groupName="association">
|
||||
<label>1: Group 1</label>
|
||||
</parameter>
|
||||
|
||||
<parameter name="group_2" type="text" groupName="association">
|
||||
<label>2: Group 2</label>
|
||||
</parameter>
|
||||
|
||||
<parameter name="group_3" type="text" groupName="association">
|
||||
<label>3: Group 3</label>
|
||||
</parameter>
|
||||
|
||||
<!-- STATIC DEFINITIONS -->
|
||||
<parameter name="node_id" type="integer" min="1" max="232" readOnly="true" required="true">
|
||||
<label>Node ID</label>
|
||||
<advanced>true</advanced>
|
||||
</parameter>
|
||||
|
||||
</config-description>
|
||||
|
||||
</thing-type>
|
||||
|
||||
</thing:thing-descriptions>
|
|
@ -0,0 +1,148 @@
|
|||
<?xml version="1.0" encoding="UTF-8"?>
|
||||
<thing:thing-descriptions bindingId="zwave"
|
||||
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
|
||||
xmlns:thing="https://openhab.org/schemas/thing-description/v1.0.0"
|
||||
xsi:schemaLocation="https://openhab.org/schemas/thing-description/v1.0.0
|
||||
https://openhab.org/schemas/thing-description/v1.0.0">
|
||||
|
||||
<thing-type id="polycontrol_btze_00_000" listed="false">
|
||||
<label>Danalock V3-BTZE Z-Wave controlled door lock with Bluetooth Smart</label>
|
||||
<description><![CDATA[
|
||||
Z-Wave controlled door lock with Bluetooth Smart<br /> <h1>Overview</h1><p>Danalock supports:</p> <ul><li>S0/S2 Z-Wave Security</li> <li>Bluetooth Smart</li> <li>Twist Assist</li> <li>Auto Lock</li> <li>Hold And Release</li> <li>Back To Back </li> </ul> <br /> <h2>Inclusion Information</h2><p>To add or include the Danalock into a Z-Wave network</p> <ol><li>Pair the lock with bluetooth first</li> <li> <p>Set the controller in inclusion mode</p> </li> <li> <p>Push the switch once.</p> </li> <li> <p>Wait 5 seconds. </p> </li> </ol> <br /> <h2>Exclusion Information</h2><p>To remove or exclude the Danalock into a Z-Wave network</p> <ol><li>Delete the lock within the app (bluetooth)</li> <li> <p>Set the controller in exclusion mode</p> </li> <li> <p>Push the switch once.</p> </li> <li> <p>Wait 5 seconds. </p> </li> </ol>
|
||||
]]></description>
|
||||
<category>Lock</category>
|
||||
|
||||
<!-- CHANNEL DEFINITIONS -->
|
||||
<channels>
|
||||
<channel id="lock_door" typeId="lock_door">
|
||||
<label>Door Lock</label>
|
||||
<properties>
|
||||
<property name="binding:*:OnOffType">COMMAND_CLASS_DOOR_LOCK</property>
|
||||
</properties>
|
||||
</channel>
|
||||
<channel id="alarm_entry" typeId="alarm_entry">
|
||||
<label>Entry Alarm</label>
|
||||
<properties>
|
||||
<property name="binding:*:OnOffType">COMMAND_CLASS_ALARM</property>
|
||||
</properties>
|
||||
</channel>
|
||||
<channel id="battery-level" typeId="system.battery-level">
|
||||
<properties>
|
||||
<property name="binding:*:PercentType">COMMAND_CLASS_BATTERY</property>
|
||||
</properties>
|
||||
</channel>
|
||||
</channels>
|
||||
|
||||
<!-- DEVICE PROPERTY DEFINITIONS -->
|
||||
<properties>
|
||||
<property name="vendor">Poly-control</property>
|
||||
<property name="modelId">Danalock V3-BTZE</property>
|
||||
<property name="manufacturerId">010E</property>
|
||||
<property name="manufacturerRef">0009:0001</property>
|
||||
<property name="dbReference">708</property>
|
||||
<property name="defaultAssociations">1</property>
|
||||
</properties>
|
||||
|
||||
<!-- CONFIGURATION DESCRIPTIONS -->
|
||||
<config-description>
|
||||
|
||||
<!-- GROUP DEFINITIONS -->
|
||||
<parameter-group name="configuration">
|
||||
<context>setup</context>
|
||||
<label>Configuration Parameters</label>
|
||||
</parameter-group>
|
||||
|
||||
<parameter-group name="association">
|
||||
<context>link</context>
|
||||
<label>Association Groups</label>
|
||||
</parameter-group>
|
||||
|
||||
<!-- PARAMETER DEFINITIONS -->
|
||||
<parameter name="config_1_1" type="integer" groupName="configuration">
|
||||
<label>1: Twist Assist</label>
|
||||
<description><![CDATA[
|
||||
Helps you to lock and unlock your door lock.<br /> <h1>Overview</h1><p>0 -> disabled</p> <p>1 -> enabled</p>
|
||||
]]></description>
|
||||
<default>0</default>
|
||||
<options>
|
||||
<option value="0">Disabled</option>
|
||||
<option value="1">Enabled</option>
|
||||
</options>
|
||||
<limitToOptions>false</limitToOptions>
|
||||
</parameter>
|
||||
|
||||
<parameter name="config_2_4" type="integer" groupName="configuration">
|
||||
<label>2: Hold and Release</label>
|
||||
<description><![CDATA[
|
||||
After a unlock operation the lock holds the lock, so the door can be opened<br /> <h1>Overview</h1><p>0 -> disabled</p> <p>1 to 2147483647 -> enabled no. of seconds</p>
|
||||
]]></description>
|
||||
<default>0</default>
|
||||
<options>
|
||||
<option value="0">Disabled</option>
|
||||
</options>
|
||||
<limitToOptions>false</limitToOptions>
|
||||
</parameter>
|
||||
|
||||
<parameter name="config_3_1" type="integer" groupName="configuration">
|
||||
<label>3: Block to block</label>
|
||||
<description>The lock will run the motor until it hits resistance.</description>
|
||||
<default>0</default>
|
||||
<options>
|
||||
<option value="0">Disabled</option>
|
||||
<option value="1">Enabled</option>
|
||||
</options>
|
||||
<limitToOptions>false</limitToOptions>
|
||||
</parameter>
|
||||
|
||||
<parameter name="config_4_4" type="integer" groupName="configuration"
|
||||
min="0" max="2147483647">
|
||||
<label>4: BLE Temporary Allowed</label>
|
||||
<description><![CDATA[
|
||||
The BLE is enabled for a number of seconds.<br /> <h1>Overview</h1><p>0 -> Disabled</p> <p>1 to 2147483647 -> enabled no. of seconds</p>
|
||||
]]></description>
|
||||
<default>0</default>
|
||||
<limitToOptions>false</limitToOptions>
|
||||
</parameter>
|
||||
|
||||
<parameter name="config_5_1" type="integer" groupName="configuration">
|
||||
<label>5: BLE Always Allowed</label>
|
||||
<description><![CDATA[
|
||||
BLE Always Allowed<br /> <h1>Overview</h1><p>0 -> BLE Always disabled</p> <p>1 -> BLE Always enabled</p>
|
||||
]]></description>
|
||||
<default>0</default>
|
||||
<options>
|
||||
<option value="0">BLE Always disabled</option>
|
||||
<option value="1">BLE Always enabled</option>
|
||||
</options>
|
||||
<limitToOptions>false</limitToOptions>
|
||||
</parameter>
|
||||
|
||||
<parameter name="config_6_4" type="integer" groupName="configuration"
|
||||
min="0" max="2147483647">
|
||||
<label>6: Autolock</label>
|
||||
<description><![CDATA[
|
||||
Lock after an unlock.Value is delay in sec before lock is locked again.<br /> <h1>Overview</h1><p>0 -> disabled</p> <p>1 to 2147483647 -> enabled no. of seconds</p>
|
||||
]]></description>
|
||||
<default>0</default>
|
||||
<limitToOptions>false</limitToOptions>
|
||||
</parameter>
|
||||
|
||||
<!-- ASSOCIATION DEFINITIONS -->
|
||||
<parameter name="group_1" type="text" groupName="association">
|
||||
<label>1: Lifeline</label>
|
||||
<description><![CDATA[
|
||||
Z-Wave Plus Lifeline<br /> <h1>Overview</h1><p>Z-Wave Plus Lifeline</p> <ul> <li>Device Reset Locally: triggered upon reset.</li> <li>Battery/notification: triggered upon low battery.</li> <li>Door Lock operation report: triggered upon a change in door lock</li> <li>Notification: triggered upon a change in door lock</li> </ul>
|
||||
]]></description>
|
||||
</parameter>
|
||||
|
||||
<!-- STATIC DEFINITIONS -->
|
||||
<parameter name="node_id" type="integer" min="1" max="232" readOnly="true" required="true">
|
||||
<label>Node ID</label>
|
||||
<advanced>true</advanced>
|
||||
</parameter>
|
||||
|
||||
</config-description>
|
||||
|
||||
</thing-type>
|
||||
|
||||
</thing:thing-descriptions>
|
|
@ -32,7 +32,7 @@ Remote switch<br /> <h1>Overview</h1><p>* Control other Z-Wave devices and enabl
|
|||
<property name="vendor">Zooz</property>
|
||||
<property name="modelId">ZEN34</property>
|
||||
<property name="manufacturerId">027A</property>
|
||||
<property name="manufacturerRef">0004:F001,7000:F001</property>
|
||||
<property name="manufacturerRef">0004:F001</property>
|
||||
<property name="dbReference">1310</property>
|
||||
<property name="defaultAssociations">1</property>
|
||||
</properties>
|
||||
|
|
|
@ -0,0 +1,124 @@
|
|||
<?xml version="1.0" encoding="UTF-8"?>
|
||||
<thing:thing-descriptions bindingId="zwave"
|
||||
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
|
||||
xmlns:thing="https://openhab.org/schemas/thing-description/v1.0.0"
|
||||
xsi:schemaLocation="https://openhab.org/schemas/thing-description/v1.0.0
|
||||
https://openhab.org/schemas/thing-description/v1.0.0">
|
||||
|
||||
<thing-type id="zooz_zen34v101_00_000" listed="false">
|
||||
<label>zen34v1.01 Remote Switch</label>
|
||||
<description><![CDATA[
|
||||
Remote Switch<br /> <h1>Overview</h1><p>* Control other Z-Wave devices and enable scenes with a click of a button</p><p>* Classic paddle switch look and size + magnetic base</p><p>* Install in your electrical switch box or mount anywhere on a wall with just adhesive tape</p><p>* The latest 700 chip with S2 and SmartStart support</p><p>* Powered by batteries, no wires needed</p> <br /> <h2>Inclusion Information</h2><p>Put the openHAB Z-Wave binding into inclusion mode and click the upper paddle 6 times quickly. The LED indicator will blink blue during the process and light up green once added successfully. It will light up red if failed.<br /></p> <br /> <h2>Exclusion Information</h2><p>Put the openHAB Z-Wave binding into exclusion mode and click the lower paddle 6 times quickly.<br /></p> <br /> <h2>Wakeup Information</h2><p><br /></p>
|
||||
]]></description>
|
||||
<category>RemoteControl</category>
|
||||
|
||||
<!-- CHANNEL DEFINITIONS -->
|
||||
<channels>
|
||||
<channel id="scene_number" typeId="scene_number">
|
||||
<label>Scene Number</label>
|
||||
<properties>
|
||||
<property name="binding:*:DecimalType">COMMAND_CLASS_CENTRAL_SCENE</property>
|
||||
</properties>
|
||||
</channel>
|
||||
<channel id="battery-level" typeId="system.battery-level">
|
||||
<properties>
|
||||
<property name="binding:*:PercentType">COMMAND_CLASS_BATTERY</property>
|
||||
</properties>
|
||||
</channel>
|
||||
</channels>
|
||||
|
||||
<!-- DEVICE PROPERTY DEFINITIONS -->
|
||||
<properties>
|
||||
<property name="vendor">Zooz</property>
|
||||
<property name="modelId">zen34v1.01</property>
|
||||
<property name="manufacturerId">027A</property>
|
||||
<property name="manufacturerRef">7000:F001</property>
|
||||
<property name="dbReference">1382</property>
|
||||
<property name="defaultAssociations">1</property>
|
||||
</properties>
|
||||
|
||||
<!-- CONFIGURATION DESCRIPTIONS -->
|
||||
<config-description>
|
||||
|
||||
<!-- GROUP DEFINITIONS -->
|
||||
<parameter-group name="configuration">
|
||||
<context>setup</context>
|
||||
<label>Configuration Parameters</label>
|
||||
</parameter-group>
|
||||
|
||||
<parameter-group name="association">
|
||||
<context>link</context>
|
||||
<label>Association Groups</label>
|
||||
</parameter-group>
|
||||
|
||||
<!-- PARAMETER DEFINITIONS -->
|
||||
<parameter name="config_1_1" type="integer" groupName="configuration"
|
||||
min="0" max="2">
|
||||
<label>1: LED indication mode</label>
|
||||
<default>1</default>
|
||||
<options>
|
||||
<option value="0">LED always OFF</option>
|
||||
<option value="1">LED ON when button pressed</option>
|
||||
<option value="2">LED always ON (color from #2)</option>
|
||||
<option value="3">LED always ON (color from #3)</option>
|
||||
</options>
|
||||
</parameter>
|
||||
|
||||
<parameter name="config_2_1" type="integer" groupName="configuration"
|
||||
min="0" max="6">
|
||||
<label>2: Upper paddle indicator color</label>
|
||||
<default>1</default>
|
||||
<options>
|
||||
<option value="0">White</option>
|
||||
<option value="1">Blue</option>
|
||||
<option value="2">Green</option>
|
||||
<option value="3">Red</option>
|
||||
<option value="4">Magenta</option>
|
||||
<option value="5">Yellow</option>
|
||||
<option value="6">Cyan</option>
|
||||
</options>
|
||||
</parameter>
|
||||
|
||||
<parameter name="config_3_1" type="integer" groupName="configuration"
|
||||
min="0" max="6">
|
||||
<label>3: Lower paddle indicator color</label>
|
||||
<default>1</default>
|
||||
<options>
|
||||
<option value="0">White</option>
|
||||
<option value="1">Blue</option>
|
||||
<option value="2">Green</option>
|
||||
<option value="3">Red</option>
|
||||
<option value="4">Magenta</option>
|
||||
<option value="5">Yellow</option>
|
||||
<option value="6">Cyan</option>
|
||||
</options>
|
||||
</parameter>
|
||||
|
||||
<!-- ASSOCIATION DEFINITIONS -->
|
||||
<parameter name="group_1" type="text" groupName="association">
|
||||
<label>1: Lifeline</label>
|
||||
</parameter>
|
||||
|
||||
<parameter name="group_2" type="text" groupName="association" multiple="true">
|
||||
<label>2: On/Off</label>
|
||||
<description>1-tap for on.off control</description>
|
||||
<multipleLimit>5</multipleLimit>
|
||||
</parameter>
|
||||
|
||||
<parameter name="group_3" type="text" groupName="association" multiple="true">
|
||||
<label>3: dimming</label>
|
||||
<description>Paddle held/released for dimming</description>
|
||||
<multipleLimit>5</multipleLimit>
|
||||
</parameter>
|
||||
|
||||
<!-- STATIC DEFINITIONS -->
|
||||
<parameter name="node_id" type="integer" min="1" max="232" readOnly="true" required="true">
|
||||
<label>Node ID</label>
|
||||
<advanced>true</advanced>
|
||||
</parameter>
|
||||
|
||||
</config-description>
|
||||
|
||||
</thing-type>
|
||||
|
||||
</thing:thing-descriptions>
|
|
@ -0,0 +1,151 @@
|
|||
## Bintray shutdown ## May 3, 2021
|
||||
Bintray, the hosting service formerly used for the openHAB stable distribution,
|
||||
has shutdown their service effective May 1st, 2021. As a result any APT
|
||||
repositories using the Bintray service need to be replaced. For openHAB, we have
|
||||
moved to using Artifactory as our hosting service, `openhabian-config` will ask
|
||||
you on startup about automatically replacing the openHAB stable repository for
|
||||
you. Check `/etc/apt/sources.list.d/*` afterwards for any other APT repositories
|
||||
using Bintray as they will not be automatically replaced.
|
||||
|
||||
## Future of master branch ## January 20, 2021
|
||||
We will no longer make regular updates to the master branch as we migrate away
|
||||
from supporting openHAB 2. As such in the coming months we will make bug fixes
|
||||
directly to the `stable` branch for openHA 2. With that said, please migrate off
|
||||
of the `master` branch as it will be deleted soon. You can change branches at
|
||||
any time using menu option 01.
|
||||
|
||||
|
||||
## openHAB 3 released ## December 21, 2020
|
||||
In the darkest of times (midwinter for most of us), openHAB 3 was released.
|
||||
See [documentation](openhabian.md#on-openhab-2-and-3) and
|
||||
[www.openhab.org](https://www.openhab.org) for details.
|
||||
|
||||
Merry Christmas and a healthy New Year!
|
||||
|
||||
|
||||
## WiFi hotspot ## November 14, 2020
|
||||
Whenever your system has a WiFi interface that fails to initialize on
|
||||
installation or startup, openHABian will now launch a
|
||||
[WiFi hotspot](openhabian.md#WiFi-Hotspot) you can use to bootstrap WiFi i.e. to
|
||||
connect your system to an existing WiFi network.
|
||||
|
||||
|
||||
## openHAB 3 readiness ## October 28, 2020
|
||||
openHABian now provides menu options 4X to upgrade your system to openHAB3 and
|
||||
to downgrade back to current openHAB 2 See [documentation](openhabian.md) for
|
||||
details. Please be aware that openHAB3 as well as openHABian are not thoroughly
|
||||
tested so be prepared to meet bugs and problems in the migration process as
|
||||
well. Don't migrate your production system unless you're fully aware of the
|
||||
consequences.
|
||||
|
||||
|
||||
## Tailscale VPN network ## October 6, 2020
|
||||
Tailscale is a management toolset to establish a WireGuard based VPN between
|
||||
multiple systems if you want to connect to openHAB(ian) instances outside your
|
||||
LAN over Internet. It'll take care to detect and open ports when you and your
|
||||
peers are located behind firewalls. Don't worry, for private use it's free of
|
||||
charge.
|
||||
|
||||
|
||||
## Offline installation ## October 1, 2020
|
||||
We now allow for deploying openHABian to destination networks without Internet
|
||||
connectivity. While the optional components still require access to download,
|
||||
the openHABian core is fully contained in the download image and can be
|
||||
installed and run without Internet. This will also provide a failsafe
|
||||
installation when any of the online sources for the tools we need to download is
|
||||
unavailable for whatever reason.
|
||||
|
||||
|
||||
## Auto-backup ## August 29, 2020
|
||||
openHABian can automatically take daily syncs of your internal SD card to
|
||||
another card in an external port. This allows for fast swapping of cards to
|
||||
reduce impact of a failed SD card. The remaining space on the external device
|
||||
can also be used to setup openHABian's Amanda backup system.
|
||||
|
||||
|
||||
## Wireguard VPN ## July 4, 2020
|
||||
Wireguard can be deployed to enable for VPN access to your openHABian box when
|
||||
it's located in some remote location.
|
||||
You need to install the Wireguard client from <http://www.wireguard.com/install>
|
||||
to your local PC or mobile device that you want to use for access.
|
||||
Copy the configuration file `/etc/wireguard/wg0-client.conf` from this box or
|
||||
transmit QR code to load the tunnel.
|
||||
Any feedback is highly appreciated on the forum.
|
||||
|
||||
|
||||
### New Java providers now out of beta
|
||||
Java 11 has been proven to work with openHAB 2.5.
|
||||
|
||||
|
||||
## End support for PINE A64(+) and older Linux distributions ## June 17, 2020
|
||||
`openhabian-config` will now issue a warning if you start on unsupported
|
||||
hardware or OS releases. See [README](../README.md) for supported HW and OS.
|
||||
|
||||
In short, PINE A64 is no longer supported and OS releases other than the current
|
||||
`stable` and the previous one are deprecated. Running on any of those may still
|
||||
work or not.
|
||||
|
||||
The current and previous Debian / Raspberry Pi OS (previously called Raspbian)
|
||||
releases are 10 ("buster") and 9 ("stretch"). The most current Ubuntu LTS
|
||||
releases are 20.04 ("focal") and 18.04 ("bionic").
|
||||
|
||||
|
||||
## New parameters in `openhabian.conf` ## June 10, 2020
|
||||
See `/etc/openhabian.conf` for a number of new parameters such as the useful
|
||||
`debugmode`, a fake hardware mode, the option to disable ipv6 and the ability to
|
||||
update from a custom repository other than the `master` and `stable` branches.
|
||||
|
||||
In case you are not aware, there is a Debug Guide in the `docs/` directory.
|
||||
|
||||
|
||||
### New Java options
|
||||
Preparing for openHAB 3, new options for the JDK that runs openHAB are now
|
||||
available:
|
||||
|
||||
- Java Zulu 8 32-Bit OpenJDK (default on ARM based platforms)
|
||||
- Java Zulu 8 64-Bit OpenJDK (default on x86 based platforms)
|
||||
- Java Zulu 11 32-Bit OpenJDK
|
||||
- Java Zulu 11 64-Bit OpenJDK
|
||||
- AdoptOpenJDK 11 OpenJDK (potential replacement for Zulu)
|
||||
|
||||
openHAB 3 will be Java 11 only. 2.5.X is supposed to work on both, Java 8 and
|
||||
Java 11. Running the current openHAB 2.X on Java 11 however has not been tested
|
||||
on a wide scale. Please be aware that there is a small number of known issues in
|
||||
this: v1 bindings may or may not work.
|
||||
|
||||
Please participate in beta testing to help create a smooth transition user
|
||||
experience for all of us.
|
||||
|
||||
See [announcement thread](https://community.openhab.org/t/Java-testdrive/99827)
|
||||
on the community forum.
|
||||
|
||||
|
||||
## Stable branch ## May 31, 2020
|
||||
Introducing a new versioning scheme to openHABian. Please welcome the `stable`
|
||||
branch.
|
||||
|
||||
Similar to openHAB where there's releases and snapshots, you will from now on be
|
||||
using the stable branch. It's the equivalent of an openHAB release. We will keep
|
||||
providing new changes to the master branch first as soon as we make them
|
||||
available, just like we have been doing in the past. If you want to keep living
|
||||
on the edge, want to make use of new features fast or volunteer to help a little
|
||||
in advancing openHABian, you can choose to switch back to the master branch.
|
||||
Anybody else will benefit from less frequent but well better tested updates to
|
||||
happen to the stable branch in batches, whenever the poor daring people to use
|
||||
`master` have reported their trust in these changes to work flawlessly.
|
||||
|
||||
You can switch branches at any time using the menu option 01.
|
||||
|
||||
|
||||
### Zram enabled by default
|
||||
Swap, logs and persistence files are now put into zram per default.
|
||||
See [zram status thread](https://community.openhab.org/t/zram-status/80996) for
|
||||
more information.
|
||||
|
||||
|
||||
### Supported hardware and Operating Systems
|
||||
openHABian now fully supports all Raspberry Pi SBCs with our fast-start image.
|
||||
As an add-on package, it is supposed to run on all Debian based OSs.
|
||||
|
||||
Check the [README](../README.md) to see what "supported" actually means and what
|
||||
you can do if you want to run on other HW or OS.
|
|
@ -195,7 +195,7 @@ apt-get install git
|
|||
# download, link and create config file
|
||||
git clone -b openHAB3 https://github.com/openhab/openhabian.git /opt/openhabian
|
||||
ln -s /opt/openhabian/openhabian-setup.sh /usr/local/bin/openhabian-config
|
||||
cp /opt/openhabian/openhabian.conf.dist /etc/openhabian.conf
|
||||
cp /opt/openhabian/build-image/openhabian.conf /etc/openhabian.conf
|
||||
```
|
||||
|
||||
Edit `/etc/openhabian.conf` to match your needs, then finally use
|
||||
|
|
Loading…
Reference in New Issue